Tuple Comprehension in Python
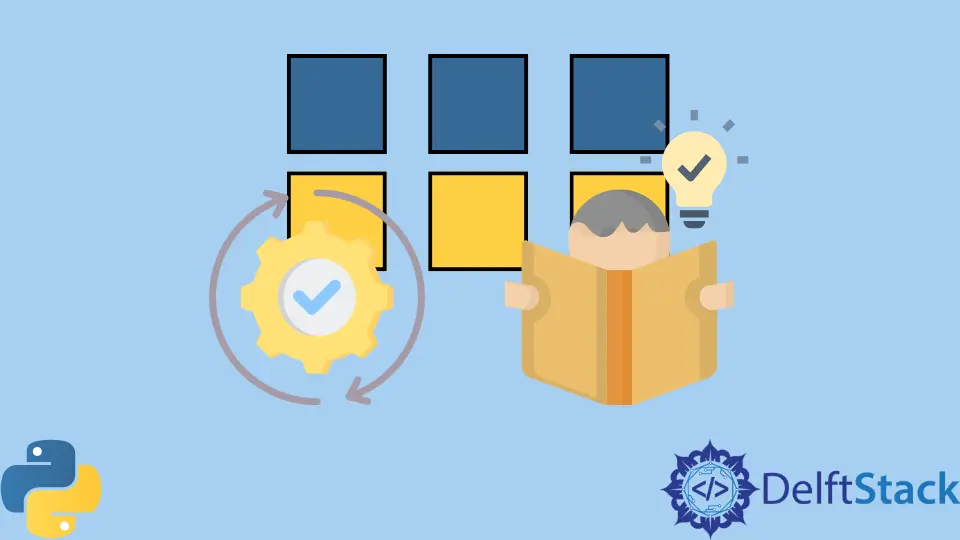
Python programming language has a straightforward and easy-to-understand syntax. The syntax is so simple that one can quickly write one-liner codes with Python. One such feature is list iteration or list comprehension. We can iterate over a list and return a new list with squares of list elements quickly by doing this [i ** 2 for i in [1, 2, 3, 4, 5, 6, 7]]
. The same applies to dictionaries; they can also be iterated over in a single line.
Python has various linear data structures such as lists, tuples, etc. The list comprehension shown above does not apply to tuples. This means we can’t perform (i ** 2 for i in (1, 2, 3, 4, 5, 6, 7))
. This will throw an error. If this is not possible, how do we perform tuple comprehension in a single line in Python? This article will talk about the same.
Tuple Comprehension in Python
One can perform tuple comprehension in Python using the following syntax.
x = tuple(i for i in (1, 2, 3, 4, 5, 6, 7))
print(x)
print(type(x))
y = tuple(i ** 2 for i in (1, 2, 3, 4, 5, 6, 7))
print(y)
print(type(y))
Output:
(1, 2, 3, 4, 5, 6, 7)
<class 'tuple'>
(1, 4, 9, 16, 25, 36, 49)
<class 'tuple'>
Python 3.5 came up with a new way to perform tuple comprehension. It was using the process of unpacking. We can use *
to perform unpacking. Refer to the following code for the same.
x = (*(i for i in [1, 2, 3, 4, 5, 6, 7]),) # Notice the comma (,) at the end
print(x)
print(type(x))
Output:
(1, 2, 3, 4, 5, 6, 7)
<class 'tuple'>
Do note that this syntax is equivalent to writing x = tuple([i for i in [1, 2, 3, 4, 5, 6, 7]])
.
x = tuple([i for i in [1, 2, 3, 4, 5, 6, 7]])
print(x)
print(type(x))
Output:
(1, 2, 3, 4, 5, 6, 7)
<class 'tuple'>