Virtualenv in Python3
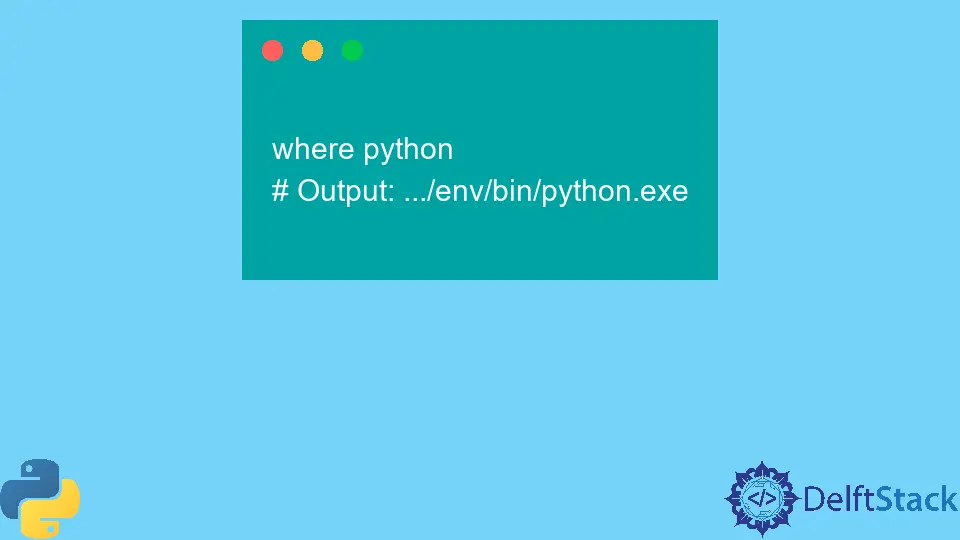
A virtual environment is an independent directory tree that accommodates the Python installation for a specific version of Python, along with a certain number of additional packages.
Virtualenv
can be defined as a tool that creates isolated Python environments. In the versions of Python 3.3 and above, a subset of it has been coordinated into the standard library, which can be accessed by the venv
module.
This tutorial will discuss what a virtual environment is and how to create it in Python 3.
Use the venv
Module in Python
In Python 2, the virtualenv
module was used for creating and managing virtual environments. It was replaced with the venv
module in Python 3.
The venv
module offers support for generating lightweight virtual environments along with their site directories, which can be segregated from the system directories if the user wants.
Creating the Virtual Environment
Whenever a user needs to switch projects, they can simply generate a new virtual environment and do not need to worry about the disintegration of the packages installed in the other existing environments. Using a virtual environment for developing Python applications is always recommended.
The creation of a virtual environment can be done by using the following command.
python3 -m venv envname
The second argument in the above code is the location to generate the virtual environment. It can generally be created in the user’s project and can be given a name.
Activating a Virtual Environment
Before reaching the stage where we can install or use packages in the virtual environment, we first need to activate them. When a virtual environment is activated, it puts the virtual pip
and python
executables into your shell’s path
.
.\envname\Scripts\activate
Confirming our existence in the virtual environment can be done by checking the current location of the Python Interpreter.
where python
# Output: .../env/bin/python.exe
The pip
and python
commands will continue to work as long as the virtual environment is active and the Python application will be able to use and import packages.
Leaving the Virtual Environment
Switching to different projects and environments is possible in this case. Leaving a particular virtual environment for another one is also a possibility.
The following code uses a simple command used to leave the virtual environment.
deactivate
If there is a need to enter this particular virtual environment again, the above instructions can be followed, except we don’t activate the virtual environment. Re-creation of the virtual environment does not need to happen.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn