How to Multiply Matrix in R
Sheeraz Gul
Feb 02, 2024
R
R Matrix
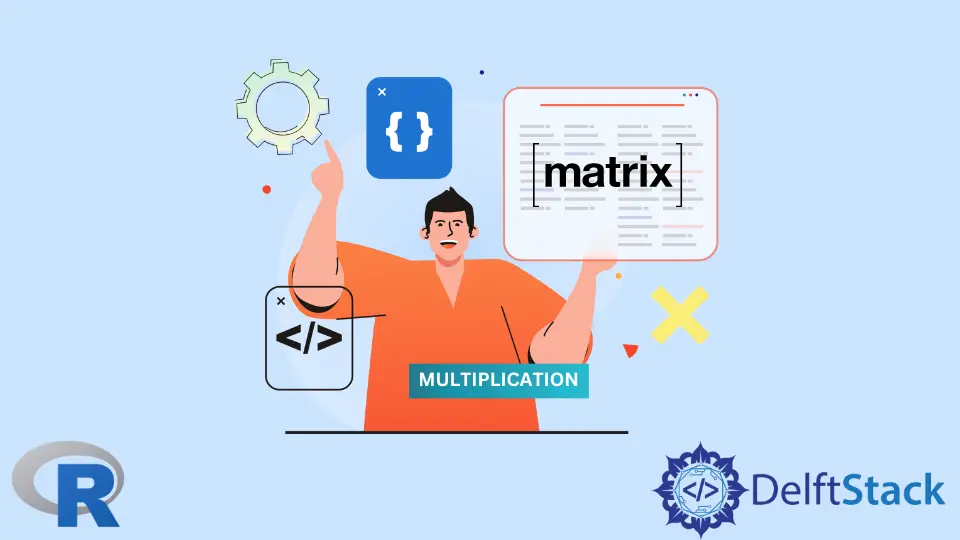
This tutorial demonstrates how to perform matrix multiplication in R.
Matrix Multiplication in R
In R, the %*%
operator performs multiplication between two matrices. The syntax is simple A %*% B
.
Let’s see an example.
demo_data <- c(2, 4, 2, 10, 41, 32, 20, 22, 61)
A <- matrix(demo_data, nrow = 3, ncol = 3)
demo_data1 <- c(10, 14, 21, 31, 50, 23, 11, 33, 23)
B <- matrix(demo_data1, nrow = 3, ncol = 3)
Multi_Result <- A %*% B
print("The Matrix A is: ")
print(A)
print("The Matrix B is: ")
print(B)
print("The Matrix Multiplication Result is:")
print(Multi_Result)
The code above will multiply two given matrices. See the output:
[1] "The Matrix A is: "
[,1] [,2] [,3]
[1,] 2 10 20
[2,] 4 41 22
[3,] 2 32 61
[1] "The Matrix B is: "
[,1] [,2] [,3]
[1,] 10 31 11
[2,] 14 50 33
[3,] 21 23 23
[1] "The Matrix Multiplication Result is:"
[,1] [,2] [,3]
[1,] 580 1022 812
[2,] 1076 2680 1903
[3,] 1749 3065 2481
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook