XOR in Python
-
Get XOR in Python Using the
^
Operator - Get XOR in Python Using Logical Operators
-
Get XOR in Python Using the Built-In
xor()
Method
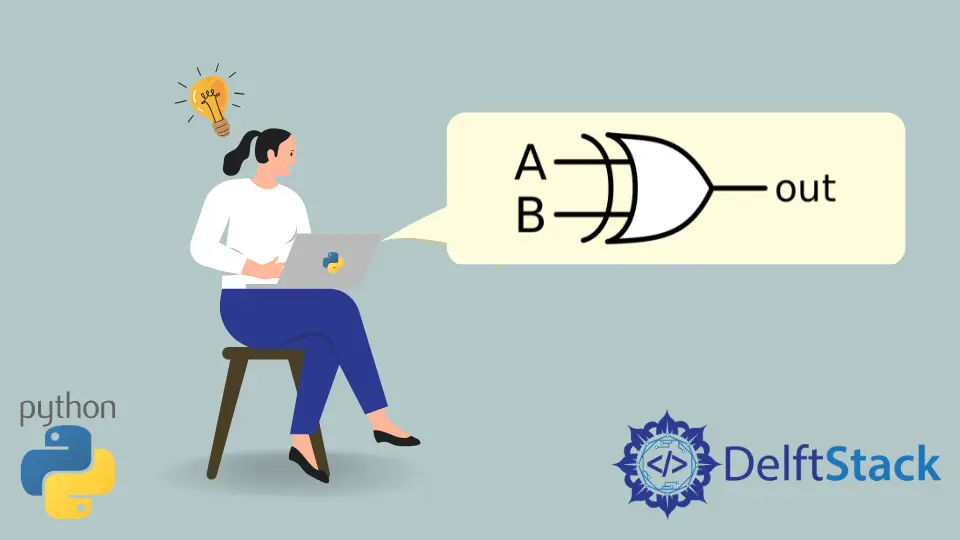
This tutorial will explain multiple ways to perform XOR (exclusive OR) operation on two variables in Python. The XOR operation is usually used in different protocols like in error checking or in situations where we do not want two conditions to be true at the same time.
Get XOR in Python Using the ^
Operator
The ^
operator in Python is for bitwise XOR and can also be used on booleans. The below code examples demonstrate how we can perform XOR operation on the Booleans and bitwise XOR operation on the integers.
Python XOR Operation Example 1:
a = bool(1)
b = bool(0)
print(a ^ b)
Output:
True
Python XOR Operation Example 2:
a = 3
b = 5
print(a ^ b)
Output:
6
3
in binary is 0b11
and 5
is 0b101
, so the XOR of 0b011
and 0b101
will be 0b110
, which is 6
in decimal.
Get XOR in Python Using Logical Operators
We can implement a user-defined xor()
method using logical operators in Python. The below code example shows how we can implement the xor()
method on booleans using and
, or
and not
operators in Python.
Example code:
def xor(x, y):
return bool((x and not y) or (not x and y))
print(xor(0, 0))
print(xor(0, 1))
print(xor(1, 0))
print(xor(1, 1))
Output:
False
True
True
False
Get XOR in Python Using the Built-In xor()
Method
The xor()
method of the operator
module of Python can also be used to get XOR of Booleans and integers. The functionality of xor()
method in Python is the same as the ^
operator. It also performs bitwise XOR operation on integers and XOR operation on the booleans. The below example code explains how to use the xor()
method to apply XOR on booleans and integers.
from operator import xor
print(xor(bool(0), bool(0)))
print(xor(5, 3))
Output:
False
6