How to Get Values of Dictionary in Python
-
Use the
list()
Function to Get the Values of a Dictionary in Python -
Use the Unpack Operator
*
to Get the Values of a Dictionary in Python -
Use the
extend()
Function to Get the Values of a Dictionary in Python
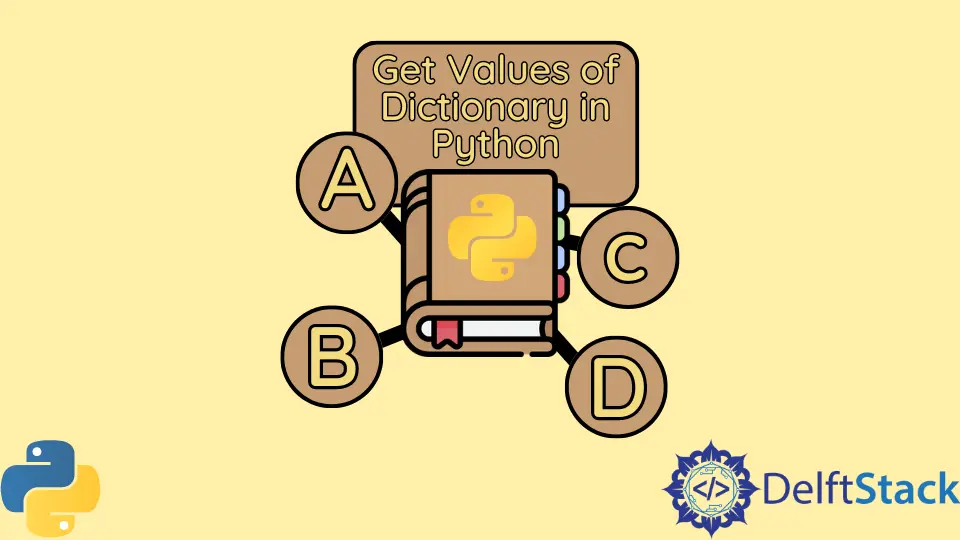
In this tutorial, we will learn how to get the values of a dictionary in Python.
The values()
method of the dictionary returns a representation of a dictionary’s values in a dict_values
object.
For example,
d = {"1": "a", "2": "b", "3": "c", "4": "d"}
print(d.values(), type(d.values()))
Output:
dict_values(['a', 'b', 'c', 'd']) <class 'dict_values'>
We will learn how to get the values of a dictionary by converting this object to a list.
Use the list()
Function to Get the Values of a Dictionary in Python
The list()
function can be used to type-cast and convert the dict_values
object returned by the values()
function to a proper list.
d = {"1": "a", "2": "b", "3": "c", "4": "d"}
lst = list(d.values())
print(lst)
Output:
['a', 'b', 'c', 'd']
Use the Unpack Operator *
to Get the Values of a Dictionary in Python
The *
operator in Python can be used to unpack elements from an iterable object. We can unpack all the items individually into a new list.
We can use this operator to get all the values of the dictionary in a list.
The following code shows how to use this operator with the values()
function in Python.
d = {"1": "a", "2": "b", "3": "c", "4": "d"}
lst = [*d.values()]
print(lst)
Output:
["a", "b", "c", "d"]
Use the extend()
Function to Get the Values of a Dictionary in Python
The extend()
function can accept an iterable object and add all the elements from this object to the end of some list.
We can use this to get all values of a dictionary in a list. This function can accept the dict_values()
object and add the items to the end of some empty list.
The following code implements this logic.
d = {"1": "a", "2": "b", "3": "c", "4": "d"}
lst = []
lst.extend(d.values())
print(lst)
Output:
['a', 'b', 'c', 'd']
Now in this tutorial, we discussed three methods. Out of these three methods, the list()
function and the *
unpack operator are considered the most efficient in terms of speed. The unpack operator *
is the fastest one for small dictionaries, whereas the list()
function is considered more efficient for large dictionaries.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn