How to Find Index of an Element in a R Vector
-
Use the
which()
Function to Find the Index of an Element in R -
Use the
match()
Function to Find the Index of an Element in R
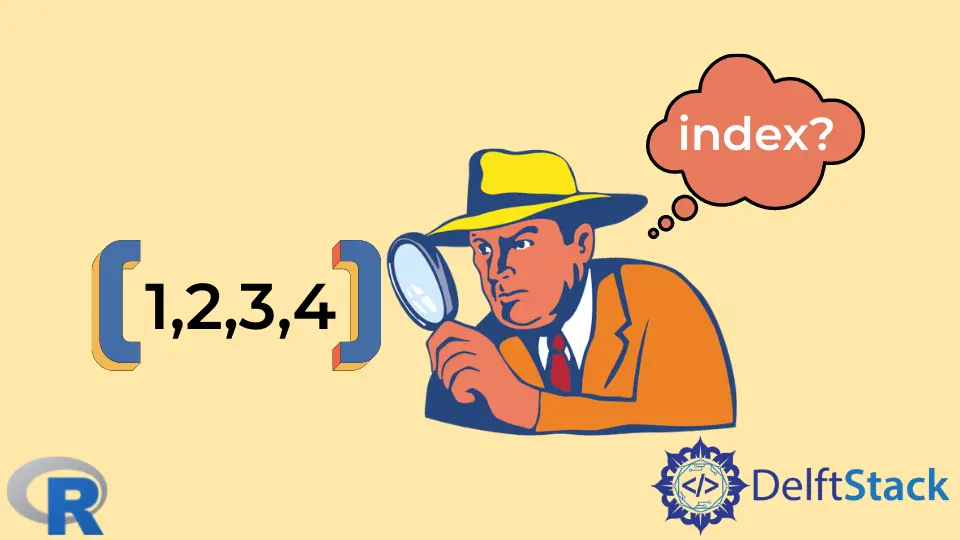
A vector is one of the fundamental data structures in R. It is used to store elements in a sequence, but unlike lists, all elements in a vector must be of the same data type.
Every element in the vector has a particular position or index. It is possible to know the index of any element using built-in functions in R like which()
, and match()
.
In this tutorial, we will find the index of element 7
and 8
of the following vector:
x <- c(5,6,8,9,7,5,7,8)
Use the which()
Function to Find the Index of an Element in R
The which()
function returns a vector with the index (or indexes) of the element which matches the logical vector (in this case ==
).
For example:
x <- c(5,6,8,9,7,5,7,8)
which(x == 7)
[1] 5 7
Notice that since 7
is present twice in the vector, both of its positions are returned. If we want the first index of 7
, we can simply do it as shown below:
which(x == 7)[1]
[1] 5
We can also use the which()
function to find the indexes of multiple elements using the %in%
parameter, which returns a vector with a True
value for every element that matches.
which(x %in% c(7,8))
[1] 3 5 7 8
As you can see, all the positions of both 7
and 8
are returned.
Use the match()
Function to Find the Index of an Element in R
The match()
function is very similar to the which()
function. It returns a vector with the first index (if the element is at more than one position as in our case) of the element and is considered faster than the which()
function.
x <- c(5,6,8,9,7,5,7,8)
match(7,x)
[1] 5
We can also use it for finding the first index of multiple elements, as shown below:
x <- c(5,6,8,9,7,5,7,8)
match(c(7,8),x)
[1] 5 3
Note that only the first positions of both 7
and 8
are returned by the match()
function. That is why the match()
is considered to be faster of the two methods for such situations where only the first index is required.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn