Static Class Variables in Python
-
Use the
staticmethod()
to Define Static Variables in Python -
Use the
@staticmethod
to Define Static Variables in Python
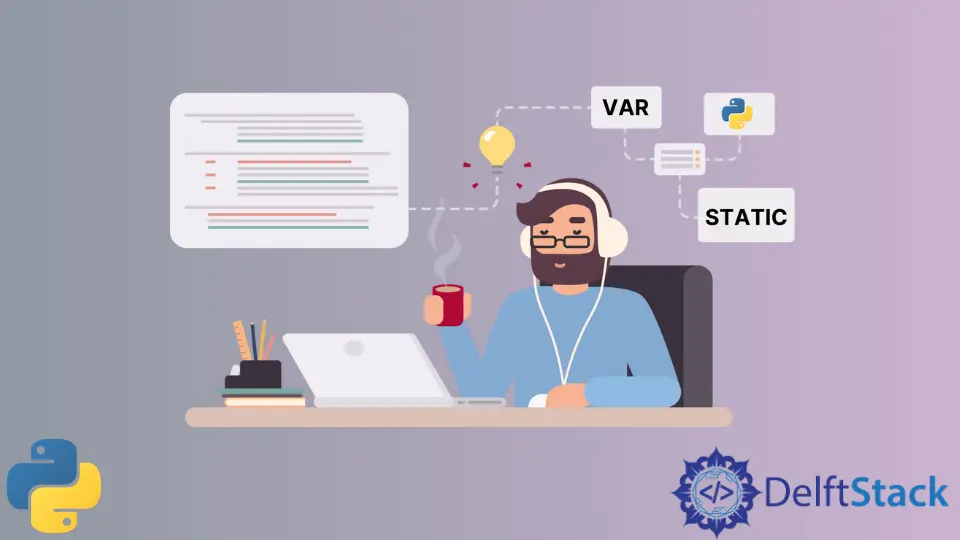
A static variable in Python is a variable that is declared inside a defined class but not in a method. This variable can be called through the class inside which it is defined but not directly. A Static variable is also called a class variable. These variables are confined to the class, so they cannot change the state of an object.
This tutorial will demonstrate different ways of defining static variables in Python.
Use the staticmethod()
to Define Static Variables in Python
The staticmethod()
in Python is a built-in function used to return a static variable for a given function.
This method is now considered to be an old way of defining a static variable in Python.
Example:
class StaticVar:
def random(text):
print(text)
print("This class will print random text.")
StaticVar.random = staticmethod(StaticVar.random)
StaticVar.random("This is a random class.")
Output:
This is a random class.
This class will print random text.
Here, first, we make a class called StaticVar
. In the program, we declare a variable called random
as a static variable outside the class by using staticmethod()
function. By this, we can call the random()
directly using the StaticVar
class.
Use the @staticmethod
to Define Static Variables in Python
@staticmethod
is a modern and the most used way to define a static variable. The @staticmethod
is a built-in decorator in Python. A decorator is a designed pattern in Python used to make a new functionality to an already existing object without changing its initial structure. So, the @staticmethod
decorator is used to define a static variable inside a class in Python.
Example:
class StaticVar:
@staticmethod
def random(text):
# show custom message
print(text)
print("This class will print random text.")
StaticVar.random("This is a random class.")
Output:
This is a random class.
This class will print random text.
Note that the @staticmethod
decorator is defined before defining the static variable random
. Due to this, we can easily call the random
variable at the end through the StaticVar
class.
Also, note that, in both the methods, we do not use the self
argument, which is used to access the function attributes and methods while defining the random
variable. It is because static variables never operate through objects.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn