How to Zip Lists in Python
- What is List Zipping in Python?
-
Using the
zip()
Function - Unzipping Lists
- Handling Different Lengths of Lists
- Conclusion
- FAQ
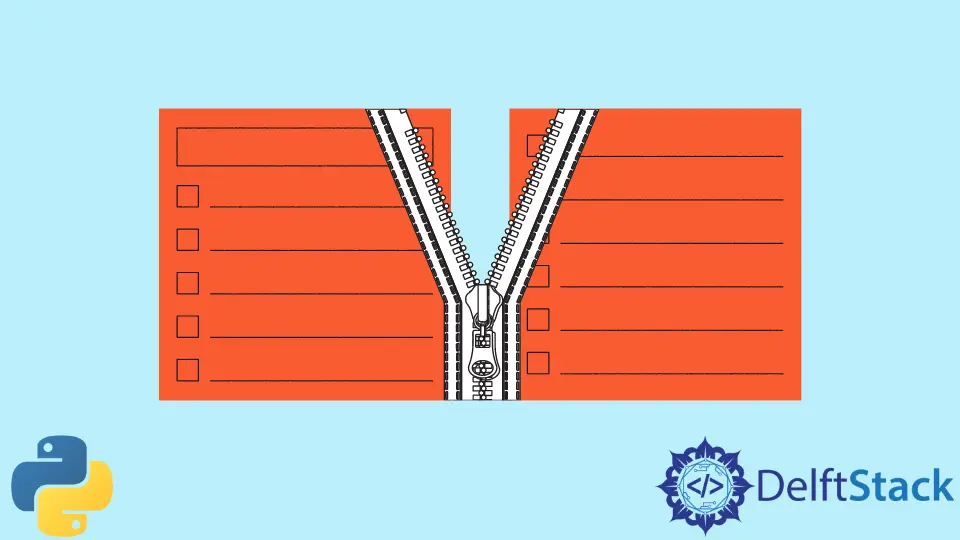
In the world of programming, data manipulation is a fundamental skill. One common operation is zipping lists together, which allows you to pair elements from multiple lists into tuples. This can be incredibly useful when you want to combine related data or iterate over multiple sequences simultaneously.
In this tutorial, we will explore how to zip lists in Python, covering various methods and providing clear code examples. Whether you’re a beginner or an experienced Python developer, understanding how to zip lists will enhance your coding toolkit. So, let’s dive into the fascinating world of Python list zipping!
What is List Zipping in Python?
List zipping refers to the process of combining two or more lists into a single iterable where each element is a tuple containing elements from the input lists at the same index. The built-in zip()
function in Python makes this process simple and efficient. When the lists are of different lengths, zip()
will stop creating tuples when the shortest input iterable is exhausted. This feature is useful for ensuring that you don’t run into index errors during iterations.
Let’s see how the zip()
function works with a basic example.
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
zipped = zip(list1, list2)
zipped_list = list(zipped)
print(zipped_list)
Output:
[(1, 'a'), (2, 'b'), (3, 'c')]
In this example, list1
and list2
are combined into a new list of tuples. Each tuple contains one element from list1
and one from list2
, making it easy to work with related data.
Using the zip()
Function
The simplest and most common way to zip lists in Python is by using the built-in zip()
function. This function takes multiple iterable arguments and returns an iterator of tuples. Each tuple contains elements from the input lists at corresponding positions.
Here’s a more detailed example to illustrate how zip()
works.
names = ['Alice', 'Bob', 'Charlie']
ages = [25, 30, 35]
zipped_info = zip(names, ages)
zipped_info_list = list(zipped_info)
print(zipped_info_list)
Output:
[('Alice', 25), ('Bob', 30), ('Charlie', 35)]
In this case, we have two lists: one with names and another with ages. By zipping them together, we create a new list that pairs each name with its corresponding age. This method is efficient and straightforward, making it a go-to option for many developers.
