How to Get Sum of a List in Python
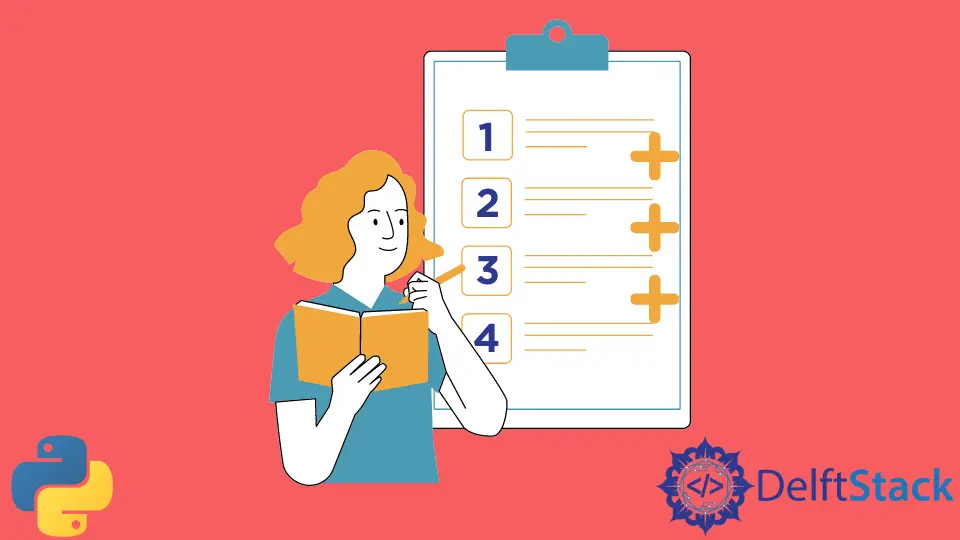
The list is one of the most commonly used data structures in Python. In other programming languages, they are regarded as arrays, and they have the same functionality.
Sum a List in Python With the sum()
Function
The most basic and simplistic way to sum a list in Python is to use Python’s in-built function sum()
.
myList = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
listSum = sum(myList)
print(f"Sum of list -> {listSum}")
Output:
Sum of list -> 55
The sum()
function accepts two arguments. The first argument is an iterable data structure, and the second argument is the start index. An iterable data structure could be a list of numbers, Python dictionaries, and tuples. And the start index is basically the position in the iterable data structure from where the summing process should start.
If a list of anything other than numeric values is directly provided to the sum()
method, it will raise a TypeError
.
In such cases, you’ve to filter the data, or other words, preprocess the data.
For example, if you have a list of numeric strings, you must first convert strings to their numeric equivalents and then sum them. Refer to the following code for the same.
myList = ["1", "3", "5", "7", "9"]
myNewList = [int(string) for string in myList]
sum1 = sum(myNewList)
sum2 = sum(number for number in myNewList)
print(f"Sum of list -> {sum1}")
print(f"Sum of list -> {sum2}")
Output:
Sum of list -> 25
Sum of list -> 25
Get Sum of a List by Iteration Over List
myList = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
listSum = 0
for number in myList:
listSum += number
print(f"Sum of list -> {listSum}")
Output:
Sum of list -> 55
The above code iterates over each element of the list, adds them to a variable, and finally prints the sum.
If you want to follow a more conventional way of iterating over the indexes and accessing elements using them, refer to the following code snippet.
myList = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
length = len(myList)
listSum = 0
for i in range(length):
listSum += myList[i]
print(f"Sum of list -> {listSum}")
Output:
Sum of list -> 55
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python