Static Class in Python
-
Use the
@staticmethod
Decorator to Create a Static Class in Python -
Use the
@classmethod
Decorator to Create a Static Class in Python - Use a Module File to Create a Static Class in Python
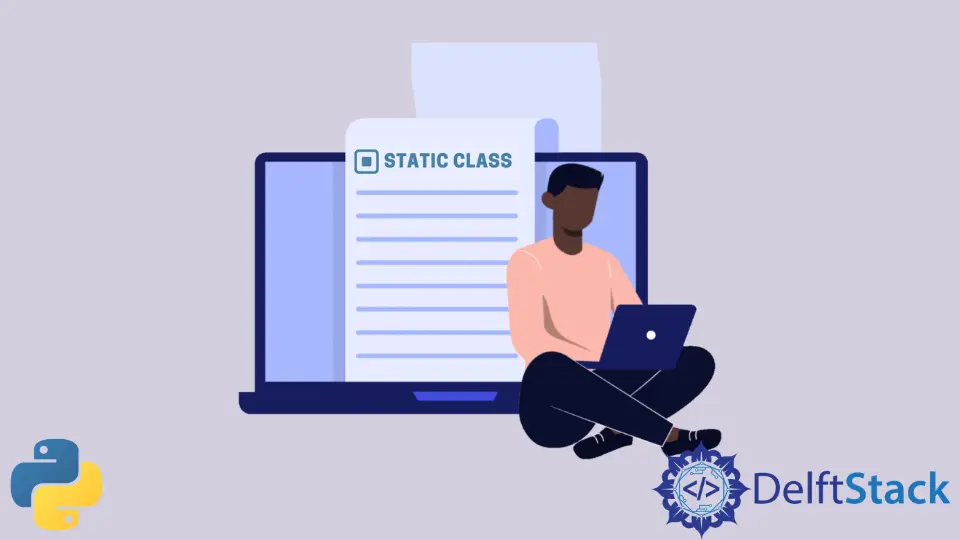
A static class is a handy feature in programming. A static class cannot be inherited and cannot be instantiated. There is no direct way to make a class static. In Python, we can implement a static class by making its methods and variables static.
In this article, we will implement such methods.
Use the @staticmethod
Decorator to Create a Static Class in Python
To implement a static class, we can make its methods and variables static. For this, we can use the @staticmethod
decorator to create methods in a class static. A decorator is a special function specified before a function and takes the whole function as a parameter.
For example,
class abc(object):
@staticmethod
def static_method():
print("Hi,this tutorial is about static class in python")
abc.static_method()
Output:
Hi,this tutorial is about static class in python
Note that this method only works on versions of Python higher than 2.6.
Use the @classmethod
Decorator to Create a Static Class in Python
The @classmethod
can make a method static to the class and not its object. It has several advantages over the @staticmethod
decorator. It will also work with subclasses and can modify the class state.
However, it requires a compulsory argument to be included in the method called cls
.
See the following code.
class abc(object):
@classmethod
def static_method(cls):
print("Hi,this tutorial is about static class in python")
abc.static_method()
Output:
Hi,this tutorial is about static class in python
Use a Module File to Create a Static Class in Python
In Python, probably the best way to implement a static class is by creating a module. We can import a module, and its functions can be accessed using the module name. It does not require any objects to be instantiated, and it cannot be inherited.
See the following example.
def static_method():
print("Hi this is the content of the module file")
The above code can be a simple function in a Python script that can be imported as a module.
For example, if the name of the above Python script is abc.py
, we can do something as shown below.
import abc
abc.static_method()
Output:
Hi,this tutorial is about static class in python