How to Export Data Frame to CSV in R
-
Use
write.csv
From Base R to Export Data Frame to CSV in R -
Use
fwrite
Fromdata.table
Package to Export Data Frame to CSV in R -
Use
write_csv
FromReader
Package to Export Data Frame to CSV in R
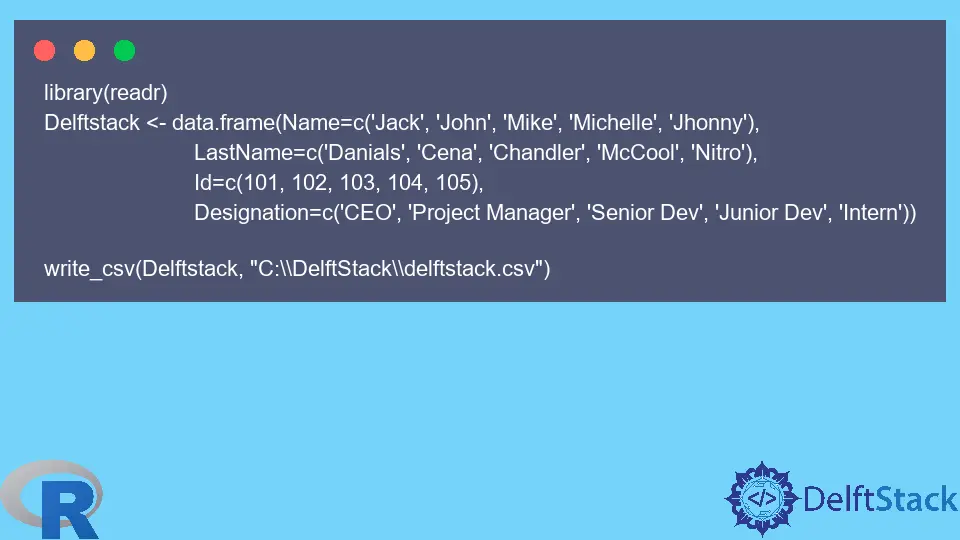
There are multiple ways to export a data frame to CSV in R. This tutorial demonstrates how to export a data frame to CSV in R.
Use write.csv
From Base R to Export Data Frame to CSV in R
The base R has the function write.csv()
, which writes a data frame into a CSV file. It takes three parameters: data frame, file path and name, and row.names
.
The row.names
can be false
if you don’t want to export the row names in the CSV file. See example:
Delftstack <- data.frame(Name=c('Jack', 'John', 'Mike', 'Michelle', 'Jhonny'),
LastName=c('Danials', 'Cena', 'Chandler', 'McCool', 'Nitro'),
Id=c(101, 102, 103, 104, 105),
Designation=c('CEO', 'Project Manager', 'Senior Dev', 'Junior Dev', 'Intern'))
write.csv(Delftstack, "C:\\Users\\Sheeraz\\OneDrive\\Documents\\data.csv", row.names=TRUE)
The code above will write the given data frame to CSV. See the output:
Use fwrite
From data.table
Package to Export Data Frame to CSV in R
fwrite
is a function from the data.table
package, which can write data frames to CSV files and take two parameters: the data frame and the file path and name. See example:
library(data.table)
Delftstack <- data.frame(Name=c('Jack', 'John', 'Mike', 'Michelle', 'Jhonny'),
LastName=c('Danials', 'Cena', 'Chandler', 'McCool', 'Nitro'),
Id=c(101, 102, 103, 104, 105),
Designation=c('CEO', 'Project Manager', 'Senior Dev', 'Junior Dev', 'Intern'))
fwrite(Delftstack, "C:\\DelftStack\\delftstack.csv")
The code above will write the given data frame to CSV. See the output:
Use write_csv
From Reader
Package to Export Data Frame to CSV in R
write_csv
is a function from the reader
package that can write data frames to CSV files; it takes two parameters: the data frame and the file path and name. See example:
library(readr)
Delftstack <- data.frame(Name=c('Jack', 'John', 'Mike', 'Michelle', 'Jhonny'),
LastName=c('Danials', 'Cena', 'Chandler', 'McCool', 'Nitro'),
Id=c(101, 102, 103, 104, 105),
Designation=c('CEO', 'Project Manager', 'Senior Dev', 'Junior Dev', 'Intern'))
write_csv(Delftstack, "C:\\DelftStack\\delftstack.csv")
The code above will write the given data frame to CSV. See the output:
The packages in these examples might be installed first if they are not already installed. We use \\
in each example to avoid backslash error.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook