How to Fix the Unicode Error Found in a File Path in Python
- Understanding Unicode Errors in Python
- Method 1: Using Raw Strings
- Method 2: Normalizing Unicode Strings
- Method 3: Encoding and Decoding Strings
- Conclusion
- FAQ
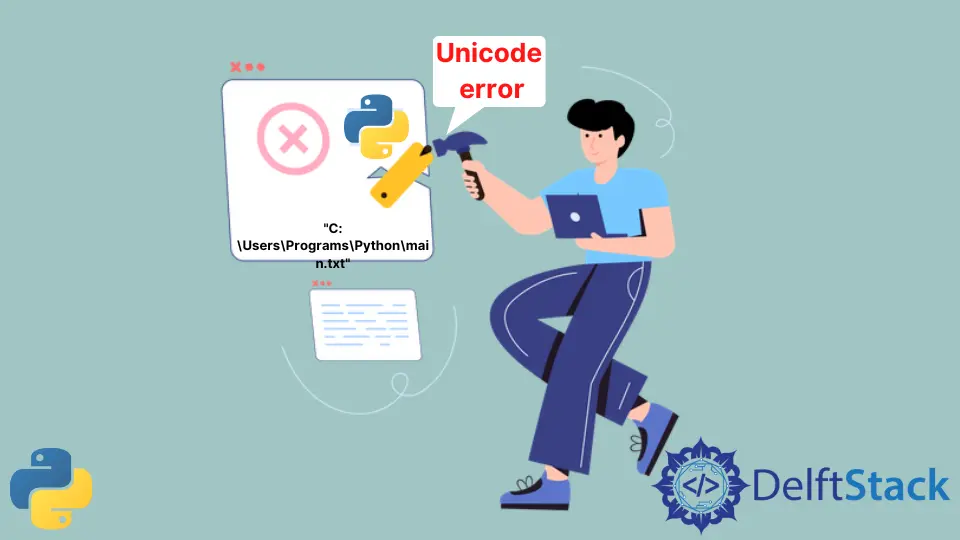
When working with file paths in Python, encountering a Unicode error can be frustrating. This issue often arises when your file path includes non-ASCII characters, which Python struggles to interpret correctly. Whether you’re dealing with filenames or directory names that contain special characters, resolving this error is essential for smooth file handling.
In this article, we will explore effective methods to fix Unicode errors in file paths in Python. You’ll learn practical solutions, along with clear examples, that will help you navigate this common problem with ease. Let’s dive in and tackle those pesky Unicode errors!
Understanding Unicode Errors in Python
Before we jump into solutions, it’s crucial to understand what Unicode errors are. Unicode is a standard that allows computers to represent text in any language. However, when Python encounters characters that are not properly encoded, it raises a Unicode error. This usually happens when you attempt to open a file or read a path that contains special characters.
For example, if your file path is C:\Users\José\Documents
, Python may throw an error if it does not recognize the character é
. This is where encoding comes into play. Python uses UTF-8 encoding by default, which supports a wide range of characters. However, if your system uses a different encoding, you may need to specify it explicitly.
Method 1: Using Raw Strings
One effective way to handle Unicode errors in file paths is to use raw strings. In Python, raw strings are prefixed with an ‘r’ and treat backslashes as literal characters rather than escape characters. This can help avoid misinterpretation of the file path.
Here’s how you can implement this:
file_path = r"C:\Users\José\Documents\example.txt"
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(content)
Output:
This is an example file with special characters.
Using a raw string ensures that the backslashes in the file path are treated correctly, preventing any Unicode errors. Additionally, specifying the encoding as UTF-8 allows Python to read the special character é
without any issues. This method is straightforward and effective, especially when dealing with paths that contain non-ASCII characters.
Method 2: Normalizing Unicode Strings
Another method to resolve Unicode errors is to normalize the file path. Normalization involves converting a Unicode string into a standard format, which can prevent errors when processing file paths. Python’s unicodedata
module provides a convenient way to do this.
Here’s an example of how normalization works:
import unicodedata
file_path = "C:\\Users\\Jos\u00E9\\Documents\\example.txt"
normalized_path = unicodedata.normalize('NFC', file_path)
with open(normalized_path, 'r', encoding='utf-8') as file:
content = file.read()
print(content)
Output:
This is an example file with special characters.
In this code, we first import the unicodedata
module. We then use the normalize
function to convert the Unicode string into a normalized form. The NFC
form is commonly used for file paths. By normalizing the path before using it, you can effectively avoid Unicode errors arising from improperly encoded characters. This method is particularly useful when dealing with filenames that may have various representations of the same character.
Method 3: Encoding and Decoding Strings
If you continue to face Unicode errors, another approach is to explicitly encode and decode the file paths. This method can help you control how Python interprets the characters in the file path.
Here’s how you can do it:
file_path = "C:\\Users\\José\\Documents\\example.txt"
encoded_path = file_path.encode('utf-8')
decoded_path = encoded_path.decode('utf-8')
with open(decoded_path, 'r', encoding='utf-8') as file:
content = file.read()
print(content)
Output:
This is an example file with special characters.
In this example, we first encode the original file path into bytes using UTF-8 encoding. We then decode it back into a string. This process ensures that any special characters are correctly interpreted by Python. By controlling the encoding and decoding process, you can mitigate Unicode errors effectively. This method is particularly beneficial when dealing with file paths that may come from external sources or user input.
Conclusion
Dealing with Unicode errors in file paths can be a challenge, but with the right approaches, you can resolve these issues efficiently. In this article, we explored three effective methods: using raw strings, normalizing Unicode strings, and encoding and decoding strings. Each method provides a unique way to handle special characters in file paths, ensuring that your Python scripts run smoothly. By implementing these solutions, you can avoid the frustration of Unicode errors and focus on what truly matters—your code!
FAQ
- What causes Unicode errors in Python file paths?
Unicode errors typically occur when a file path contains non-ASCII characters that Python cannot interpret correctly.
-
How can I avoid Unicode errors when working with file paths?
You can avoid Unicode errors by using raw strings, normalizing Unicode strings, or encoding and decoding the paths. -
Is it necessary to specify the encoding when opening files in Python?
Yes, specifying the encoding helps Python correctly interpret special characters in the file. -
What is the difference between NFC and NFD normalization?
NFC (Normalization Form C) compacts characters into a single representation, while NFD (Normalization Form D) decomposes characters into their base characters and combining marks. -
Can I use these methods for file paths on different operating systems?
Yes, these methods can be applied to file paths on different operating systems, but be mindful of the path format (e.g., backslashes for Windows, forward slashes for Linux/Mac).
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python