How to Compute Natural Logarithm in R
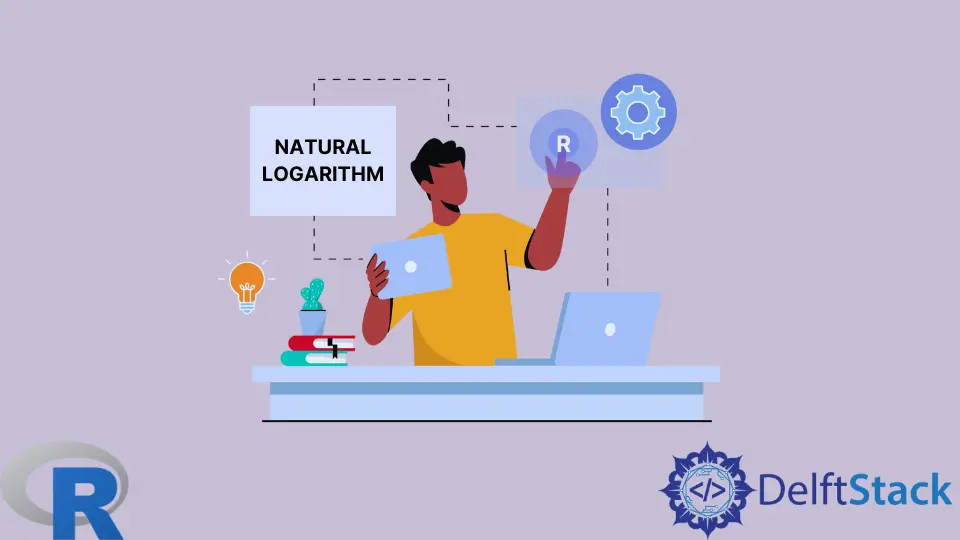
Logarithm, the inverse of the exponential, is very useful in solving complex non-linear exponential solutions.
The log()
function in R returns the natural logarithmic values. The log10()
and the log2()
functions have base 10 and 2 respectively. The following code snippet shows the use of these functions:
log(5)
[1] 1.609438
log10(5)
[1] 0.69897
log2(5)
[1] 2.321928
We can also specify the base in the log()
function using the base
parameter. The following example computes the log value of 5 with base 3:
log(5,3)
[1] 1.464974
The log1p()
function is also available in R, and it computes the natural logarithmic value of 1+n
, where n is the value passed to the function. The following example computes the log value of 1+4
:
log1p(4)
[1] 1.609438
Depending on the value and base of the logarithmic value required, we can also use them in equations. A sample equation is shown below:
y ~ a + b + log(10/x)
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn