How to Get the Number of Columns in R
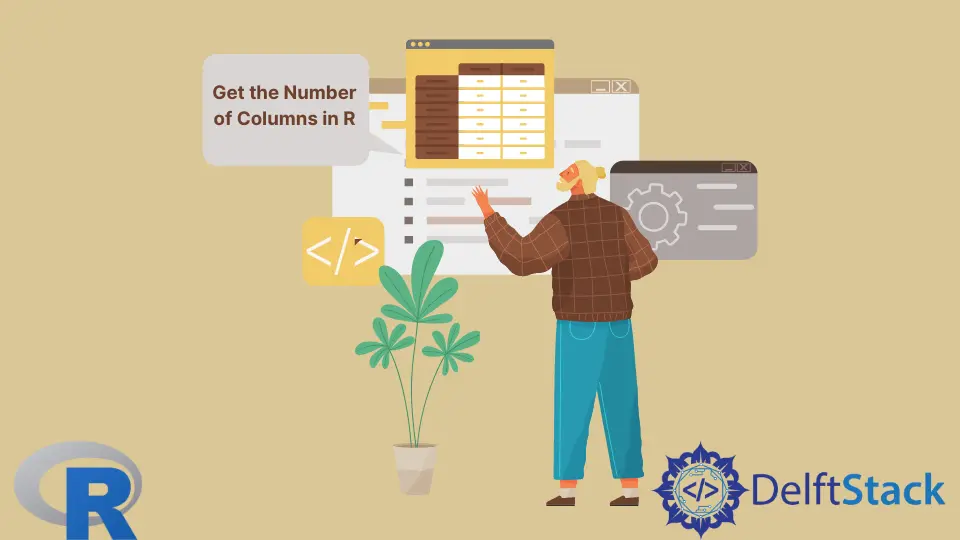
R has built-in functions to get the total number of columns and the number of a column by name. This tutorial demonstrates how to get the number of columns in R.
Get the Total Number of Columns in R
The built-in function ncol()
is used to get the total number of columns in the R language. It takes one parameter, the data frame.
Example code:
#create a data frame
Delftstack <- data.frame(Name=c('Jack', 'John', 'Mike', 'Michelle', 'Jhonny'),
LastName=c('Danials', 'Cena', 'Chandler', 'McCool', 'Nitro'),
Id=c(101, 102, 103, 104, 105),
Designation=c('CEO', 'Project Manager', 'Senior Dev', 'Junior Dev', 'Intern'))
#find number of columns
number_columns <- ncol(Delftstack)
#print the number of columns
cat("Number of columns in the Data Frame is:", number_columns)
The code above checks for the total number of columns in the data frame.
Output:
Number of columns in the Data Frame is: 4
Get the Number of Columns by Name in R
We can also get the column by name using the which()
method with the colnames(df)
method.
Example code:
#create a data frame
Delftstack <- data.frame(Name=c('Jack', 'John', 'Mike', 'Michelle', 'Jhonny'),
LastName=c('Danials', 'Cena', 'Chandler', 'McCool', 'Nitro'),
Id=c(101, 102, 103, 104, 105),
Designation=c('CEO', 'Project Manager', 'Senior Dev', 'Junior Dev', 'Intern'))
# Get the column number by name using Which method
which( colnames(Delftstack)=="Id" )
The code above will get the number of a column by column name as the number of column ID is 3
.
Output:
[1] 3
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook