How to Stop a for Loop in Python
Najwa Riyaz
Feb 02, 2024
-
Use a
break
Statement to Stop a Pythonfor
Loop -
Wrap the Code in a Function, and Then Use the
return
Statement -
Raise an Exception to Stop a Python
for
Loop
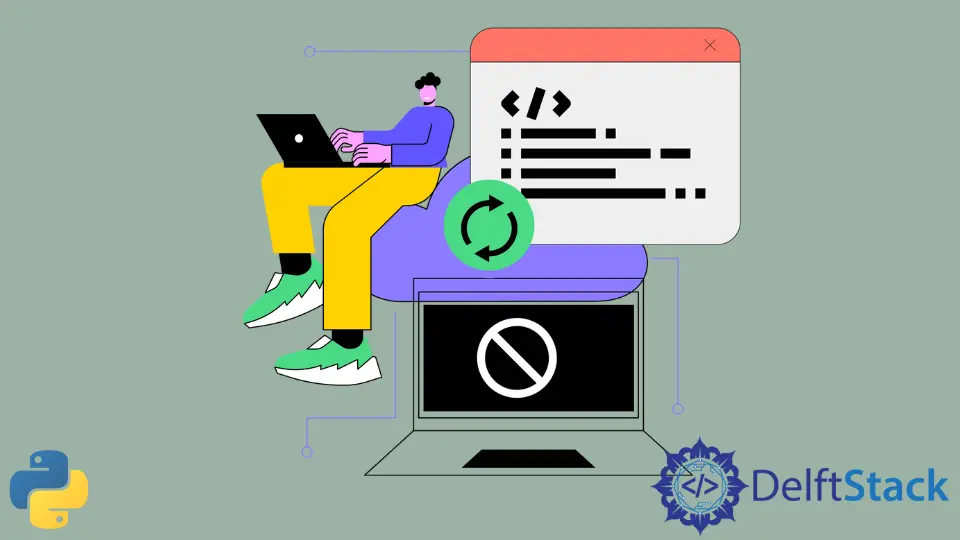
This article introduces different methods to stop a for
loop in Python.
Use a break
Statement to Stop a Python for
Loop
Use a break
statement to stop a for
loop in Python.
For example,
max = 4
counter = 0
for a in range(max):
if counter == 3:
print("counter value=3. Stop the for loop")
break
else:
print("counter value<3. Continue the for loop. Counter value=", counter)
counter = counter + 1
continue
break
Output:
counter value<3. Continue the for loop. Counter value= 0
counter value<3. Continue the for loop. Counter value= 1
counter value<3. Continue the for loop. Counter value= 2
counter value=3. Stop the for loop
Here, as long as the for
loop criteria is met, the following print statement is printed. For example -
counter value<3. Continue the for loop. Counter value= 0
However, once the counter
value is equal to 3
, it breaks out of the for loop. Hence, the for
loop stops.
Wrap the Code in a Function, and Then Use the return
Statement
Wrap the code in a function, and then use the return
statement.
For example,
def fncreturn():
return
max = 4
counter = 0
for a in range(max):
if counter == 3:
print("counter value=3. Stop the for loop")
fncreturn()
else:
print("counter value<3. Continue the for loop. Counter value=", counter)
counter = counter + 1
continue
break
Output:
counter value<3. Continue the for loop. Counter value= 0
counter value<3. Continue the for loop. Counter value= 1
counter value<3. Continue the for loop. Counter value= 2
counter value=3. Stop the for loop
Here, when the counter value reaches to 3
, the function is called. The function has just a return
statement. Post that, it helps exit from the for loop.
Raise an Exception to Stop a Python for
Loop
Raise an exception to stop a for
loop.
For example,
max = 4
counter = 0
try:
for a in range(max):
if counter == 3:
print("counter value=3. Stop the for loop")
raise StopIteration
else:
print("counter value<3. Continue the for loop. Counter value=", counter)
counter = counter + 1
except StopIteration:
pass
Output:
counter value<3. Continue the for loop. Counter value= 0
counter value<3. Continue the for loop. Counter value= 1
counter value<3. Continue the for loop. Counter value= 2
counter value=3. Stop the for loop
Here, when the counter value reaches to 3
, the exception is raised. Immediately it exits from the for
loop.