How to try Without except in Python
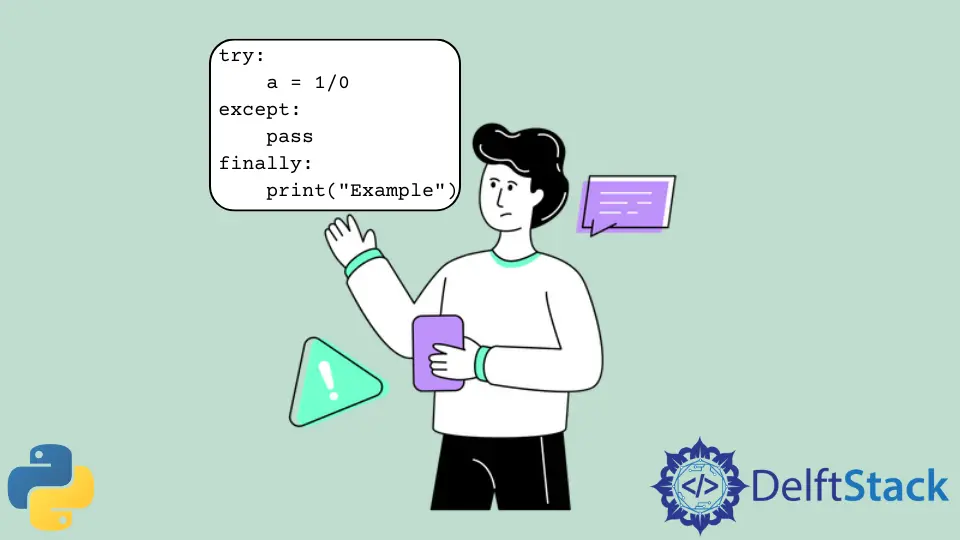
Exceptions in Python are the errors detected during the execution of the code. Different types of exceptions are NameError
, TypeError
, ZeroDivisionError
, OSError
and more.
The try
statement in Python is used to test a block of code for exceptions, and the except
statement is used to handle those exceptions. When the code in the try
block raises an error, the code in the except
block is executed.
We can catch all the exceptions, including KeyboardInterrupt
, SystemExit
and GeneratorExit
. This method should not be used to handle exceptions since it is a general statement and will hide all the trivial bugs.
We will discuss how to use the try
block without except
in Python. To achieve this, we should try to ignore the exception.
We cannot have the try
block without except
so, the only thing we can do is try to ignore the raised exception so that the code does not go the except
block and specify the pass
statement in the except
block as shown earlier. The pass
statement is equivalent to an empty line of code.
We can also use the finally
block. It will execute code regardless of whether an exception occurs or not.
try:
a = 1 / 0
except:
pass
finally:
print("Example")
Output:
Example
In the above code, if the try
block raises an error, the except
block will print the raised exception.
To ignore exceptions, we can use the suppress()
function from the contextlib
module to handle exceptions in Python
The suppress()
function from the contextlib
module can be used to suppress very specific errors. This method can only be used in Python 3.
For example,
from contextlib import suppress
with suppress(IndexError):
a = [1, 2, 3]
a[3]
In the above example, it will not raise the IndexError
.