How to Solve the ValueError: Zero Length Field Name in Format Error in Python
- Understanding the ValueError: Zero Length Field Name in Format
- Solution 1: Use Named Placeholders
- Solution 2: Use Positional Arguments
- Solution 3: Upgrade Your Python Version
- Conclusion
- FAQ
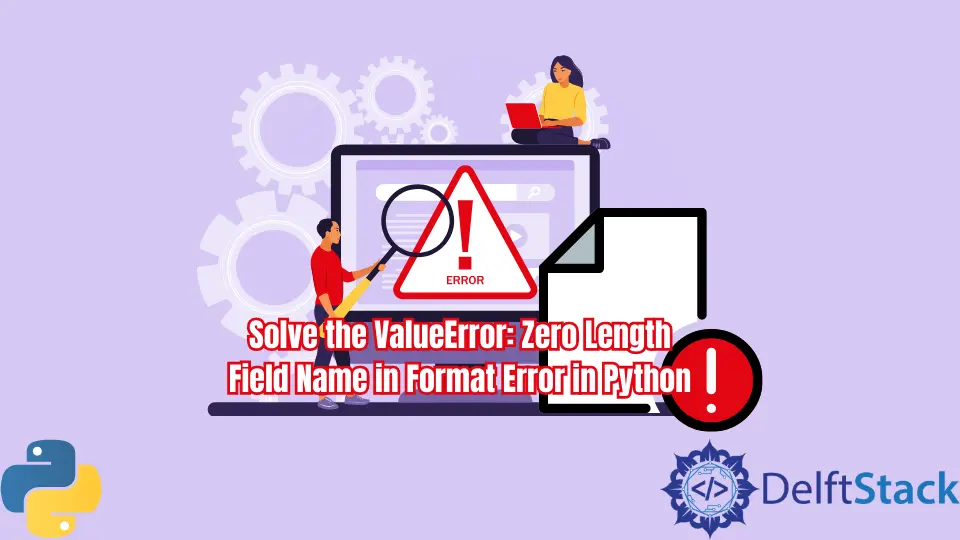
When working with Python, encountering errors is a common part of the development process. One error that can be particularly confusing is the ValueError: zero length field name in format. This issue arises when using the str.format()
method incorrectly, often due to an oversight in how placeholders are defined.
In this tutorial, we’ll break down this error, explore its causes, and provide clear, effective solutions to help you resolve it. Whether you’re a beginner or an experienced programmer, understanding how to tackle this error will enhance your coding skills and improve your overall experience with Python.
Understanding the ValueError: Zero Length Field Name in Format
The ValueError: zero length field name in format occurs when you attempt to use the str.format()
method without properly defining the placeholders in your string. Introduced in Python 2.7 and 3.0, the str.format()
method allows for more readable and flexible string formatting. However, if you use empty curly braces {}
without specifying a field name or index, Python raises this ValueError.
For example, consider the following code snippet:
name = "Alice"
greeting = "Hello, {}!"
formatted_greeting = greeting.format(name)
Output:
Hello, Alice!
In this case, the placeholder {}
is correctly filled with the variable name
. However, if you try to use an empty placeholder without a corresponding argument, like this:
message = "Value: {}"
formatted_message = message.format()
Output:
ValueError: zero length field name in format
This will trigger the ValueError. Understanding the root cause of this error is the first step in resolving it.
Solution 1: Use Named Placeholders
One effective way to avoid the ValueError is to use named placeholders in your format strings. Named placeholders allow you to specify the variable names directly within the string, which can make your code more readable and prevent errors related to missing arguments.
Here’s how you can implement named placeholders:
name = "Alice"
age = 30
message = "My name is {name} and I am {age} years old."
formatted_message = message.format(name=name, age=age)
Output:
My name is Alice and I am 30 years old.
By using named placeholders, you explicitly define which variable corresponds to which part of the string. This approach not only prevents the zero length field name error but also enhances the clarity of your code. When you use named placeholders, you can easily see which values are being inserted into the string, making debugging simpler.
In addition, if you accidentally forget to provide a value for one of the named placeholders, Python will raise a more informative error, indicating which variable is missing. This can save you time and frustration when troubleshooting your code.
Solution 2: Use Positional Arguments
Another way to avoid the ValueError is to use positional arguments in your format strings. Positional arguments allow you to specify the order in which the values should be inserted into the string. This method can be particularly useful when you want to format a string with multiple variables.
Here’s an example of using positional arguments:
first_name = "Alice"
last_name = "Smith"
message = "Hello, {0} {1}!"
formatted_message = message.format(first_name, last_name)
Output:
Hello, Alice Smith!
In this case, {0}
corresponds to the first argument passed to format()
, and {1}
corresponds to the second argument. By using positional arguments, you ensure that the correct values are inserted into the string without triggering the ValueError.
It’s important to note that when using positional arguments, the order in which you provide the arguments to the format()
method must match the order of the placeholders in the string. If you mistakenly provide fewer arguments than there are placeholders, you’ll encounter a different error, but it won’t be the zero length field name error.
Using positional arguments can make your code concise and straightforward, especially when dealing with simple formatting scenarios.
Solution 3: Upgrade Your Python Version
If you are using an older version of Python, you may encounter the ValueError due to changes in how string formatting is handled in newer versions. Starting from Python 3.1, empty curly braces are allowed, and the formatting behavior has been improved. Therefore, upgrading your Python version can help resolve this error.
To check your current Python version, you can use the following command in your terminal or command prompt:
python --version
If you find that you are using an outdated version, consider upgrading to the latest stable release. You can do this using the following command:
pip install --upgrade python
After upgrading, you can try running your code again. This should eliminate the ValueError, as newer versions of Python handle string formatting more gracefully.
Upgrading not only resolves this specific issue but also provides you with access to new features and improvements in the language. Staying updated with the latest version of Python is a good practice for any developer, as it ensures that you are using the most efficient and secure version of the language.
Conclusion
In summary, the ValueError: zero length field name in format can be a frustrating hurdle when working with Python strings. However, by understanding the root cause of the error and implementing effective solutions—such as using named placeholders, positional arguments, or upgrading your Python version—you can easily overcome this issue. Remember that clear and readable code is essential for maintaining and debugging your projects. By applying these techniques, you’ll not only resolve the error but also enhance your overall coding skills.
FAQ
-
What causes the ValueError: zero length field name in format?
This error occurs when you use empty curly braces in thestr.format()
method without providing a corresponding argument. -
How can I avoid the ValueError when formatting strings in Python?
You can avoid this error by using named placeholders or positional arguments, ensuring that you always provide the correct number of arguments. -
Is the ValueError related to a specific version of Python?
Yes, this error can occur in older versions of Python. Upgrading to a newer version can help resolve the issue. -
What is the best practice for string formatting in Python?
Using f-strings (formatted string literals) introduced in Python 3.6 is often considered the best practice for string formatting due to their readability and efficiency.
- Can I still use the older formatting methods in Python?
Yes, while newer methods like f-strings are recommended, you can still use the older%
formatting andstr.format()
methods if needed.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python