How to Find Mode of a Vector in R
- Use Custom Function to Find Mode of an R Vector
-
Use
map_dbl
to ApplyFindMode
Function to Each Data Frame Column in R
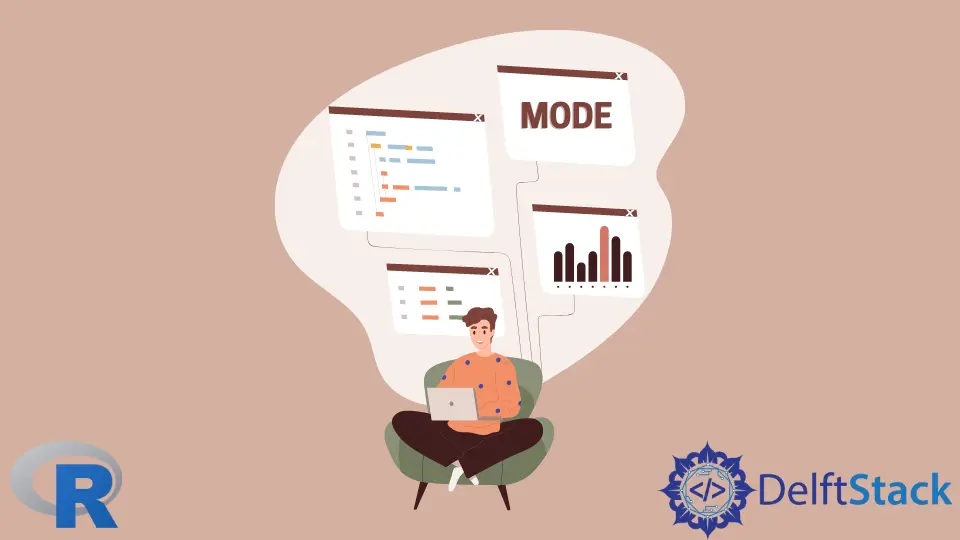
This article will explain several methods of how to find a mode of a vector in R.
Use Custom Function to Find Mode of an R Vector
Mode is one of the most basic statistical concepts denoting the maximum occurrence value in a set of values. As a result, it can be observed in different data types, such as numeric or character-based. R language does not have a built-in function for calculating the mode, but we can implement it using the functions: unique
, which.max
, tabulate
and match
. We define a function named FindMode
that takes one argument denoted as x
. At first, the unique
function is called on x
and stored in a separate object. unique
extracts non-duplicate values from the set. It can take a vector object, data frame, or an array as the first argument.
Then, we have multiple chained functions starting with match
that takes two vectors and returns a vector of position matches from them. tabulate
function counts and returns the number of times each integer appears in a vector. Note that the returned vector contains a count for every integer that is less or equal to the maximum integer in the argument vector. Finally, the which.max
function finds the index of the maximum element in the integer vector. We can invoke the FindMode
function on every column of the data frame using the apply
function. In this case, we declare a simple integer vector stored as a data frame and then passed to the apply
function to calculate the mean.
library(purrr)
FindMode <- function(x) {
u <- unique(x)
u[which.max(tabulate(match(x, u)))]
}
x <- c(12, 44, 3, -4.2, 3, 3.2, 54, 4, -11, -8, 2.5)
df <- data.frame(x)
apply(df, 2, FindMode)
Output:
x
3
Alternatively, we can call the FindMode
function on the data frame directly. The following example code demonstrates the usage with the cars
dataset included in the datasets
package.
library(purrr)
FindMode <- function(x) {
u <- unique(x)
u[which.max(tabulate(match(x, u)))]
}
apply(cars, 2, FindMode)
Output:
speed dist
20 26
Use map_dbl
to Apply FindMode
Function to Each Data Frame Column in R
Another useful function to find the mean for each column of the given data frame is map_dbl
, which is part of the purrr
package included in the tidyverse
. Note that these methods are not to be called on the vector objects.
library(purrr)
FindMode <- function(x) {
u <- unique(x)
u[which.max(tabulate(match(x, u)))]
}
x <- c(12, 44, 3, -4.2, 3, 3.2, 54, 4, -11, -8, 2.5)
df <- data.frame(x)
map_dbl(df, FindMode)
map_dbl(cars, FindMode)
Output:
x
3
speed dist
20 26
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook