tostring() Equivalent in Python
- Using str() Function
- Using join() Method for Lists
- Using f-Strings for Formatting
-
Using the
format()
Method - Using Custom str Method
- Conclusion
- FAQ
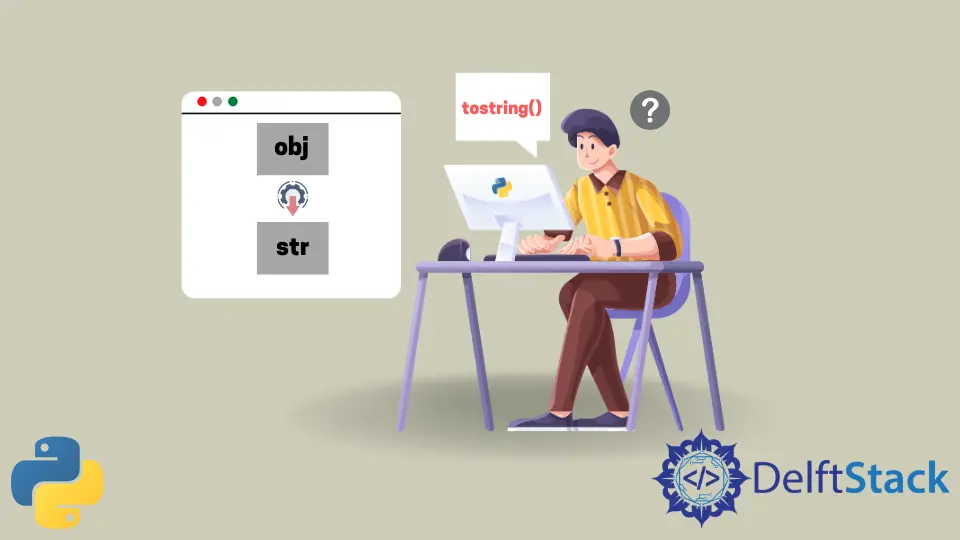
When working with data in Python, you may find yourself needing to convert various data types into strings for easier manipulation or display. In languages like Java, the tostring()
function serves this purpose, but in Python, there are several methods to achieve similar results.
This tutorial will guide you through the equivalent methods in Python to convert data into string format. Whether you’re dealing with lists, dictionaries, or custom objects, you’ll discover how to effectively use Python’s built-in functionalities and libraries to convert data types into strings. Let’s dive in!
Using str() Function
The simplest way to convert an object to a string in Python is by using the built-in str()
function. This function can take almost any data type as an argument and return its string representation.
Here’s a quick example:
number = 123
string_number = str(number)
print(string_number)
Output:
123
The str()
function is versatile and works with various data types, including integers, floats, lists, and even custom objects if they implement the __str__
method. In the example above, we converted an integer into a string. This method is straightforward and efficient, making it a go-to choice for many developers.
When using str()
, remember that the returned string is a representation of the object, which may not always capture the full complexity of the data structure. For instance, when you convert a list or dictionary, the output will be a string that looks like the list or dictionary itself, rather than a more user-friendly format.
Using join() Method for Lists
If you have a list of strings and want to concatenate them into a single string, the join()
method is an excellent choice. This method is particularly useful for creating a readable string from a collection of string elements.
Consider the following example:
words = ["Hello", "world", "from", "Python"]
sentence = " ".join(words)
print(sentence)
Output:
Hello world from Python
In this example, we created a list of words and then used the join()
method to concatenate them into a single sentence. The string " "
serves as the separator between each word. You can customize this separator to suit your needs, whether it’s a comma, space, or any other character.
The join()
method is not only efficient but also elegant. It avoids the need for cumbersome loops and string concatenation operators, making your code cleaner and more readable. Keep in mind that all elements in the list must be strings; otherwise, a TypeError
will be raised.
Using f-Strings for Formatting
Introduced in Python 3.6, formatted string literals, or f-strings, provide a powerful way to embed expressions inside string literals. This method is particularly useful when you want to include variable values within a string.
Here’s how you can use f-strings:
name = "Alice"
age = 30
greeting = f"My name is {name} and I am {age} years old."
print(greeting)
Output:
My name is Alice and I am 30 years old.
In this example, we defined two variables, name
and age
, and then created a greeting string using an f-string. The expressions inside the curly braces {}
are evaluated at runtime and the result is included in the final string.
F-strings are not only concise but also highly readable, making it easy to format strings dynamically. They also support a variety of formatting options, allowing you to control how numbers, dates, and other data types are represented as strings. This makes f-strings a preferred method for many Python developers when creating formatted output.
Using the format()
Method
Another way to convert and format strings in Python is by using the format()
method. This method allows you to create complex string representations by using placeholders.
Here’s an example of how to use the format()
method:
item = "apple"
quantity = 5
message = "I have {0} {1}s.".format(quantity, item)
print(message)
Output:
I have 5 apples.
In this example, the format()
method replaces the placeholders {0}
and {1}
with the values of quantity
and item
, respectively. This method is especially useful when you need to construct strings from multiple variables or when you want to control the order of insertion.
The format()
method is versatile and can handle various data types, including numbers, strings, and even complex objects. However, with the introduction of f-strings, many developers prefer the latter for its simplicity and readability. Nonetheless, format()
remains a powerful tool for string manipulation in Python, especially for older versions of the language.
Using Custom str Method
If you are working with custom objects in Python, you can define your own string representation by implementing the __str__
method in your class. This method is called when you use the str()
function or print()
on an instance of your class.
Here’s an example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __str__(self):
return f"{self.name} is {self.age} years old."
person = Person("Bob", 25)
print(person)
Output:
Bob is 25 years old.
In this example, we created a Person
class with a custom __str__
method. When we print the person
object, Python calls the __str__
method, returning a formatted string that describes the object. This allows for a more meaningful string representation of your objects, which is particularly useful for debugging and logging purposes.
Defining a custom __str__
method is a best practice when creating classes in Python, as it enhances the readability of your code and provides a clear way to represent your objects as strings.
Conclusion
In summary, Python offers several methods for converting various data types to strings, each with its unique advantages. Whether you choose to use the str()
function for simple conversions, join()
for list concatenation, f-strings for dynamic formatting, or the format()
method for more complex strings, you have a variety of tools at your disposal. Implementing the __str__
method in your custom classes can further enhance how your objects are represented as strings. By understanding these different methods, you can choose the most appropriate one for your specific use case, making your Python programming experience smoother and more efficient.
FAQ
-
What is the str() function in Python?
The str() function is a built-in method used to convert various data types into their string representation. -
Can I convert a list of integers to a single string?
Yes, you can use the join() method, but you’ll need to convert the integers to strings first. -
What are f-strings in Python?
F-strings are formatted string literals introduced in Python 3.6 that allow you to embed expressions inside string literals using curly braces. -
How do I create a custom string representation for my class?
You can define a str method in your class, which returns a string that describes the object. -
Is the format() method still relevant in Python?
Yes, while f-strings are more popular for formatting, the format() method is still widely used and remains a powerful option for string manipulation.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn