How to Use of Memory Caching in Python
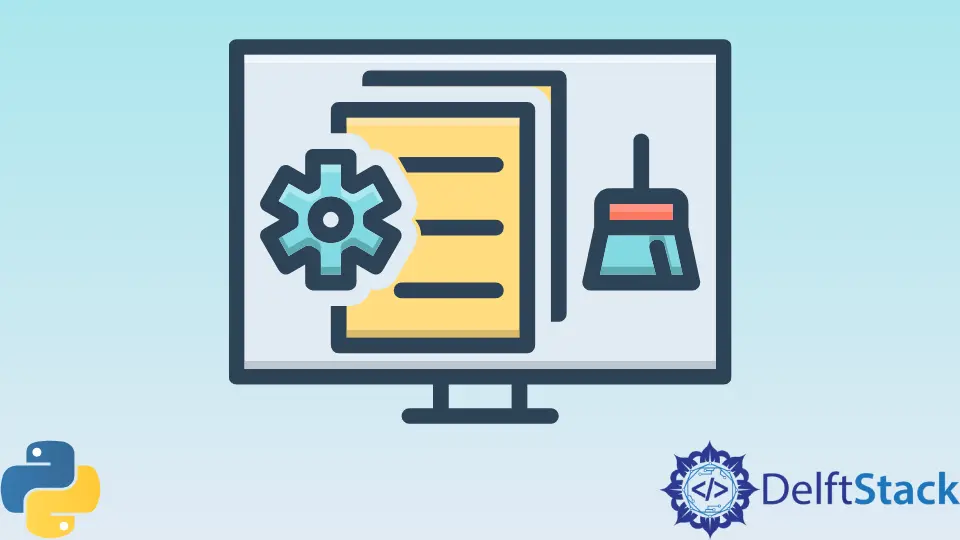
When writing Python applications, caching is important. Using a cache to avoid recomposing data or accessing a slow database can boost your performance.
In Python, we can use the memcached
module to include memory caching in our scripts. This article will discuss preparing memory caching operations and the primary memcached
usage.
We will also learn about advanced patterns using Python cache
and set
.
Install Memcached
Module
The Memcached
package is available for many platforms:
- For Linux, we can install it using
yum install memcached
orapt-get install memcached
. This script will install thememcached
package from a pre-built package. - For macOS, the easiest choice is to utilize Homebrew. After installing the Homebrew package manager, type
brew install memcached
. - For Windows, you would have to compile
memcached
yourself by going to the officialmemcached
website.
Once installed, memcached
can be launched by calling the memcached
command:
memcached
Set and Get Cached Values Using Python
The memcached
package is straightforward to grasp if you have never used it. In addition, it gives access to a sizable vocabulary over the network.
This dictionary differs from a traditional Python dictionary in a few ways, mainly:
- Values and keys have to be in bytes data type
- Values and keys are automatically deleted after a given expiration time
Therefore, set
and get
are the two fundamental procedures for dealing with memcached
. They are employed to give a key a value or to get a value from a key, as we would have imagined.
The code below demonstrates how to utilize memcached
as a network-distributed cache in your Python applications:
import memcache
mcobject = memcache.Client(["127.0.0.1:11212"], debug=0)
mcobject.set("some_key", "Some value")
value = mc.get("some_key")
mcobject.set("another_key", 3)
mcobject.delete("another_key")
mcobject.set("key", "1")
mcobject.incr("key")
mcobject.decr("key")
The memcached
network protocol is straightforward. Due to its lightning-fast implementation, storing data that would otherwise take a long time to compute or get from the canonical source of data is advantageous.
While straightforward, this example allows storing key-value
tuples across the network and accessing them through multiple, distributed, running copies of your application.
This process is simplistic yet powerful. And it’s a significant first step toward optimizing your application.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn