String Builder Equivalent in Python
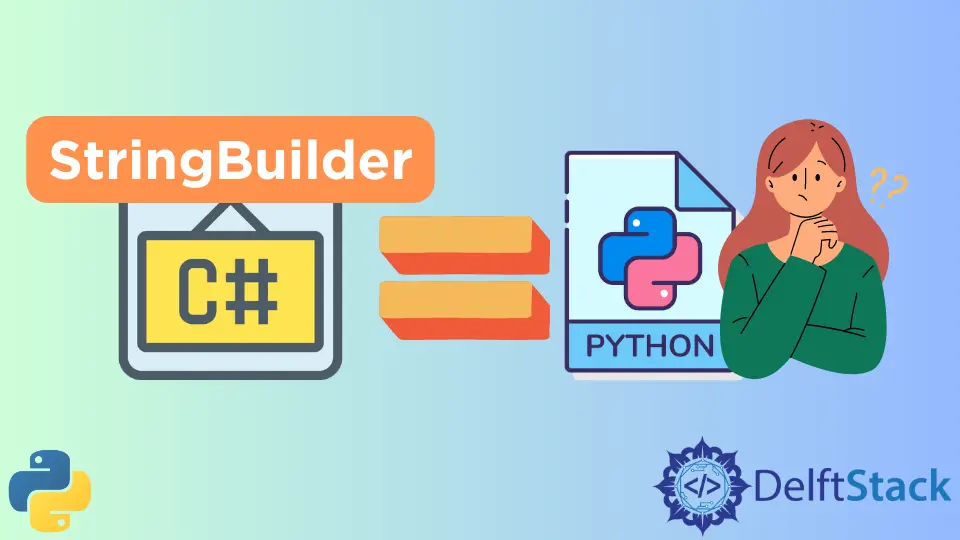
A string is a collection of characters and is an immutable object. We have to specify a new memory block for every change in a string rather than altering the already existing block.
The StringBuilder
class in C# programming creates mutable string objects and allows dynamic memory allocation. We don’t have such calss in Python, but we can use string concatenation to achieve this and create long efficient string objects.
The main idea behind this is that we take a list of strings and join them to build large strings.
Use the join()
Function
To join strings from a list, we can use the join()
function. The join()
method returns a string after combining all the elements from an iterable object. The following code snippet explains this.
mylist = ["abcd" for i in range(5)]
mystring = "".join(mylist)
print(mystring)
Output:
abcdabcdabcdabcdabcd
There are many other ways to concatenate strings from a list, but the join()
function is considered the fastest of them all.
Use the String Concatenation Method
We can easily concatenate strings from a list using the for
loop and +
operator. The following example shows how.
mylist = ["abcd" for i in range(5)]
mystring = ""
for i in range(len(mylist)):
mystring += mylist[i]
print(mystring)
Output:
abcdabcdabcdabcdabcd
Use the StringIO
Module
The StringIO
module can be used to read and write strings in the memory buffer. We create a StringIO
object and write it to this object after looping through the list. We can print the required string using the getvalue()
method. For example,
file_str = StringIO()
for i in range(len(mylist)):
file_str.write(mylist[i])
print(file_str.getvalue())
Output:
abcdabcdabcdabcdabcd
We can also create a class of our own that can append strings using functions from the StringIO
module. For example,
from io import StringIO
class StringBuilder:
_file_str = None
def __init__(self):
self._file_str = StringIO()
def Append(self, str):
self._file_str.write(str)
def __str__(self):
return self._file_str.getvalue()
sb = StringBuilder()
sb.Append("Hello ")
sb.Append("World")
print(sb)
Output:
Hello World
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn