How to Convert a String to Binary in Python
-
Convert a String to Its Binary Representation in Python Using the
format()
Function -
Convert a String to Its Binary Representation in Python Using the
bytearray
Method -
Convert a String to Its Binary Representation in Python Using the
map()
Function -
Convert a String to Its Binary Representation in Python Using the
ASCII
Method
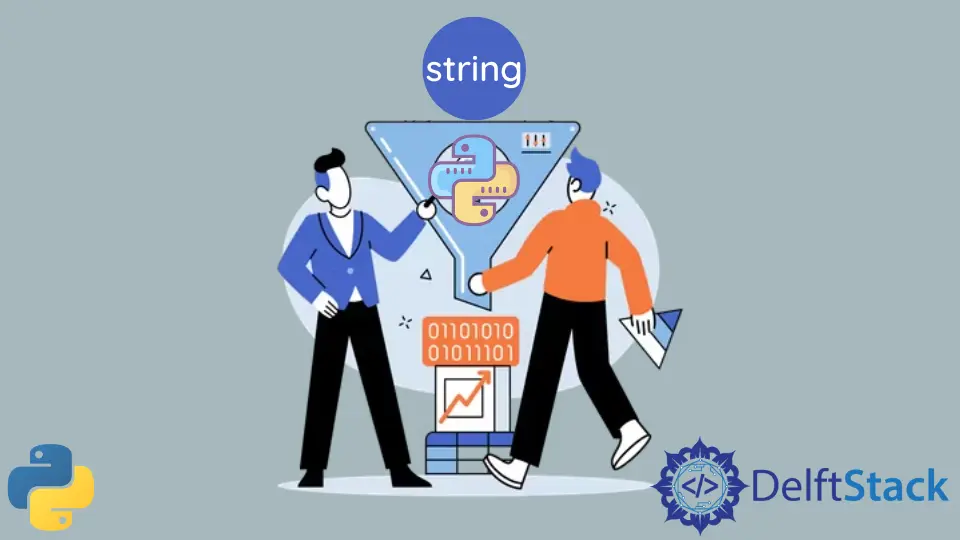
When a string is converted to binary, it generates a list of binary values representing the original characters. Each character must be iterated over and converted to binary.
This article will discuss some methods to convert a string to its binary representation in Python.
Convert a String to Its Binary Representation in Python Using the format()
Function
We use the ord()
function that translates the Unicode point of the string to a corresponding integer. The format()
function converts an integer to a number in base two using the b
binary format.
The complete example code is given below.
string = "Python"
binary_converted = " ".join(format(ord(c), "b") for c in string)
print("The Binary Representation is:", binary_converted)
Output:
The Binary Represntation is: 1010000 1111001 1110100 1101000 1101111 1101110
Convert a String to Its Binary Representation in Python Using the bytearray
Method
A byte array is a set of bytes that may store a list of binary data. Instead of iterating over the string explicitly, we can iterate over a byte sequence. It can be achieved without using the ord()
function, but using the bytearray()
function.
The complete example code is given below.
string = "Python"
binary_converted = " ".join(format(c, "b") for c in bytearray(string, "utf-8"))
print("The Binary Represntation is:", binary_converted)
Output:
The Binary Representation is: 1010000 1111001 1110100 1101000 1101111 1101110
Convert a String to Its Binary Representation in Python Using the map()
Function
We can also use the map()
function in replacement of the format()
function. map()
convert string to a byte array using the bytearray()
function and then use bin
to convert the array of bytes in binary representation.
The complete example code is given below.
string = "Python"
binary_converted = " ".join(map(bin, bytearray(string, "utf-8")))
print("The Binary Represntation is:", binary_converted)
In Python 3, we must define an encoding scheme like utf-8
; otherwise, an error will be raised.
Output:
The Binary Represntation is: 0b1010000 0b1111001 0b1110100 0b1101000 0b1101111 0b1101110
Convert a String to Its Binary Representation in Python Using the ASCII
Method
In Python 3, utf-8
is the default encoding scheme. But this method will use an ASCII
encoding scheme instead of utf-8
. For basic alphanumeric strings, the variations between UTF-8
and ASCII
encoding are not noticeable. But they will become significant if we are processing text that incorporates characters that are not part of the ASCII
character collection.
The complete example code is given below:
st = "Python"
a_bytes = bytes(st, "ascii")
binary_converted = " ".join(["{0:b}".format(x) for x in a_bytes])
print("The Binary Represntation is:", binary_converted)
Output:
The Binary Representation is: 1010000 1111001 1110100 1101000 1101111 1101110
Related Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python