The zip()
function can handle more than two lists as well. If you have three or more lists, it will still create tuples containing elements from all of them.
cities = ['New York', 'Los Angeles', 'Chicago']
states = ['NY', 'CA', 'IL']
populations = [8419600, 3980400, 2716000]
zipped_cities = zip(cities, states, populations)
zipped_cities_list = list(zipped_cities)
print(zipped_cities_list)
Output:
[('New York', 'NY', 8419600), ('Los Angeles', 'CA', 3980400), ('Chicago', 'IL', 2716000)]
Here, we have combined three lists: cities, states, and populations. The result is a list of tuples where each tuple contains a city, its state abbreviation, and its population. This demonstrates how versatile the zip()
function can be in organizing related data.
Unzipping Lists
Sometimes, you may find yourself needing to unzip a list of tuples back into separate lists. This can be accomplished using the zip()
function in conjunction with the unpacking operator *
. Let’s take a look at how this works.
zipped_data = [('Alice', 25), ('Bob', 30), ('Charlie', 35)]
names_unzipped, ages_unzipped = zip(*zipped_data)
print(names_unzipped)
print(ages_unzipped)
Output:
('Alice', 'Bob', 'Charlie')
(25, 30, 35)
In this example, we start with a list of tuples that contains names and ages. By using the unpacking operator *
, we can easily separate the names and ages back into their respective lists. This is particularly useful in scenarios where you need to manipulate or analyze the data in its original format.
Handling Different Lengths of Lists
When zipping lists of different lengths, it’s important to understand how Python handles this situation. The zip()
function will only iterate up to the length of the shortest list, discarding any extra elements from the longer lists. This behavior can be beneficial or problematic, depending on your use case.
Let’s see an example where we have lists of unequal lengths.
list1 = [1, 2, 3, 4]
list2 = ['a', 'b']
zipped_diff = zip(list1, list2)
zipped_diff_list = list(zipped_diff)
print(zipped_diff_list)
Output:
[(1, 'a'), (2, 'b')]
In this scenario, list1
has four elements while list2
has only two. The zip()
function stops at the second index, resulting in a list of two tuples. If you need to include all elements, you may want to consider using the itertools.zip_longest()
function from the itertools
module, which fills in missing values with a specified fill value.
from itertools import zip_longest
zipped_longest = zip_longest(list1, list2, fillvalue='N/A')
zipped_longest_list = list(zipped_longest)
print(zipped_longest_list)
Output:
[(1, 'a'), (2, 'b'), (3, 'N/A'), (4, 'N/A')]
In this case, zip_longest()
allows us to combine the lists while filling in the missing values with ‘N/A’. This is particularly useful when dealing with datasets that may not always align perfectly.
Conclusion
Zipping lists in Python is a powerful technique that enables developers to efficiently combine and manipulate data. The built-in zip()
function provides a straightforward way to pair elements from multiple lists, while the zip_longest()
function from the itertools
module offers added flexibility when dealing with lists of different lengths. Understanding how to zip and unzip lists can significantly enhance your data handling capabilities in Python, making your code cleaner and more efficient. Whether you’re organizing related data or preparing it for analysis, mastering list zipping is an essential skill for any Python programmer.
FAQ
-
What is the zip() function in Python?
The zip() function combines elements from multiple lists into tuples based on their index positions. -
Can I zip more than two lists in Python?
Yes, the zip() function can handle multiple lists, creating tuples that contain elements from all of them. -
What happens if the lists have different lengths?
The zip() function will stop creating tuples when the shortest list is exhausted, discarding any extra elements from the longer lists. -
How can I unzip a list of tuples back into separate lists?
You can use the zip() function with the unpacking operator (*) to easily separate the tuples back into individual lists. -
What is zip_longest() in Python?
zip_longest() is a function from the itertools module that combines lists of different lengths, filling in missing values with a specified fill value.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python