How to Solve the TypeError: An Integer Is Required in Python
- Understanding the TypeError: An Integer Is Required
- Method 1: Type Casting
- Method 2: Input Validation
- Method 3: Using Try-Except Blocks
- Conclusion
- FAQ
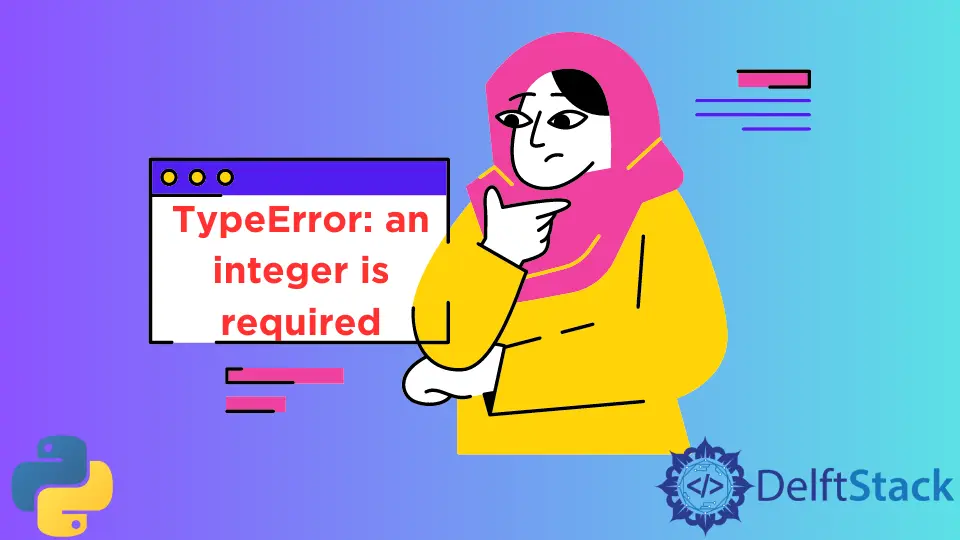
When working with Python, encountering errors is a common part of the development process. One such error that can be particularly frustrating is the TypeError: an integer is required. This error typically occurs when a piece of code expects an integer input but receives a different data type instead. Whether you’re a seasoned developer or just starting out, understanding how to diagnose and fix this error is crucial for smooth coding.
In this tutorial, we will dive into the causes of this error and provide you with practical solutions to resolve it. By the end of this guide, you’ll be equipped with the knowledge to tackle this TypeError confidently.
Understanding the TypeError: An Integer Is Required
The TypeError: an integer is required usually pops up in scenarios where Python functions, methods, or operations expect an integer but receive something else, like a string or a float. For instance, if you try to use a string in a mathematical operation or pass a non-integer value to a function that strictly requires an integer, you’ll trigger this error.
To illustrate, consider the following example:
value = "10"
result = value + 5
This code will raise a TypeError because you cannot add a string and an integer directly.
Output:
TypeError: an integer is required
To fix this, you need to ensure that the values you are working with are of the correct type. In the next sections, we will explore various methods to resolve this issue.
Method 1: Type Casting
One of the most straightforward solutions to the TypeError: an integer is required is to use type casting. Type casting allows you to convert the variable into the desired data type. In this case, you can convert a string or float to an integer using the int()
function. Here’s how you can do it:
value = "10"
result = int(value) + 5
In this code snippet, we convert the string "10"
into an integer before performing the addition. This ensures that both operands are integers.
Output:
15
Type casting is a powerful tool in Python. It not only helps in resolving TypeErrors but also allows for more flexible and dynamic code. However, it’s essential to ensure that the value being cast is indeed convertible to an integer. For example, trying to cast a non-numeric string will still raise an error. Always handle such cases to avoid unexpected crashes in your code.
Method 2: Input Validation
Another effective method to tackle the TypeError is through input validation. This approach is particularly useful when dealing with user inputs or data from external sources. By validating the input before performing operations, you can ensure that only the correct data types are processed. Here’s an example:
value = input("Enter a number: ")
if value.isdigit():
result = int(value) + 5
else:
result = "Invalid input. Please enter an integer."
In this code, we first check if the input is a digit using the isdigit()
method. If it is, we convert it to an integer and proceed with the addition. Otherwise, we return a message indicating that the input is invalid.
Output:
Enter a number: 10
15
Input validation is crucial for creating robust applications. It prevents errors and provides users with clear feedback on their actions. By incorporating such checks, you can enhance the user experience while ensuring that your code runs smoothly.
Method 3: Using Try-Except Blocks
When dealing with operations that can potentially raise errors, using try-except blocks is a best practice. This method allows you to catch exceptions and handle them gracefully without crashing your program. Here’s how you can implement it to solve the TypeError:
value = "10.5"
try:
result = int(value) + 5
except ValueError:
result = "Invalid input. Please enter an integer."
In this example, we attempt to convert the string "10.5"
into an integer. Since this will raise a ValueError, our except block catches the error and provides a user-friendly message instead of crashing the program.
Output:
Invalid input. Please enter an integer.
Using try-except blocks not only helps in managing errors effectively but also improves the overall robustness of your code. It allows you to gracefully handle unexpected situations, making your application more user-friendly.
Conclusion
In this tutorial, we explored the TypeError: an integer is required in Python and discussed several effective methods to resolve it. From type casting and input validation to using try-except blocks, each approach has its unique advantages. By understanding the root causes of this error and applying the right solutions, you can enhance your coding skills and build more resilient applications. Remember, error handling is a vital part of programming, and mastering it will make you a better developer.
FAQ
-
What causes the TypeError: an integer is required?
This error occurs when a function or operation expects an integer but receives a different data type, such as a string or float. -
How can I convert a string to an integer in Python?
You can convert a string to an integer using theint()
function. For example,int("10")
will convert the string “10” to the integer 10. -
What is input validation, and why is it important?
Input validation is the process of checking user input to ensure it meets certain criteria before processing. It’s important because it prevents errors and enhances user experience. -
How do try-except blocks work in Python?
Try-except blocks allow you to handle exceptions in your code. You place code that might raise an error in the try block and define how to handle the error in the except block. -
Can I use type casting with non-numeric strings?
No, attempting to cast non-numeric strings to integers will raise a ValueError. Always ensure the string is numeric before casting.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedInRelated Article - Python TypeError
- How to Fix the Python TypeError: List Indices Must Be Integers, Not List
- How to Fix TypeError: Iteration Over Non-Sequence
- How to Fix TypeError: Must Be Real Number, Not STR
- How to Fix Python TypeError: Unhashable Type: List
- How to Solve the TypeError: Not All Arguments Converted During String Formatting in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python