The Walrus Operator := in Python
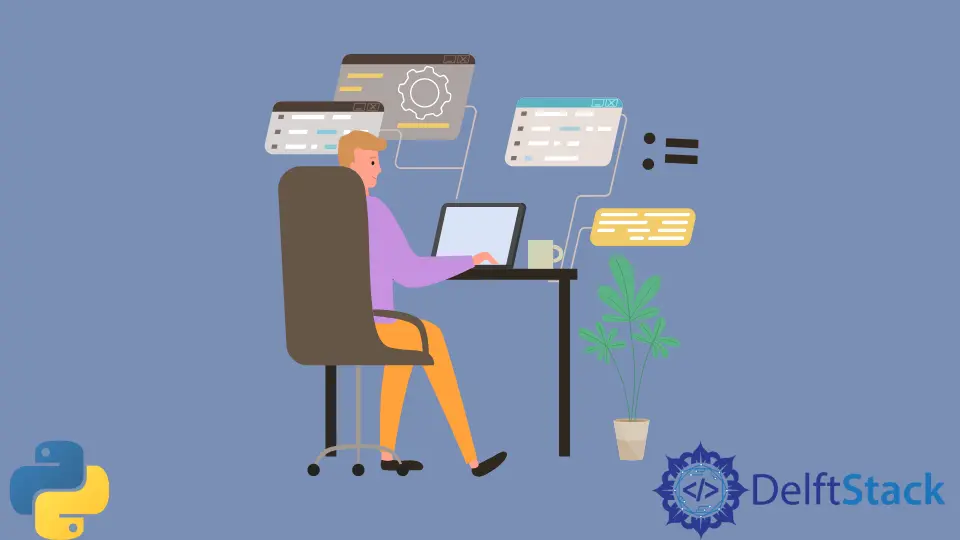
Python users receive regular updates as new changes are made, and features are added in every update. With Python 3.8, the walrus operator :=
was introduced. It’s an assignment operator.
We will discuss the walrus operator in this tutorial.
Walrus Operator in Python
Unlike the traditional assignment operator =
, which assigns the values, the walrus operator :=
can assign the value and simultaneously return the variable. This operator is a helpful feature for assigning values within an expression.
We use parentheses to assign a value to a variable while using this operator.
For example:
(a := 5)
print(a)
Output:
5
The walrus operator is useful as it allows us to avoid repeated statements in many cases. We can assign the values to a variable, which may not exist yet, in the context of an expression.
a = [1, 2, 3]
if (n := len(a)) > 5:
pass
print(n)
Output:
3
The above code will give an error if we use the traditional assignment operator. However, since we use the walrus operator, we could define the value for n
in the expression and use it afterward.
Now let us take another example.
a = [1, 2, 3]
d = {1: len(a), 2: sum(a), 3: sum(a) / len(a)}
print(d)
Output:
{1: 3, 2: 6, 3: 2.0}
In the above example, we calculate the length and sum of the list twice. We can avoid this using the walrus operator.
Using this operator, we can assign the variable for length and sum within the expression.
For example:
a = [1, 2, 3]
d = {1: (n := len(a)), 2: (s := sum(a)), 3: (n / s)}
print(d, n, s)
Output:
{1: 3, 2: 6, 3: 0.5} 3 6
Here, we used the walrus operator to assign variables n
and s
within the expression. Then, we use these variables within the expression and display them afterward.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn