What Is Difference Between Del, Remove and Pop on Python Lists
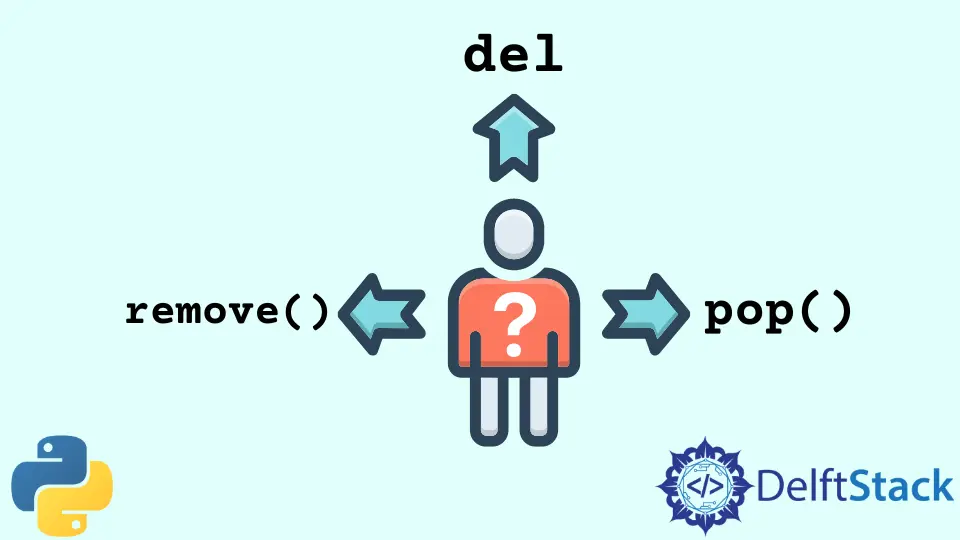
As it often goes, Python offers several means to achieve the same thing.
If you need to delete an element from a list, you may use any of
list.remove()
or list.pop()
functions or the built-in del
statement.
In the examples below, we will cover each of these approaches and discuss their differences and trade-offs.
Python List Removing by Value
list.remove()
removes the first item in the list, whose value is equal to the passed-in argument.
When using this method, you usually don’t know or care what the index of the item to be removed is.
>>> names = ['Bob', 'Cindy', 'Noah']
>>> names.remove('Cindy')
>>> names
['Bob', 'Noah']
Be careful when using this method.
If an item you are trying to delete doesn’t exist in the list,
the Python runtime will throw a ValueError
.
>>> names = ['Bob', 'Noah']
>>> names.remove('Cindy')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: list.remove(x): x not in list
To navigate around this issue, you will have to check if an item
exists in the list before trying to list.remove()
it.
>>> names = ['Bob', 'Noah']
>>> if 'Cindy' in names:
... names.remove('Cindy')
...
>>>
Python List Removing by Index
If you know the index of an element you want to remove, you can use the list.pop()
method.
>>> names = ['Bob', 'Cindy', 'Noah']
>>> names.pop(1)
'Cindy'
>>> names
['Bob', 'Noah']
list.pop()
removes an element at the specified index and returns it.
If you don’t give any index to list.pop()
, it will remove the last element of the list.
>>> names = ['Bob', 'Cindy', 'Noah']
>>> names.pop()
'Noah'
>>> names
['Bob', 'Cindy']
Python List Removing by del
Another option to remove an element from a list by index is to use the del
built-in statement.
Unlike list.pop()
the del
statement doesn’t return a removed value.
>>> names = ['Bob', 'Cindy', 'Noah']
>>> del names[1]
>>> names
['Bob', 'Noah']
The del
statement is also more flexible that list.pop()
.
You can use it to remove ranges of values from the list.
>>> names = ['Bob', 'Cindy', 'Noah']
>>> del names[0:2]
>>> names
['Noah']
You can also use it to clear the entire list.
>>> names = ['Bob', 'Cindy', 'Noah']
>>> del names[:]
>>> names
[]
As you can see from the above, each approach has slightly different use cases.
Still, if all you need is to remove a single element from the list without remembering its value, then either method will work.
Use the one that reads better in the broader context of your code.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python