How to Apply Function With Multiple Arguments in R
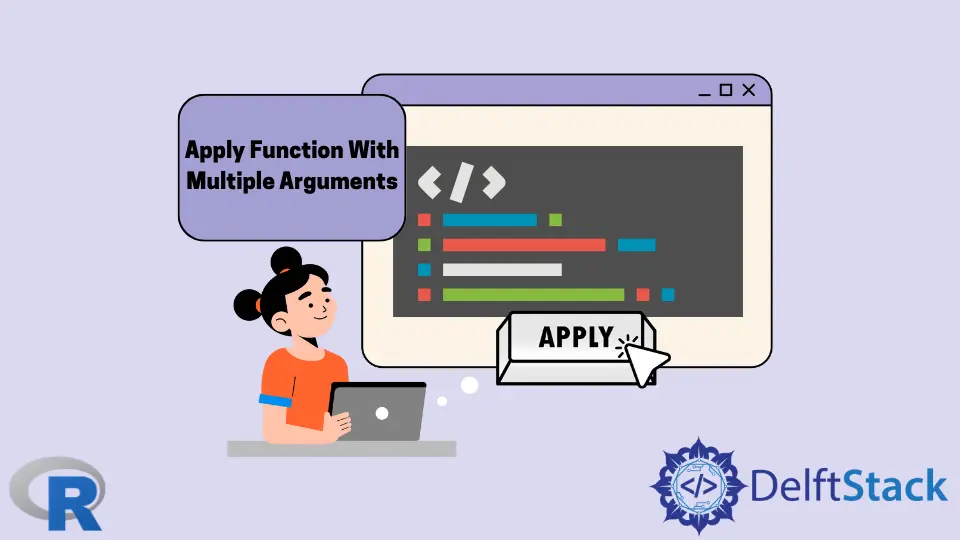
Functions are essential in any programming language. A function is a block of code that can be called to perform a specific operation in programming.
In R, we have built-in functions as well as user-defined functions. We can also apply a function directly to a list or vector with one or multiple arguments.
In this tutorial we will work with the following vectors and function:
f1 <- function(v1,v2){
v1+v2
}
vec1 <- c(1,5,9)
vec2 <- c(2,7,6)
The function is relatively simple, it just adds two elements and we have two vectors with three elements each.
In this tutorial, we will work with sapply()
, lapply()
, and the mapply()
functions, where we will apply a function to the whole vector and pass multiple parameters to the same, and pass the vectors to the function as parameters.
In situations where we want to apply a function to a given vector or list, we can use lapply()
or sapply()
.
The lapply()
function returns a list as the final output. For example:
lapply(vec1,f1,5)
[[1]]
[1] 6
[[2]]
[1] 10
[[3]]
[1] 14
typeof(lapply(vec1,f1,5))
[1] "list"
As you can see, we pass the f1
function to vec1 and pass another argument 5, since the function takes two arguments, which simply adds 5 to all elements.
The sapply()
performs the same function as the lapply()
function but is considered to be more efficient of the two since it simplifies the output and the result is not necessarily a list.
The following code snippet shows how:
sapply(vec1,f1,5)
[1] 6 10 14
typeof(sapply(vec1,f1,5))
[1] "double"
Another interesting function available is the mapply()
. It applies a function to vectors that are passed as arguments. The function is applied to the first elements of the vectors, the second elements, and so on.
mapply(f1,vec1,vec2)
[1] 3 12 15
Notice that with the mapply()
function we are able to pass the vectors as multiple arguments to a function since it returns the sum of elements at the same position.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn