How to Use the try...else Block in Python
- Understanding the try Block
- Why Use the else Block?
- Combining try…except…else for Robust Error Handling
- Best Practices for Using try…else Blocks
- Conclusion
- FAQ
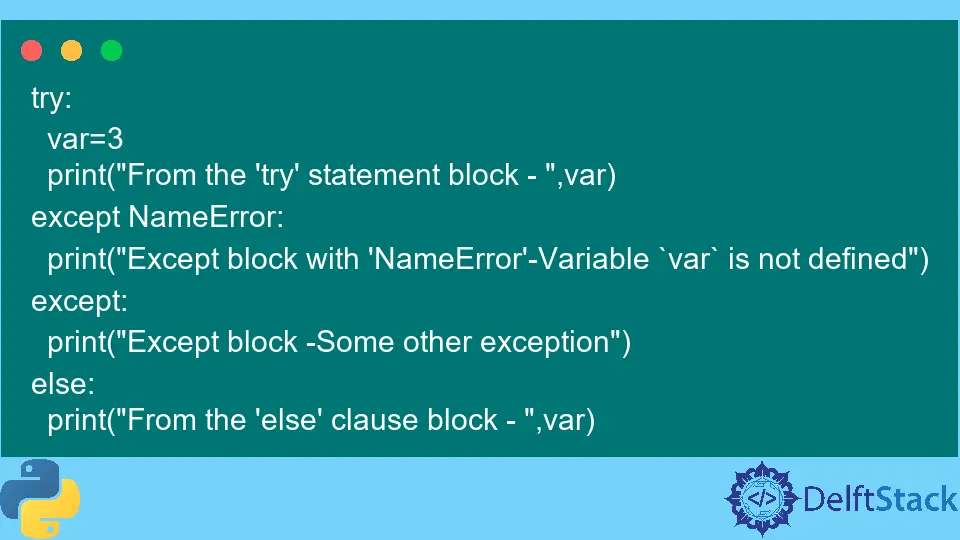
In Python, error handling is an essential skill for any programmer. One of the most powerful tools at your disposal is the try…else block. This construct allows you to handle exceptions gracefully while also executing code that should run only if no exceptions occur. The try block contains the code that might raise an exception, and if no exceptions are raised, the code in the else block will execute.
This article will delve into how to effectively use the try…else block in Python, providing practical examples and detailed explanations to help you master this feature.
Understanding the try Block
The try block is where you place the code that might throw an exception. When Python encounters an error in this block, it immediately stops executing the code and jumps to the corresponding except block, if one exists. If no errors occur, the program continues to the next block of code.
Here’s an example to illustrate this:
try:
number = int(input("Enter a number: "))
result = 10 / number
except ValueError:
print("That's not a valid number!")
except ZeroDivisionError:
print("You can't divide by zero!")
else:
print(f"The result is {result}")
In this example, the user is prompted to enter a number. If the input is not a number, a ValueError is raised, and the program will print an error message. If the input is zero, a ZeroDivisionError occurs. However, if the input is valid and not zero, the else block executes, displaying the result of the division.
Output:
Enter a number: 2
The result is 5.0
The try block allows you to handle various exceptions, ensuring your program doesn’t crash unexpectedly. The else block is particularly useful for executing code that should only run when the try block is successful, keeping your code clean and organized.
Why Use the else Block?
The else block serves a specific purpose in the try…except construct. It allows you to run code that should only execute if no exceptions were raised in the try block. This separation makes your code more readable and clear, as it clearly delineates the error-handling logic from the successful execution logic.
Consider the following example:
def divide_numbers(num1, num2):
try:
result = num1 / num2
except ZeroDivisionError:
print("You cannot divide by zero.")
else:
print(f"The result of {num1} divided by {num2} is {result}")
divide_numbers(10, 2)
divide_numbers(10, 0)
In this code, the divide_numbers function attempts to divide two numbers. If the second number is zero, it raises a ZeroDivisionError, and the except block handles this by printing an error message. If the division is successful, the else block executes, providing the result.
Output:
The result of 10 divided by 2 is 5.0
You cannot divide by zero.
Using the else block in this way helps clarify your intent to the reader. It indicates that the code within it should only run when the preceding try block completes without raising any exceptions. This structure not only enhances readability but also helps in debugging.
Combining try…except…else for Robust Error Handling
Combining the try, except, and else blocks provides a robust structure for error handling in Python. This combination allows you to capture exceptions, manage them gracefully, and execute code that should only run when no exceptions occur. This approach is especially useful in more complex applications where you may need to handle multiple types of exceptions.
Here’s an example that demonstrates this:
def read_file(file_name):
try:
with open(file_name, 'r') as file:
data = file.read()
except FileNotFoundError:
print(f"The file {file_name} does not exist.")
except IOError:
print("An error occurred while reading the file.")
else:
print("File read successfully:")
print(data)
read_file("example.txt")
In this example, the read_file function attempts to open and read a file. If the file does not exist, a FileNotFoundError is raised, which is caught by the except block. If there’s an I/O error, that is also handled. If the file is read successfully, the else block executes, confirming the successful operation.
Output:
The file example.txt does not exist.
This structure is beneficial because it allows you to clearly separate error-handling logic from successful execution logic. It also makes it easier to maintain and update your code in the future. By using try, except, and else together, you can create cleaner, more efficient Python programs.
Best Practices for Using try…else Blocks
When using the try…else block in Python, there are several best practices to keep in mind. First, only include code in the try block that is likely to raise an exception. This helps to keep the code clean and focused. Additionally, be specific in your exception handling. Catching generic exceptions can lead to unintended behavior and make debugging more challenging.
Another best practice is to use the else block to execute code that depends on the success of the try block. This not only improves readability but also helps to avoid executing code that should only run if no exceptions occurred.
Here’s a quick summary of best practices:
- Keep the try block focused on code that may raise exceptions.
- Handle specific exceptions rather than using a general exception.
- Use the else block for code that should only run when the try block is successful.
- Maintain clear and concise error messages to aid in debugging.
By following these best practices, you can enhance the reliability and maintainability of your Python code.
Conclusion
The try…else block in Python is a powerful feature that allows for effective error handling and clean code structure. By understanding how to use it effectively, you can create programs that are not only robust but also easier to read and maintain. Whether you’re handling user input or managing file operations, the try…else construct can help you manage exceptions gracefully. As you continue to develop your Python skills, mastering this feature will undoubtedly enhance your programming repertoire.
FAQ
-
What is the purpose of the try block in Python?
The try block is used to enclose code that might raise an exception, allowing you to handle errors gracefully. -
When should I use the else block?
The else block should be used for code that should only execute if the try block completes without raising any exceptions. -
Can I have multiple except blocks?
Yes, you can have multiple except blocks to handle different types of exceptions separately. -
Is it necessary to use an else block with try?
No, using an else block is optional. You can use try and except blocks without an else. -
How does the try…except…else structure improve code readability?
This structure separates error handling from successful execution logic, making the code clearer and easier to follow.