How to Fix TypeError: Non-Empty Format String Passed to Object.__format__ in Python
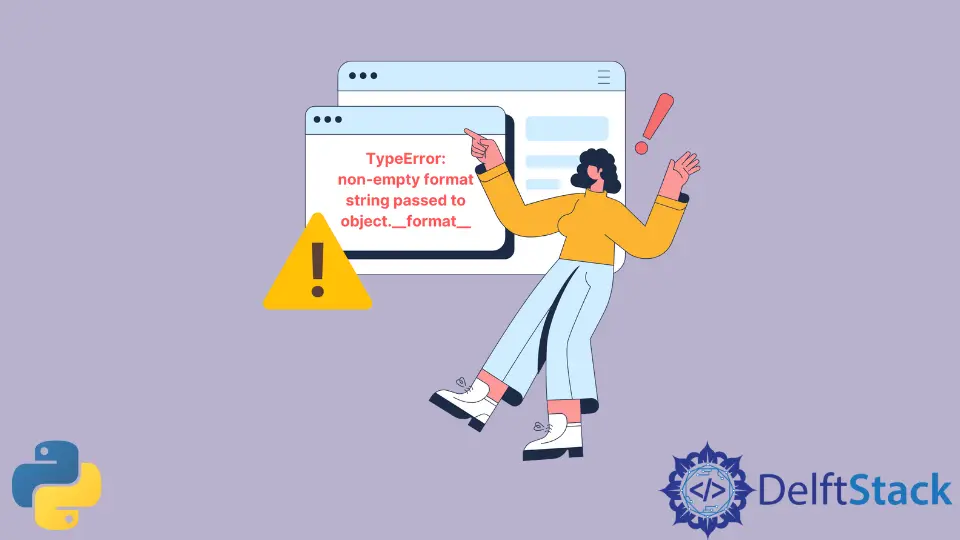
The format()
method in python lets you substitute variables and performs data formatting. This method is not designed to deal with any inputs other than the following:
- String denoted as
s
- Decimal denoted as
d
- Float denoted as
f
- Character denoted as
c
- Octal denoted as
o
- Hexadecimal denoted as
x
- Binary denoted as
b
- Exponential is denoted as
e
If any other data type accesses the method, the interpreter raises the below error:
TypeError: non-empty format string passed to object.__format__
Causes and Solutions to TypeError: Non-Empty Format String Passed to Object.__format__
in Python
Suppose we try to invoke the format()
method on a data type that does not have this method, for example, the byte
data type. The interpreter will raise an error because the byte
type object has no format()
method.
In the following code, we have intentionally invoked the format()
method with the byte
data type.
Example Code:
# Python 3.x
"{:10}".format(b"delftstack")
Output:
#Python 3.x
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-12-1909c614b7f5> in <module>()
----> 1 '{:10}'.format(b'delftstack')
TypeError: unsupported format string passed to bytes.__format__
The solution to this error is explicitly converting the data type from byte to string. We will use the !s
symbol for conversion.
Example Code:
# Python 3.x
s = "{!s:10s}".format(b"delftstack")
print(s)
Output:
#Python 3.x
b'delftstack'
The TypeError: non-empty format string passed to object.__format__
is also raised when we try to format None
.
Example Code:
# Python 3.x
"{:.0f}".format(None)
Output:
#Python 3.x
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-4-89103a1332e2> in <module>()
----> 1 '{:.0f}'.format(None)
TypeError: unsupported format string passed to NoneType.__format__
The solution is to pass a valid data type instead of None
.
Example Code:
# Python 3.x
s = "{!s:10s}".format(b"delftstack")
print(s)
Output:
#Python 3.x
b'delftstack'
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python