How to Fix ValueError Arrays Must All Be the Same Length in Python
- Understanding the ValueError
- Method 1: Trimming Arrays to the Same Length
- Method 2: Padding Arrays to the Same Length
- Method 3: Using Pandas for DataFrame Operations
- Conclusion
- FAQ
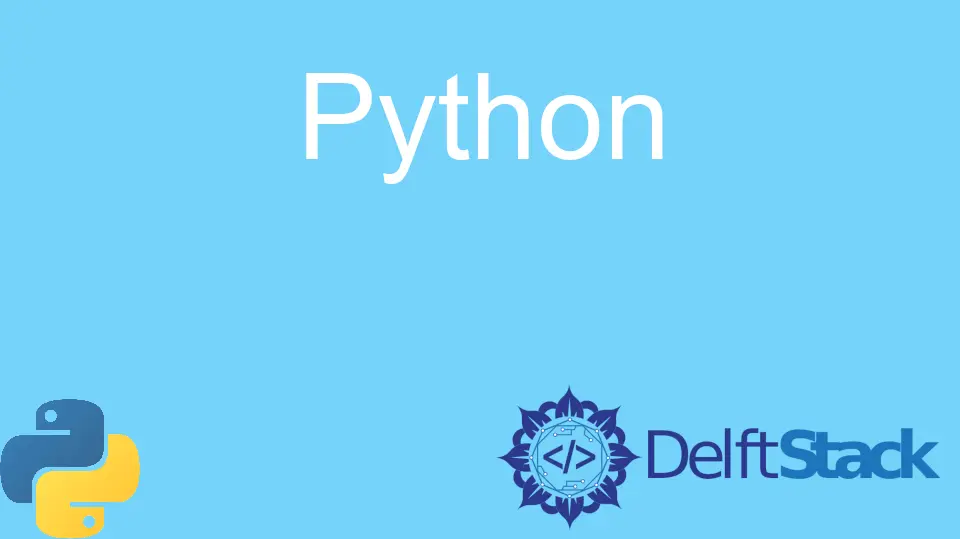
When working with data in Python, you may encounter the dreaded ValueError that states “arrays must all be the same length.” This error often arises when you’re trying to perform operations on lists, arrays, or data structures that require uniform lengths. Understanding how to troubleshoot and fix this issue can save you time and frustration.
In this article, we’ll explore effective methods to resolve this ValueError, complete with clear code examples. Whether you’re a beginner or an experienced programmer, you’ll find practical solutions to ensure your arrays align perfectly. Let’s dive into the intricacies of this common Python error and discover how to fix it.
Understanding the ValueError
Before we delve into solutions, it’s essential to understand why this error occurs. The ValueError typically surfaces when you’re attempting to combine or manipulate lists or arrays of different lengths. For example, if you’re trying to create a DataFrame from mismatched lists or perform operations that require element-wise calculations, Python will raise this error. This situation often arises in data analysis or machine learning tasks, where datasets may have missing or extra values.
To illustrate, consider the following code snippet:
import numpy as np
a = np.array([1, 2, 3])
b = np.array([4, 5])
result = a + b
The above code will throw a ValueError since a
and b
have different lengths.
Output:
ValueError: shapes (3,) and (2,) not aligned: 3 (dim 0) != 2 (dim 0)
This example highlights the need for uniform array lengths. Now, let’s explore methods to resolve this issue.
Method 1: Trimming Arrays to the Same Length
One of the simplest ways to fix the ValueError is to trim your arrays or lists so that they all have the same length. This method involves identifying the shortest array and slicing the longer arrays to match its length. Here’s how you can implement this:
import numpy as np
a = np.array([1, 2, 3, 4])
b = np.array([5, 6])
min_length = min(len(a), len(b))
a_trimmed = a[:min_length]
b_trimmed = b[:min_length]
result = a_trimmed + b_trimmed
In this code, we first determine the minimum length of the arrays using the min()
function. We then slice both arrays to that length, ensuring they are compatible for element-wise operations. Finally, we perform the addition.
Output:
array([6, 8])
By trimming the arrays, we eliminate the ValueError and successfully perform the operation. This method is straightforward and effective, particularly when dealing with datasets where the lengths can vary.
Method 2: Padding Arrays to the Same Length
Another approach to resolve the ValueError is to pad the shorter arrays with a specific value (such as zeros or NaNs) until they match the length of the longest array. This method is particularly useful when you want to retain all data points for further analysis. Here’s how you can do it:
import numpy as np
a = np.array([1, 2, 3])
b = np.array([4, 5, 6, 7])
max_length = max(len(a), len(b))
a_padded = np.pad(a, (0, max_length - len(a)), constant_values=0)
b_padded = np.pad(b, (0, max_length - len(b)), constant_values=0)
result = a_padded + b_padded
In this example, we use the np.pad()
function to add zeros to the end of the shorter array. The padding is done based on the difference in lengths, ensuring both arrays are now of equal length. After padding, we can perform the addition operation without encountering a ValueError.
Output:
array([5, 7, 9, 7])
Padding is a great way to handle mismatched lengths while preserving the integrity of your data. Just be mindful of the implications of adding padding, as it can affect the outcomes of your analysis.
Method 3: Using Pandas for DataFrame Operations
If you’re working with data in a tabular format, using the Pandas library can simplify the process of handling arrays of different lengths. Pandas automatically aligns data based on the index, which can help avoid the ValueError. Here’s an example of how to utilize Pandas to manage mismatched lengths:
import pandas as pd
data_a = {'values': [1, 2, 3]}
data_b = {'values': [4, 5, 6, 7]}
df_a = pd.DataFrame(data_a)
df_b = pd.DataFrame(data_b)
result = pd.concat([df_a, df_b], ignore_index=True)
In this code, we create two DataFrames from dictionaries. The pd.concat()
function allows us to concatenate the DataFrames while ignoring the index, effectively merging them into a single DataFrame without raising a ValueError.
Output:
values
0 1
1 2
2 3
3 4
4 5
5 6
6 7
Using Pandas not only resolves the ValueError but also provides a robust framework for data manipulation and analysis. This method is particularly advantageous when dealing with larger datasets or when performing complex operations.
Conclusion
Encountering the ValueError stating that arrays must all be the same length can be frustrating, but understanding how to resolve it is crucial for any Python programmer. Whether you choose to trim, pad, or utilize Pandas, each method provides a viable solution to ensure your arrays align correctly. By applying these techniques, you can enhance your data manipulation skills and avoid common pitfalls in your programming journey. Remember, the key is to maintain uniformity in your data structures to facilitate seamless operations. Happy coding!
FAQ
- what causes the ValueError “arrays must all be the same length” in Python?
This error occurs when you try to perform operations on arrays or lists of differing lengths, such as element-wise calculations or creating a DataFrame.
-
how can I avoid the ValueError when concatenating arrays?
You can avoid this error by ensuring that all arrays have the same length through trimming, padding, or using libraries like Pandas that handle length mismatches. -
is it better to trim or pad arrays to fix the ValueError?
It depends on your use case. Trimming is suitable when you can afford to lose data, while padding is better when you need to retain all data points. -
can I use Python built-in functions to fix array length issues?
Yes, built-in functions likemin()
,max()
, and slicing can help you adjust array lengths effectively. -
what libraries can help manage array length issues in Python?
Libraries like NumPy and Pandas are particularly useful for managing and manipulating arrays of varying lengths.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python