How to Append Elements to a List in R
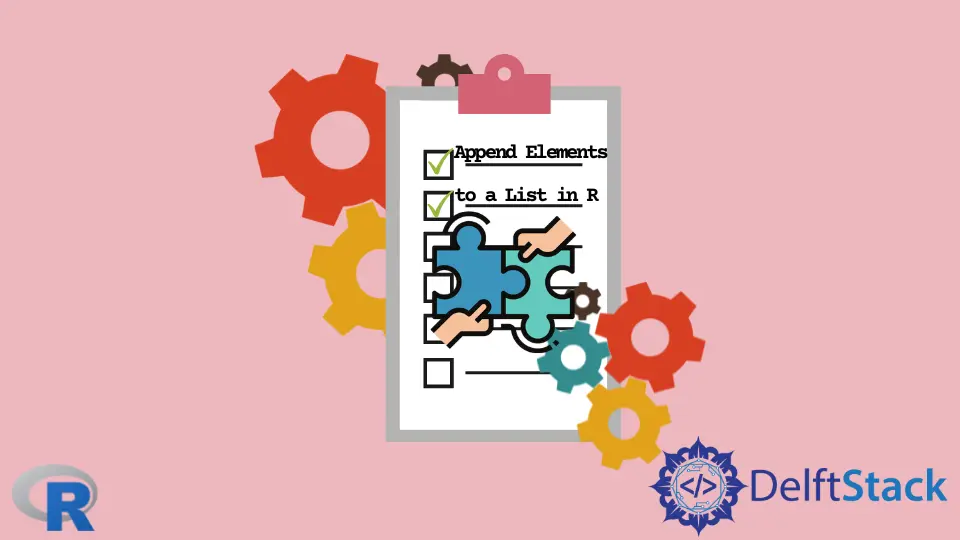
There are many ways to use a loop to add an arbitrary number of elements to a list in R. Some of them could become sluggish when the number of elements is high. This tutorial will introduce the most efficient ways to append elements to a list in an R loop.
A common way to implement such an algorithm would be to create a while
loop and use the c
(concatenate) command within the loop. Within each iteration, an element is added by using the c
function. In this example, a value (i*3
) is added to a list until the index variable reaches 10,000:
i <- 1
mylist <- c()
while (i<10) {
mylist <- c(mylist, i*3)
i <- i+1
}
cat(mylist)
Output:
3 6 9 12 15 18 21 24 27
The problem with the previous example is that each time the concatenation is executed, the whole list is copied. When working with large data sets, the time it takes to add all the elements could be tremendous.
Append to a List in R With the length
Function
A more efficient approach to append an arbitrary number of elements to a list within a loop is to use the length
function in each iteration. With the length
function, you can get the number of elements in the list and assign each new element to the index behind the last element: length(mylist) + 1
.
The below example does the same as the previous one, but more efficiently.
i <- 1
mylist <- c()
while (i<10) {
mylist[[(length(mylist) + 1)]] <- i*3
i <- i+1
}
cat(mylist)
Output:
3 6 9 12 15 18 21 24 27
Setting an Upper Bound for the List
If you know beforehand how many times your loop will iterate, then instead of growing a list of elements, you can define a vector of fixed length and use it to hold all the elements. For example, if you know your loop will iterate 10,000 times, you can initialize the vector this way:
mylist <- vector("list", 10000)
After this, you can use a for
loop and set the value of each element by its index:
i <- 1
for (i in 1:10000) {
mylist[[i]] <- i*3
}
If you don’t know the number of iterations, but you have an upper bound for them, you can define the vector with this upper bound. Later, when the loop has finished executing, you can pick only the non-null elements. For example, if you know that your loop won’t iterate more than 10,000 times, you can initialize your vector with 10,000 elements, even if you will not use them all.