How to Unindent Code in Python
- Using Text Editors to Unindent Code
- Using the Python Command Line
- Using Online Code Editors
- Using Python IDE Features
- Conclusion
- FAQ
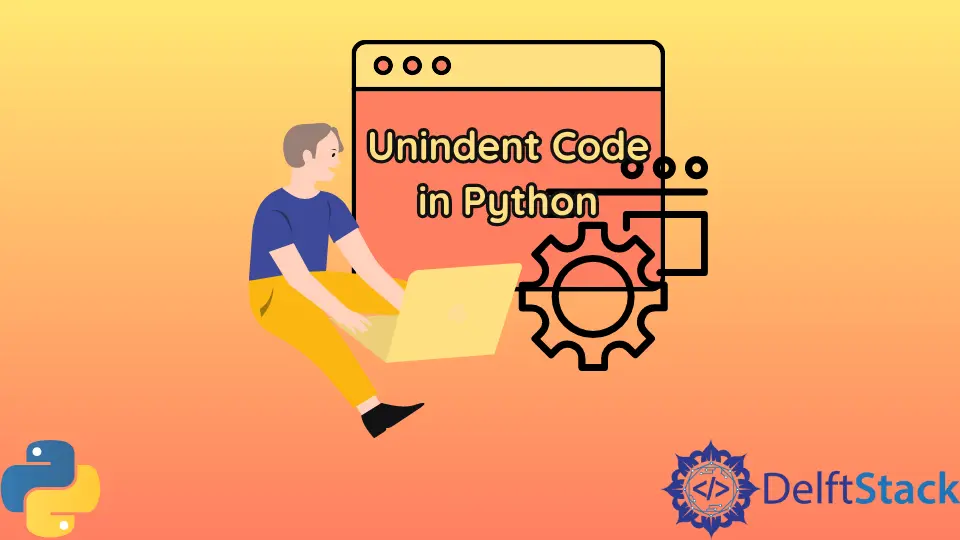
When working with Python, maintaining proper indentation is crucial. Indentation not only improves readability but also defines the structure of your code. However, there may be times when you need to unindent code, whether to correct formatting issues or to refactor your code.
This tutorial will walk you through various methods to unindent code in Python effectively. By the end, you’ll have a solid understanding of how to adjust indentation and enhance your coding practices. Let’s dive in!
Using Text Editors to Unindent Code
One of the simplest methods to unindent code in Python is by using a text editor or an Integrated Development Environment (IDE) that supports Python. Popular editors like Visual Studio Code, PyCharm, and Sublime Text offer built-in features to manage indentation easily.
Here’s how to unindent code using Visual Studio Code:
- Open your Python file in Visual Studio Code.
- Select the lines of code you want to unindent.
- Press
Shift + Tab
to decrease the indentation level.
Here’s an example of how this works:
def greet():
print("Hello, World!")
print("Welcome to Python programming.")
If you unindent the second line, it will look like this:
def greet():
print("Hello, World!")
print("Welcome to Python programming.")
Output:
Hello, World!
Welcome to Python programming.
Using a text editor to unindent code is efficient and user-friendly. Most editors allow you to customize indentation settings, such as switching between spaces and tabs. This flexibility can help you maintain a consistent style across your codebase. Additionally, many editors provide features like auto-formatting, which can automatically adjust indentation based on Python’s syntax rules, saving you time and effort.
Using the Python Command Line
If you prefer working directly in the command line, Python’s interactive shell can also help you unindent code. This method is particularly useful for quick adjustments or for testing snippets of code without having to use a full-fledged IDE.
Here’s how to do it:
- Open your terminal or command prompt.
- Start the Python interactive shell by typing
python
orpython3
. - Copy and paste your code into the shell.
- To unindent a specific line, you can simply type it without the leading spaces.
For instance, consider the following code:
def add(a, b):
result = a + b
return result
If you want to unindent the result
line, you can do this:
def add(a, b):
result = a + b
return result
Output:
SyntaxError: unexpected indent
This method is straightforward, but it’s important to remember that Python relies heavily on indentation for defining blocks of code. Unindenting a line incorrectly can lead to syntax errors, so always double-check your indentation levels before running your code.
Using Online Code Editors
For those who prefer a web-based solution, online code editors can be a great way to unindent Python code. Websites like Repl.it or Jupyter Notebook allow you to write and test your code without needing to install anything locally. These platforms often come with built-in features to adjust indentation easily.
Here’s how you can unindent code using an online editor:
- Go to an online code editor like Repl.it.
- Create a new Python file.
- Paste your code into the editor.
- Select the lines you want to unindent and hit the
Tab
key orShift + Tab
to adjust the indentation.
Consider this example in an online editor:
def calculate_area(radius):
area = 3.14 * radius * radius
return area
If you decide to unindent the area
line, it will look like this:
def calculate_area(radius):
area = 3.14 * radius * radius
return area
Output:
SyntaxError: unexpected indent
Online editors often provide additional features like collaboration tools, which can be particularly useful for team projects. They also help you test your code in real-time, making it easier to visualize how indentation affects execution.
Using Python IDE Features
Integrated Development Environments (IDEs) like PyCharm or Eclipse come equipped with features specifically designed to manage code formatting, including indentation. These tools often provide shortcuts and settings that make unindenting code a breeze.
Here’s how to unindent code in PyCharm:
- Open your Python file in PyCharm.
- Select the lines you want to unindent.
- Press
Shift + Tab
to decrease the indentation level.
Let’s say you have the following code:
for i in range(5):
print(i)
print("This is number", i)
If you want to unindent the second print
statement, you can do it like this:
for i in range(5):
print(i)
print("This is number", i)
Output:
0
1
2
3
4
This is number 4
Using an IDE not only simplifies the process of unindenting code but also enhances your overall coding experience. Features like code suggestions, error highlighting, and version control integration can significantly improve your productivity.
Conclusion
Unindenting code in Python is an essential skill that can help you maintain clean and readable code. Whether you choose to use a text editor, the command line, an online editor, or an IDE, each method has its own advantages. By mastering these techniques, you’ll be better equipped to handle Python’s indentation rules and write more efficient code. Remember, proper indentation is not just about aesthetics; it’s a fundamental aspect of Python programming that can affect the functionality of your code.
FAQ
-
How does indentation affect Python code?
Indentation in Python defines the blocks of code, such as loops and functions. Incorrect indentation can lead to syntax errors or unexpected behavior. -
Can I use tabs and spaces interchangeably for indentation?
No, it’s best to stick to either tabs or spaces for indentation to avoid inconsistencies. PEP 8 recommends using 4 spaces per indentation level. -
What should I do if my code throws an indentation error?
Check your code for inconsistent use of tabs and spaces. Ensure that all lines in a block are indented the same way. -
Are there any tools to help with code formatting?
Yes, tools like Black and autopep8 can automatically format your code, including fixing indentation issues.
- Is there a way to visualize indentation levels in my code?
Some IDEs and text editors have features that highlight indentation levels, making it easier to spot inconsistencies.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn