How to Fix ValueError: Classification Metrics Can't Handle a Mix of Multiclass and Continuous-Multioutput Targets
-
Use
1d-array
to FixValueError: Classification metrics can't handle a mix of multiclass and continuous-multioutput targets
in Python -
Fix the
ValueError: Classification metrics can't handle a mix of multiclass and continuous-multioutput targets
Error in Python
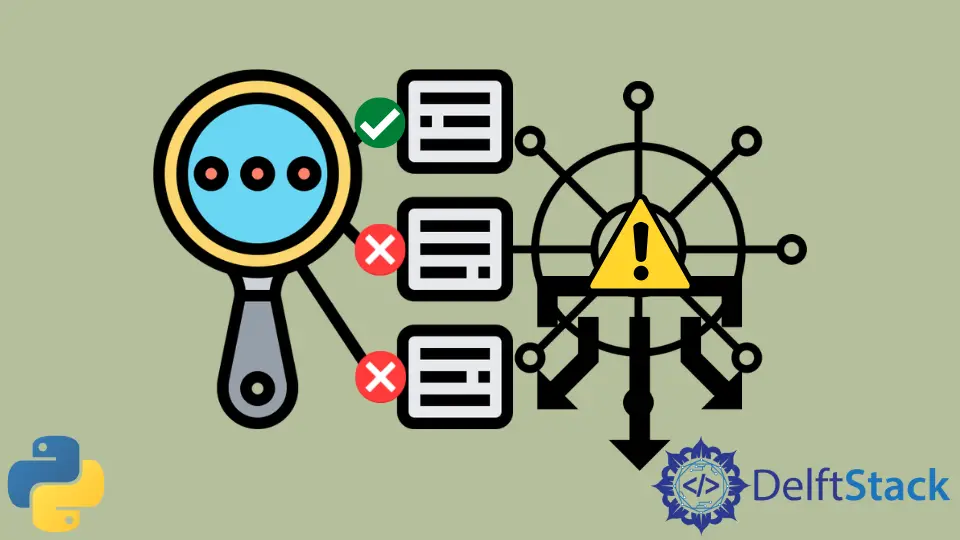
The ValueError
is raised in Python when you provide a valid argument to a function, but it is an invalid value. For instance, you will get the ValueError
when you input a negative number to the sqrt()
function of a math
module.
The error ValueError: Classification metrics can't handle a mix of multiclass and continuous-multioutput targets
occurs when you provide an invalid array in the sklearn.metrics.accuracy_score()
function. Since the accuracy score is a classification metric, the ValueError
can also be thrown when you use it with regression problems.
This tutorial will teach you to solve this error in Python.
Use 1d-array
to Fix ValueError: Classification metrics can't handle a mix of multiclass and continuous-multioutput targets
in Python
Firstly, we will recreate this error in Python.
from sklearn.metrics import accuracy_score
y_pred = [[0.5, 1], [-1, 1], [7, -6]]
y_true = [[0, 2], [-1, 2], [8, -5]]
accuracy_score(y_true, y_pred)
Output:
ValueError: Classification metrics can't handle a mix of multiclass and continuous-multioutput targets
The function accuracy_score()
does not support the multiclass-multioutput format. When the input given in the function is not 1d-array
, it displays the above error in the evaluation of the classification model.
You can solve it using the 1d-array
in the accuracy_score()
function.
Fix the ValueError: Classification metrics can't handle a mix of multiclass and continuous-multioutput targets
Error in Python
Another possible cause of the error might be that you are using the accuracy_score()
function for the regression problems. Accuracy score is not a measure of regression models; it is only for classification models.
The regression metrics are R2 Score, MSE (Mean Squared Error), and RMSE (Root Mean Squared Error), which can be used to evaluate the performance of a regression model.
from sklearn.metrics import r2_score
y_pred = [[0.5, 1], [-1, 1], [7, -6]]
y_true = [[0, 2], [-1, 2], [8, -5]]
print(r2_score(y_true, y_pred))
Output:
0.9412391668996365
Now you know how to handle ValueError: Classification metrics can't handle a mix of multiclass and continuous-multioutput targets
in Python. We hope you find these answers helpful.
Related Article - Python ValueError
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python