How to Suppress Warnings in Python
- Understanding Warnings in Python
- Using the warnings Module
- Suppressing Specific Warnings
- Context Manager for Temporary Suppression
- Conclusion
- FAQ
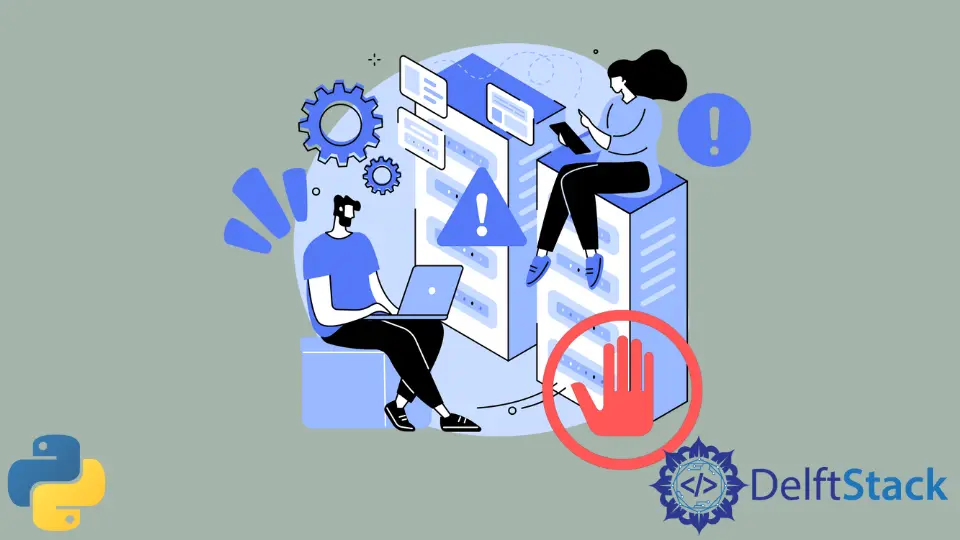
Warnings in Python can sometimes clutter your output, especially when you’re working on large projects or during development. While these warnings serve a purpose, they can be distracting.
This tutorial demonstrates effective methods to suppress warnings in your Python programs, allowing you to focus on what matters most. Whether you’re dealing with deprecated features or other non-critical alerts, knowing how to manage these warnings can enhance your coding experience. Let’s dive into the various techniques you can use to suppress warnings in Python.
Understanding Warnings in Python
Before we jump into the methods for suppressing warnings, it’s important to understand what they are. Warnings in Python are messages that inform you about conditions in your code that may not necessarily be errors but could lead to issues down the line. For example, using deprecated functions or libraries might generate warnings that suggest alternatives. While you might want to address these warnings eventually, there are times when you prefer to suppress them temporarily, especially during development or testing phases.
Python provides a built-in module called warnings
that allows you to control the visibility of these messages. Let’s explore how to use this module effectively.
Using the warnings Module
The warnings
module is the primary way to manage warnings in Python. It offers various functions to filter and suppress warnings based on your needs. Here’s a simple way to suppress all warnings in your Python script.
import warnings
warnings.filterwarnings("ignore")
# Example code that might generate a warning
def deprecated_function():
warnings.warn("This function is deprecated", DeprecationWarning)
deprecated_function()
Output:
In this example, we import the warnings
module and use the filterwarnings
function to ignore all warnings. The string “ignore” tells Python to suppress these messages. The deprecated_function()
generates a warning, but you won’t see it in the output because we’ve set the filter to ignore warnings. This is particularly useful when you know that the warnings are not critical for your current work.
The warnings
module also allows for more granular control. You can specify which warnings to ignore, or even which warnings to display. This flexibility makes it a powerful tool for managing your code’s output.
Suppressing Specific Warnings
Sometimes, you may want to suppress only specific warnings rather than all warnings. This can be done by providing more details in the filterwarnings
function. Here’s how you can achieve that:
import warnings
# Suppress only the DeprecationWarning
warnings.filterwarnings("ignore", category=DeprecationWarning)
def deprecated_function():
warnings.warn("This function is deprecated", DeprecationWarning)
def another_function():
warnings.warn("This is just a warning", UserWarning)
deprecated_function()
another_function()
Output:
This is just a warning
In this code snippet, we specify that only DeprecationWarning
should be ignored. When deprecated_function()
is called, its warning is suppressed. However, the warning from another_function()
is still displayed because it is a UserWarning
. This targeted suppression is useful when you want to keep an eye on certain warnings while ignoring others.
Context Manager for Temporary Suppression
Another effective method for suppressing warnings in Python is to use a context manager. This allows you to suppress warnings only within a specific block of code, which can be very handy for testing or when you know that a particular section of code may generate warnings.
import warnings
def deprecated_function():
warnings.warn("This function is deprecated", DeprecationWarning)
with warnings.catch_warnings():
warnings.simplefilter("ignore")
deprecated_function()
# This warning will still be shown
deprecated_function()
Output:
This function is deprecated
In this example, we use warnings.catch_warnings()
as a context manager. Inside the with
block, we set the filter to ignore warnings. The warning generated by deprecated_function()
within this block is suppressed. However, when we call deprecated_function()
outside the block, the warning is displayed again. This method is particularly useful when you want to suppress warnings temporarily without affecting the global warning settings.
Conclusion
Suppressing warnings in Python is a straightforward process, thanks to the built-in warnings
module. Whether you choose to ignore all warnings, target specific ones, or use context managers for temporary suppression, you have the tools to manage your output effectively. By implementing these techniques, you can maintain a cleaner and more focused coding environment, allowing you to concentrate on your project without distractions. Remember, while it’s okay to suppress warnings during development, it’s essential to address them eventually to ensure the long-term health of your code.
FAQ
-
What is the purpose of warnings in Python?
Warnings in Python inform you about potential issues in your code that may not be critical errors but could lead to problems later. -
Can I suppress warnings globally in Python?
Yes, you can use thewarnings.filterwarnings()
function to suppress warnings globally throughout your script. -
How do I suppress only specific warnings in Python?
You can specify the category of warnings you want to suppress using thefilterwarnings
function, such asDeprecationWarning
. -
What is a context manager in Python?
A context manager is a construct that allows you to allocate and release resources precisely when you want to. In this case, it helps manage warning suppression temporarily. -
Is it safe to ignore all warnings in Python?
While it may be convenient to ignore all warnings, it’s essential to review and address them eventually to ensure your code remains robust and functional.