How to Fix TypeError: 'map' Object Is Not Subscriptable in Python
- Understanding the TypeError: ‘map’ Object Is Not Subscriptable
- Convert the Map Object to a List
- Iterate Over the Map Object
- Use List Comprehensions
- Conclusion
- FAQ
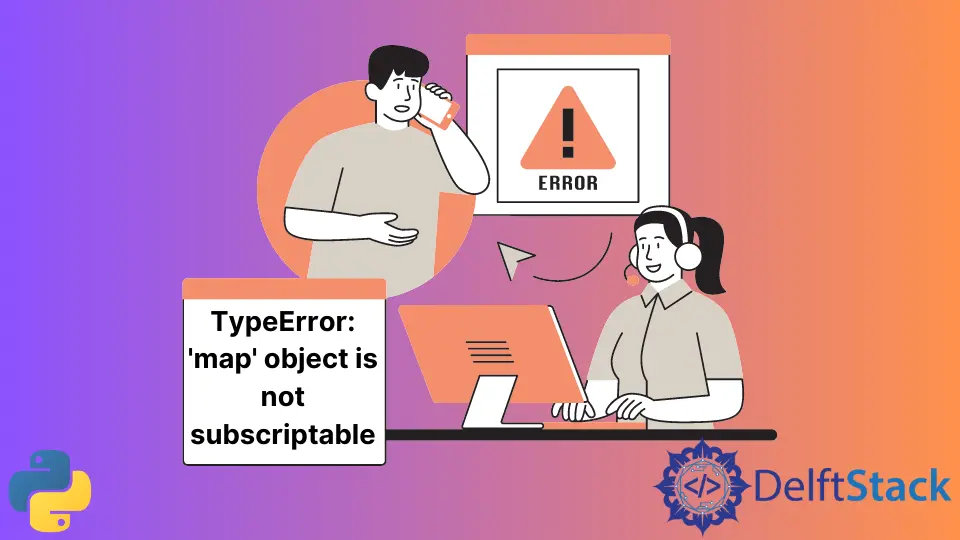
When working with Python, encountering errors is a common part of the development process. One such error that many developers face is the TypeError: ‘map’ object is not subscriptable. This error typically arises when you attempt to access elements of a map object using indexing, which is not allowed.
In this tutorial, we will delve into the reasons behind this error and provide practical solutions to resolve it. Whether you’re a beginner or an experienced programmer, understanding how to handle this error will enhance your coding skills and improve your Python projects. Let’s explore the details and get you back on track with your code!
Understanding the TypeError: ‘map’ Object Is Not Subscriptable
Before diving into the solutions, it’s essential to understand what a map object is and why it’s not subscriptable. In Python, the map()
function applies a given function to all items in an iterable (like a list) and returns a map object, which is an iterator. Unlike lists or tuples, map objects do not support indexing, which leads to the TypeError when you try to access elements using square brackets.
For example, if you run the following code:
numbers = [1, 2, 3, 4]
squared = map(lambda x: x ** 2, numbers)
print(squared[0])
You will encounter the TypeError because squared
is a map object, and you cannot access its elements using indexing.
Output:
TypeError: 'map' object is not subscriptable
Now that we have a clear understanding of the error, let’s explore some effective methods to fix it.
Convert the Map Object to a List
One of the simplest ways to resolve the TypeError: ‘map’ object is not subscriptable is to convert the map object into a list. By doing this, you can utilize indexing to access the elements as you would with any other list.
Here’s how you can do it:
numbers = [1, 2, 3, 4]
squared = map(lambda x: x ** 2, numbers)
squared_list = list(squared)
print(squared_list[0])
Output:
1
In this example, we first create a list of numbers and then use the map()
function to square each number. By converting the squared
map object into a list using the list()
function, we can now access its elements via indexing. This method is straightforward and effective, especially when you need to work with the results of the map function multiple times.
Iterate Over the Map Object
If you don’t need to access specific elements by index, another approach is to iterate over the map object directly. This method is particularly useful when you want to perform operations on each item without the need for indexing.
Here’s an example:
numbers = [1, 2, 3, 4]
squared = map(lambda x: x ** 2, numbers)
for value in squared:
print(value)
Output:
1
4
9
16
In this case, we use a for
loop to iterate through the squared
map object. Each squared value is printed directly without the need for indexing. This method is efficient and aligns well with Python’s design philosophy of working with iterators, making your code cleaner and more readable.
Use List Comprehensions
A more Pythonic way to achieve the same result is by using list comprehensions instead of the map()
function. List comprehensions are not only concise but also more readable for many developers. They allow you to create a new list by applying an expression to each item in an iterable.
Here’s how you can implement this:
numbers = [1, 2, 3, 4]
squared = [x ** 2 for x in numbers]
print(squared[0])
Output:
1
In this example, we create a new list called squared
using a list comprehension. Each number in the numbers
list is squared and stored in the new list. This method not only eliminates the TypeError but also enhances code readability and performance. You can easily access elements using indexing, making it a versatile solution.
Conclusion
The TypeError: ‘map’ object is not subscriptable can be a frustrating hurdle for Python developers, especially when working with functional programming concepts. However, by understanding the nature of map objects and implementing the solutions we’ve discussed—converting to a list, iterating directly, or using list comprehensions—you can effectively overcome this error. With these strategies, you can enhance your coding practices and create more efficient Python scripts. Remember, the key is to choose the method that best suits your specific needs and coding style.
FAQ
-
What is a map object in Python?
A map object is an iterator that applies a given function to all items in an iterable, returning the results as a map object. -
Why can’t I index a map object?
Map objects are iterators and do not support indexing, which is why you encounter the TypeError when trying to access elements using square brackets. -
How can I convert a map object to a list?
You can convert a map object to a list by using thelist()
function, which allows you to access elements using indexing. -
What is the benefit of using list comprehensions?
List comprehensions provide a more concise and readable way to create lists by applying an expression to each item in an iterable, eliminating the need for themap()
function. -
Can I convert a map object to other data types?
Yes, you can convert a map object to other data types like sets or tuples using theset()
ortuple()
functions, respectively.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python