How to Use the tryCatch Function for Condition Handling in R
- Understanding the tryCatch Function
- Basic Usage of tryCatch
- Handling Warnings with tryCatch
- Using finally in tryCatch
- Conclusion
- FAQ
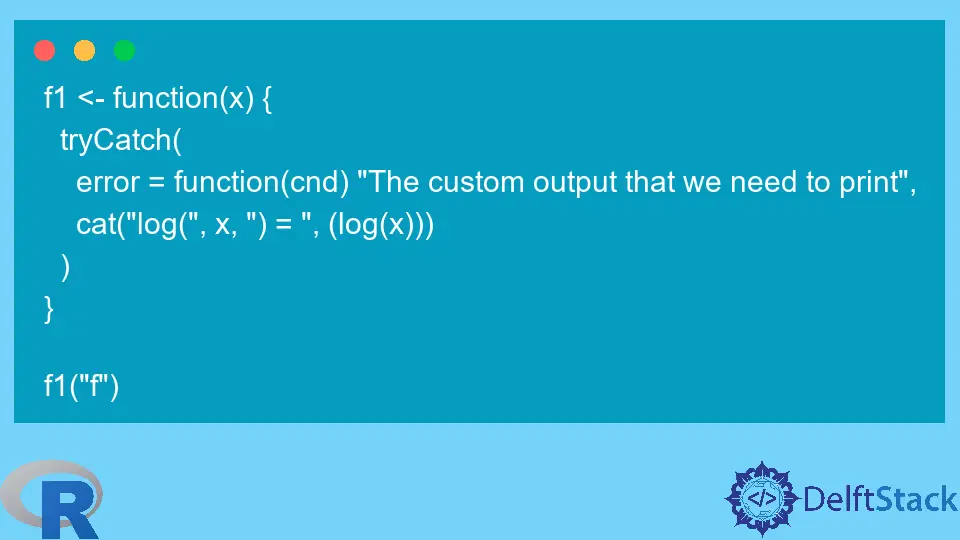
In the world of programming, errors are inevitable. Whether you’re working on a complex data analysis project or a simple script, handling errors gracefully can make a significant difference in your workflow. In R, the tryCatch
function is a powerful tool for managing conditions and errors. It allows you to execute code while anticipating potential issues, enabling you to respond appropriately without crashing your entire program.
This article will introduce you to the tryCatch
function, illustrating how to use it effectively for condition handling in R. By the end, you’ll have a solid understanding of how to implement this function in your own projects, ensuring smoother and more robust code execution.
Understanding the tryCatch Function
The tryCatch
function in R is designed to handle errors, warnings, and messages. It allows you to specify what should happen when an error occurs, enabling you to recover from it without terminating your program. The basic syntax of the tryCatch
function is as follows:
tryCatch({
# Code that may produce an error
}, error = function(e) {
# Code to handle the error
}, warning = function(w) {
# Code to handle the warning
}, finally = {
# Code to execute regardless of the outcome
})
In this structure, the first block contains the code that may generate an error. The subsequent blocks specify how to handle errors and warnings, while the finally
block will always execute, regardless of the outcome. This flexibility makes tryCatch
an essential feature for robust R programming.
Basic Usage of tryCatch
Let’s dive into a practical example of using the tryCatch
function. Suppose you have a function that attempts to divide two numbers. If the denominator is zero, it will produce an error. Here is how you can handle that using tryCatch
.
safeDivide <- function(x, y) {
result <- tryCatch({
x / y
}, error = function(e) {
paste("Error:", e$message)
})
return(result)
}
output1 <- safeDivide(10, 2)
output2 <- safeDivide(10, 0)
output1
output2
Output:
5
Error: division by zero
In this example, the safeDivide
function attempts to divide x
by y
. If y
is zero, the error handling function captures the error and returns a friendly message instead of stopping the execution. This way, you can continue processing other parts of your program without interruption.
Handling Warnings with tryCatch
In addition to handling errors, tryCatch
can also manage warnings. Let’s consider an example where we attempt to calculate the logarithm of a number. If the number is negative, R will issue a warning. We can handle this warning using tryCatch
.
logSafe <- function(x) {
result <- tryCatch({
log(x)
}, warning = function(w) {
paste("Warning:", w$message)
})
return(result)
}
output3 <- logSafe(10)
output4 <- logSafe(-10)
output3
output4
Output:
2.302585
Warning: NaNs produced
In this case, the logSafe
function computes the logarithm of x
. If x
is negative, the warning handling function captures the warning message, allowing you to handle it gracefully. This approach is beneficial when you want to log warnings or take corrective actions without halting the program.
Using finally in tryCatch
The finally
clause in tryCatch
is particularly useful for executing code that must run regardless of whether an error or warning occurred. For instance, you may want to close a connection to a database or write a log entry. Here’s how you can implement it.
fileOperation <- function(filename) {
result <- tryCatch({
con <- file(filename, "r")
data <- readLines(con)
close(con)
data
}, error = function(e) {
paste("Error:", e$message)
}, finally = {
cat("Finished attempting to read the file.\n")
})
return(result)
}
output5 <- fileOperation("data.txt")
output5
Output:
Finished attempting to read the file.
Error: cannot open the connection
In this example, we attempt to read a file. The finally
block ensures that a message is printed indicating that the file operation attempt has completed, regardless of whether it succeeded or failed. This is crucial for resource management and ensuring that your program behaves predictably.
Conclusion
The tryCatch
function is an invaluable tool for condition handling in R. By allowing you to manage errors and warnings gracefully, it enhances the robustness of your code and improves user experience. Whether you’re performing simple calculations or working with complex data operations, the ability to anticipate and respond to issues can save you time and frustration. Implementing tryCatch
in your R programming arsenal will undoubtedly lead to cleaner, more efficient code.
FAQ
- What is the purpose of the tryCatch function in R?
ThetryCatch
function is used for handling errors and warnings in R, allowing you to manage conditions gracefully without terminating your program.
-
Can tryCatch handle multiple types of conditions?
Yes,tryCatch
can handle errors, warnings, and messages, enabling you to specify how to respond to each condition. -
Is it necessary to use the finally clause in tryCatch?
No, thefinally
clause is optional. It is used for executing code that should run regardless of the outcome of the try or catch blocks. -
How can I log errors using tryCatch?
You can log errors within the error handling function by writing them to a file or printing them to the console. -
Can tryCatch be used in combination with other R functions?
Absolutely!tryCatch
can be used with any R function where you anticipate potential errors or warnings.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook