How to Fix the Iteration Over a 0-D Array Error in Python NumPy
- Understanding the 0-D Array Error
- Solution 1: Use the Item Method to Access Scalar Values
- Solution 2: Reshape the Array to a 1-D Array
- Solution 3: Check the Array Dimensionality Before Iteration
- Conclusion
- FAQ
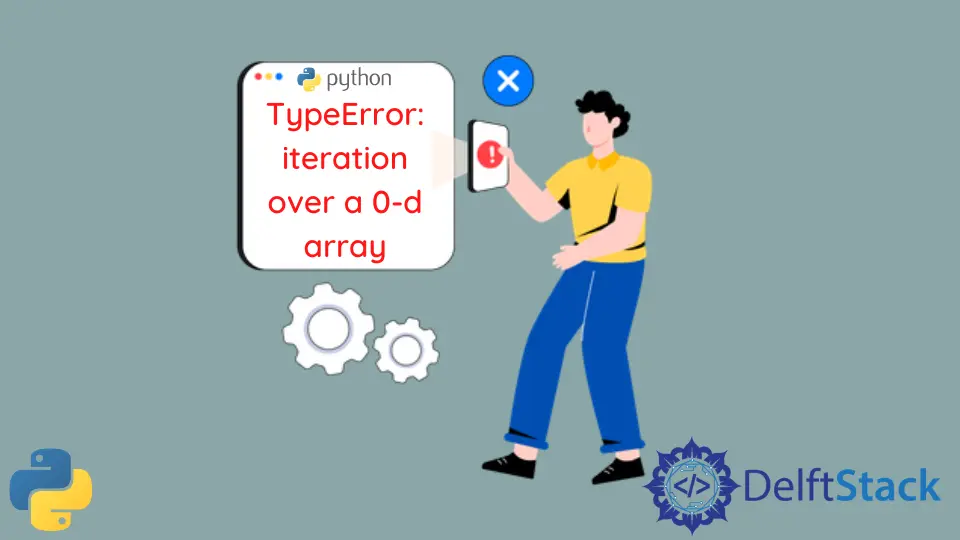
When working with Python’s NumPy library, you may encounter a frustrating error: “iteration over a 0-D array.” This error usually arises when you attempt to iterate over a scalar value represented as a 0-dimensional array. Understanding this issue is crucial for effective debugging and ensuring your code runs smoothly.
In this article, we’ll explore the causes of this error and provide practical solutions to fix it. Whether you’re a beginner or an experienced Python developer, you’ll find valuable insights and code examples to help you navigate this common pitfall. Let’s dive into the world of NumPy and learn how to tackle the iteration over a 0-D array error head-on.
Understanding the 0-D Array Error
Before we jump into the solutions, it’s essential to understand what a 0-D array is. In NumPy, arrays can have multiple dimensions, but a 0-D array is essentially a single value. When you try to iterate over such an array, Python raises an error because it’s not iterable. For instance, if you create a NumPy array with a single element, it becomes a 0-D array. Here’s a simple example:
import numpy as np
array_0d = np.array(42)
for item in array_0d:
print(item)
Output:
TypeError: 'numpy.int64' object is not iterable
This error occurs because array_0d
is a 0-D array containing a scalar value, and Python cannot iterate over it. Now that we understand the problem let’s explore some solutions.
Solution 1: Use the Item Method to Access Scalar Values
One of the simplest ways to fix the “iteration over a 0-D array” error is to use the item()
method. This method retrieves the scalar value from a 0-D array, allowing you to work with it directly without attempting to iterate. Here’s how you can implement this solution:
import numpy as np
array_0d = np.array(42)
value = array_0d.item()
print(value)
Output:
42
By calling array_0d.item()
, we extract the scalar value 42
from the 0-D array. This approach eliminates the need for iteration, making it a straightforward solution to the error. Using item()
is particularly useful when you’re dealing with single values in your computations, as it simplifies your code and avoids unnecessary complexity.
Solution 2: Reshape the Array to a 1-D Array
Another effective method to resolve the iteration over a 0-D array error is to reshape the array into a 1-D array. Reshaping allows you to convert a scalar into an array with one dimension, thus making it iterable. Here’s how to do it:
import numpy as np
array_0d = np.array(42)
array_1d = array_0d.reshape(1)
for item in array_1d:
print(item)
Output:
42
In this example, we use the reshape(1)
method to convert the 0-D array into a 1-D array. Now, array_1d
contains a single element but is iterable. This method is particularly useful if you anticipate needing to iterate over similar values in the future. Reshaping can also help maintain consistency in your code, especially when working with functions that expect arrays as input.
Solution 3: Check the Array Dimensionality Before Iteration
A more proactive approach to avoid the iteration over a 0-D array error is to check the dimensionality of the array before attempting to iterate. By using the ndim
attribute of a NumPy array, you can determine how many dimensions the array has and handle it accordingly. Here’s how to implement this solution:
import numpy as np
array_0d = np.array(42)
if array_0d.ndim == 0:
print(array_0d.item())
else:
for item in array_0d:
print(item)
Output:
42
In this code, we first check if array_0d
has 0 dimensions. If it does, we directly print the scalar value using item()
. Otherwise, we proceed with the iteration. This method is an excellent way to make your code more robust, as it prevents runtime errors and ensures that your program behaves as expected regardless of the input.
Conclusion
Encountering the “iteration over a 0-D array” error in Python NumPy can be a stumbling block for many developers. However, with the right understanding and techniques, you can easily overcome this challenge. By using methods like item()
, reshaping arrays, or checking the array’s dimensionality, you can effectively handle 0-D arrays and ensure your code runs smoothly. Remember, the key is to recognize when you’re dealing with scalar values and to adapt your approach accordingly. With these solutions in your toolkit, you can confidently navigate the world of NumPy without fear of encountering this common error.
FAQ
-
what is a 0-D array in NumPy?
A 0-D array in NumPy is essentially a scalar value represented as an array with zero dimensions. -
how can I check the dimensionality of a NumPy array?
You can check the dimensionality of a NumPy array using thendim
attribute. -
what does the
item()
method do in NumPy?
Theitem()
method retrieves the scalar value from a 0-D array, allowing you to work with it directly. -
can I iterate over a 1-D array in NumPy?
Yes, you can iterate over a 1-D array in NumPy, as it is iterable. -
why does the error occur only with 0-D arrays?
The error occurs because 0-D arrays do not contain multiple values, making them non-iterable.
Related Article - Python Array
- How to Initiate 2-D Array in Python
- How to Count the Occurrences of an Item in a One-Dimensional Array in Python
- How to Downsample Python Array
- How to Sort 2D Array in Python
- How to Create a BitArray in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python