What Is the Difference Between List Methods Append and Extend
-
Python List
append
Method -
Python List
extend
Method -
Conclusion of Difference Between
append
andextend
in Python List
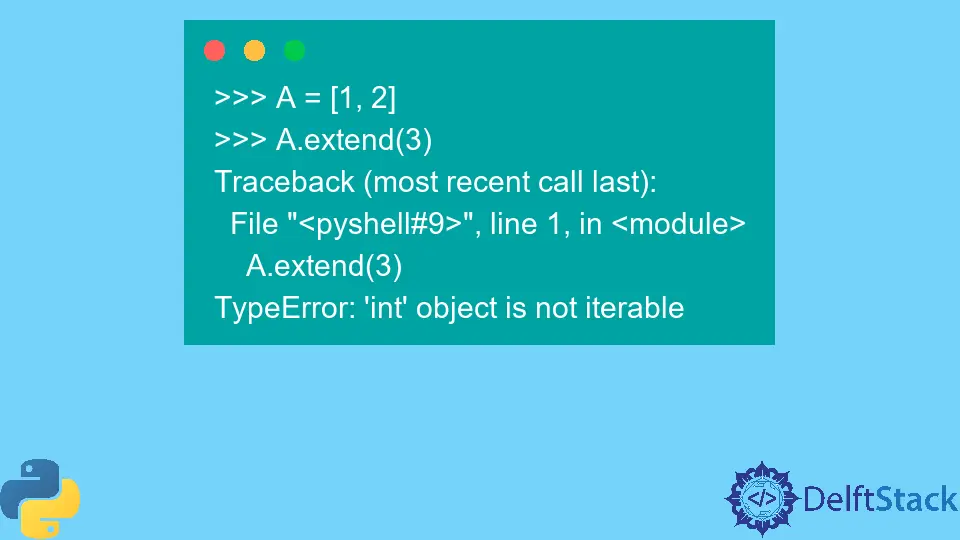
This article introduces the difference between list append
and extend
methods in Python.
Python List append
Method
append
adds the object to the end of the list. The object could be any data type in Python, like list, dictionary or class object.
>>> A = [1, 2]
>>> A.append(3)
>>> A
[1, 2, 3]
>>> A.append([4, 5])
>>> A
[1, 2, 3, [4, 5]]
The length of the list will increase by one after the append
is done.
Python List extend
Method
extend
extends the list by appending elements from the iterable argument. It iterates over the argument and then adds each element to the list. The given argument must be iterable type, like list, otherwise, it will raise TypeError
.
>>> A = [1, 2]
>>> A.extend(3)
Traceback (most recent call last):
File "<pyshell#9>", line 1, in <module>
A.extend(3)
TypeError: 'int' object is not iterable
If you want to add 3
to the end of the list, you should firstly put the 3
in a new list.
>>> A = [1, 2]
>>> A.extend([3])
>>> A
[1, 2, 3]
extend
method iterates the elements in the iterable object and then adds them one to one to the end of the list.
>>> A = [1, 2]
>>> A.extend([3, 4])
>>> A
[1, 2, 3, 4]
Python extend
String Type
Be aware that when the given object is a string
type, it will append each character in the string to the list.
>>> A = ["a", "b"]
>>> A.extend("cde")
>>> A
['a', 'b', 'c', 'd', 'e']
Conclusion of Difference Between append
and extend
in Python List
append
adds the given object to the end of the list, therefore the length of the list increases only by 1.
On the other hand, extend
adds all the elements in the given object to the end of the list, therefore the length of the list increases by the length of the given object.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook