How to Fix the Pylint Unresolved Import Error in Python
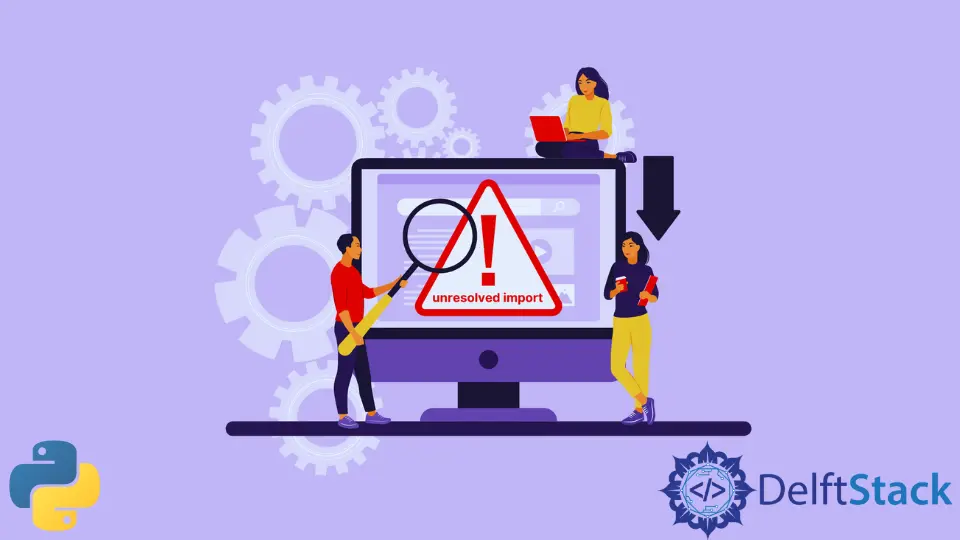
If we have just started coding Python in Visual Studio Code, ensure the Python environment has been set. If we have not set the Python environment, Visual Studio Code couldn’t detect the specific Python executor.
This article will discuss solving the unresolved import
error with Pylint in Python.
Fix the Pylint unresolved import
Error in Python
To solve the unresolved import
error in Python, set your Python path in your workspace settings. For example, if we work with Visual Studio Code and import any library, we will face this error: unresolved import
.
{
"python.pythonPath": "/path/to/your/venv/bin/python",
}
Then reload the Visual Studio Code to fix the error.
It resolves the unresolved import
issue for the Python-specific imports, but it won’t fix your modules. Instead, when importing your modules, it will return the same error, and to fix this error, use the following setting in your workspace: .vscode/settings.json
.
"python.autoComplete.extraPaths": ["./path-to-your-code"],
The workspace settings file is in your root folder under the .vscode
folder.
The imports are left unresolved when you import your Python modules from the workspace folder, but the main script is not in the workspace folder’s root directory.
We can also use the command interface in another manner.
Enter Cmd
or Ctrl+Shift+P followed by "Python: Select Interpreter"
and then choose the one with the packages we look for. To know more about the Visual Studio Code workspace settings, you may refer to the official documentation that can be found in this link.
Use the .env
File
We can also create a .env
file in your project root folder. Then add a PYTHONPATH
to it like the following code.
pythonPATH = path / to / your / code
And in your settings.json
file, add the following code.
"python.envFile": ".env"
Then reload Visual Studio Code to fix the error.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python