How to Pass Command Line Arguments to R CMD BATCH and Rscript
-
Use the
commandArgs()
Function to Retrieve Arguments in R -
Use the
R CMD BATCH
Command to Redirect Output in R - Create the Script and Run the Command in R
-
Pass Command Line Arguments to
R CMD BATCH
Command in R -
Pass Command Line Arguments to
Rscript
in R
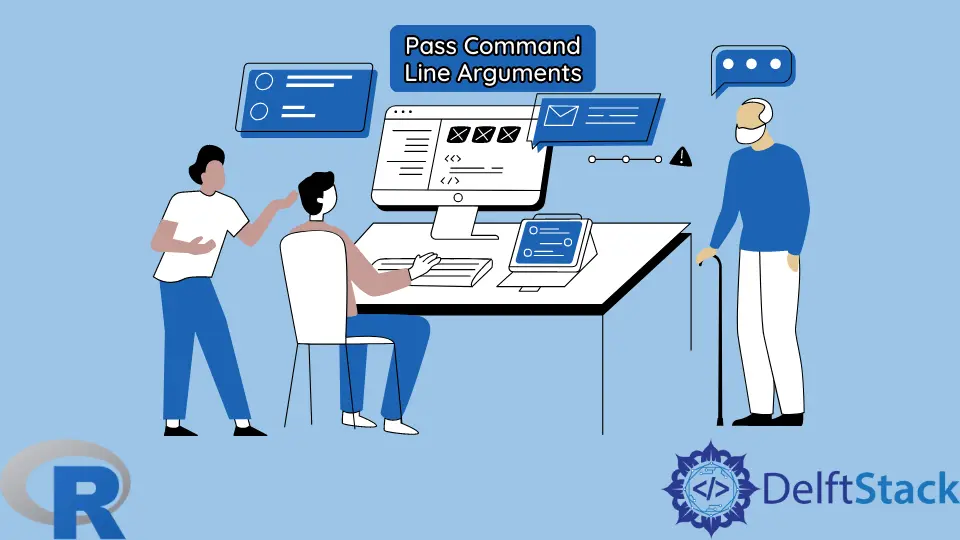
When using the command line to run an R script file, we may want to pass arguments and save the output in a file. This article demonstrates these techniques using the R CMD BATCH
command and the Rscript
front-end.
We will start with some details common to both methods.
Use the commandArgs()
Function to Retrieve Arguments in R
Arguments passed on the command line should be retrieved using the commandArgs()
function. We will use it with the argument trailingOnly=TRUE
.
The function returns a character vector.
Use the R CMD BATCH
Command to Redirect Output in R
The R CMD BATCH
command redirects the output to a .Rout
file. The file contains the input commands, the result and error messages.
With the Rscript
front-end, we have to redirect the output using the appropriate command for the shell/terminal. For several terminals, it is > filename
.
We can redirect the input using options(echo=TRUE)
at the beginning of the file. Error messages are not redirected.
We have to be careful when redirecting output to a file. The file will get created if it does not exist but will get overwritten otherwise.
Create the Script and Run the Command in R
To see how each method works, do the following.
-
Create a file with the given R code, and save it with the extension
.R
in a directory to execute R commands. -
Open the shell in the directory containing the script file.
-
Run the appropriate command after replacing the given file names with the names of your files.
-
The first argument is
hobby
, and the second isyears
in these sample scripts.
Pass Command Line Arguments to R CMD BATCH
Command in R
The syntax is below.
R CMD BATCH options "--args userargument1 userargument2" script_filename.R
Arguments cannot contain spaces. The exact quoting will depend on the shell in use.
The --vanilla
option combines several R options.
Example code for the R file:
# Create 5 values in a sequence.
x = seq(from=0, to=20, by=5)
print(x)
# Get the arguments as a character vector.
myargs = commandArgs(trailingOnly=TRUE)
myargs
# The first argument is the text for hobby.
hobby = myargs[1]
hobby
# The second argument is the number of years of practice.
years = as.numeric(myargs[2])
years
The command:
R CMD BATCH --vanilla "--args Golf 8" script_filename.R
An output file of the same name as the input file, with the .Rout
extension, gets created in the input file’s same directory.
Pass Command Line Arguments to Rscript
in R
Rscript
is a scripting front-end for R.
Arguments may contain spaces. Such arguments need to be in quotes.
The syntax is below.
Rscript script_filename.R 'user argument 1' userargument2 > rscript_output_file_name.txt
Example code for the R file:
# To print the command and its result in the output.
options(echo=TRUE)
# five random values from the standard normal distribution
x = rnorm(5)
print(x)
# Get the arguments as a character vector.
myargs = commandArgs(trailingOnly=TRUE)
myargs
# The first argument is the text for hobby.
hobby = myargs[1]
hobby
# The second argument is the number of years of practice.
years = as.numeric(myargs[2])
years
The command:
Rscript script_filename.R 'Playing Guitar' 3 > rscript_output_file_name.txt
The output file gets created. It has the input and output but not error messages.
References and Help
See Appendix B, Invoking R, of the manual: An Introduction to R.
Also, refer to R’s documentation of the commandArgs()
function and the Rscript
tool.