How to Captilize String in Python
-
String Capitalization With the
upper()
Function in Python -
String Capitalization With the
capitalize()
Function in Python
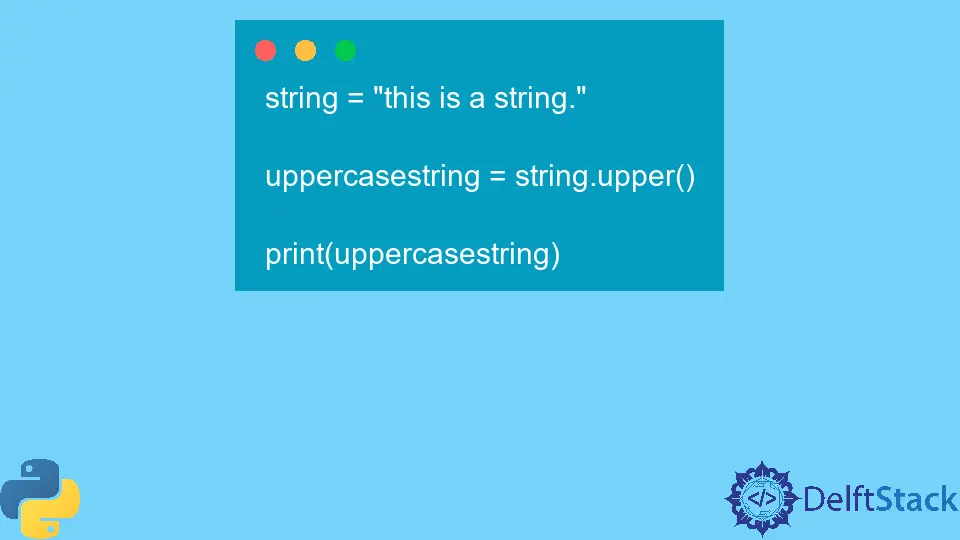
In this tutorial, we will discuss methods used for string capitalization in Python.
String Capitalization With the upper()
Function in Python
The upper()
function gives us a string with all uppercase characters. The following code example shows us how we can use the upper()
function to capitalize a string in Python.
string = "this is a string."
uppercasestring = string.upper()
print(uppercasestring)
Output:
THIS IS A STRING
In the above code, we first initialize a string with all lowercase characters. After that, we convert the string to uppercase using the upper()
function.
The upper()
converts all the letters to uppercase. If we only want to capitalize the first character in the string, we have to use the capitalize()
function.
String Capitalization With the capitalize()
Function in Python
The capitalize()
function only converts the first letter of the string to uppercase. The following code example shows how we can capitalize a string using the capitalize()
function.
string = "this is a string."
capitalizedstring = string.capitalize()
print(capitalizedstring)
Output:
This is a string.
In the above code, we first initialize a string with all lowercase characters. After that, we capitalize the first character of the string using the capitalize()
function.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn