How to Fix the Unhashable Type numpy.ndarray Error in Python
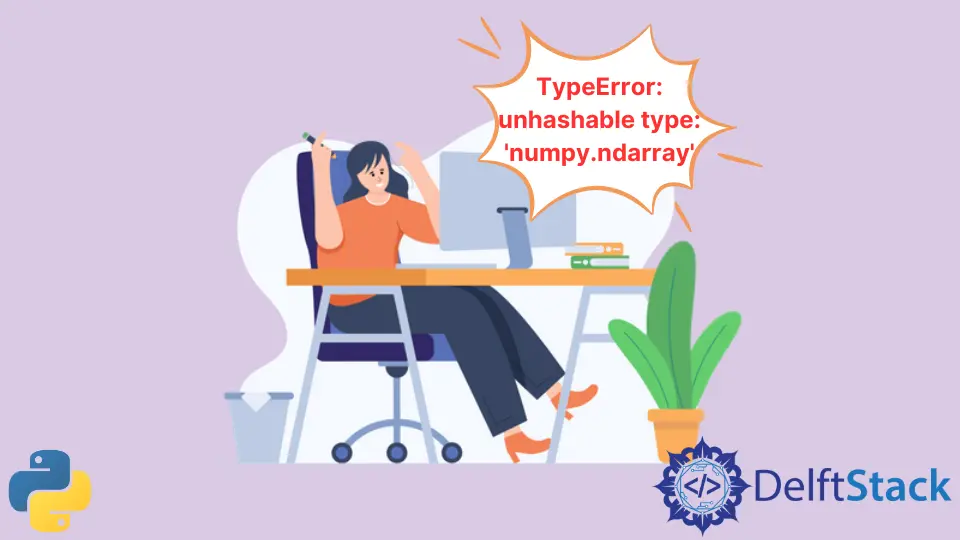
Python dictionary is a robust and scalable data structure that stores data in the form of key-value pairs. In key-value pairs, unique key points to some value, and there are no limitations to what the value is. A value can be an integer, a floating-point number, a boolean, a list of numbers, a class object, a list of class objects, a tuple, a dictionary, and many more. Whereas there are some limitations to what a key can be.
The essential condition for a key is that it should be a hashable object. A hashable object refers to an object which once defined, can not be further modified during its lifetime or immutable and can be represented with a unique hash value. A hash value is a unique integer value.
Data types such as int
, float
, str
, tuple
, and class objects are immutable objects. It means that they can be safely used as keys in dictionaries. Problems arise when we are not particular about the data type of keys. For example, if we try to use a list
or a numpy.ndarray
as a key, we will run into TypeError: unhashable type: 'list'
and TypeError: unhashable type: 'numpy.ndarray'
errors respectively.
In this article, we will learn how to avoid this error for NumPy arrays.
Fix the unhashable type numpy.ndarray
Error in Python
We have to convert a NumPy array to a data type that can be safely used as a key to fixing this error. And, in the case of arrays and lists, a tuple is the way to go. Refer to the following Python code for the same.
import numpy as np
dictionary = {}
n = np.array([1.234, 21.33, 3.413, 4.4, 15.0000])
n = tuple(n) # Conversion
dictionary[n] = "Hello World"
print(dictionary)
Output:
{(1.234, 21.33, 3.413, 4.4, 15.0): 'Hello World'}
Python’s in-built tuple()
method will perform the necessary conversion for the iterable object.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python