How to Start A Thread in Python
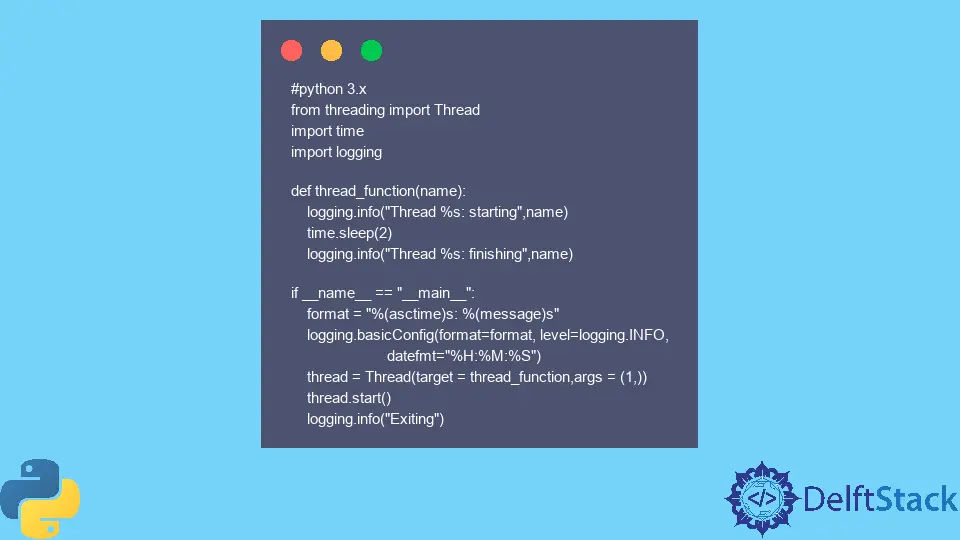
This tutorial will introduce the threading
module to create and start a thread in Python.
Thread Definition
A thread is a set of operations that need to be executed. Running threads means that the program will have two or more things running at once. A thread can only run in one core of the CPU. Threading is about how we handle the threads in one CPU core.
Thread Implementation in Python
Python uses the threading
module to implement threads in programs. You can create a Thread
instance and then call it to start a separate thread.
When you create a Thread
instance, you pass in a function and a list of arguments for that function. In this case, you are telling the Thread
to run the function thread_function()
and pass it 1 as an argument.
The thread_function()
logs some messages and sleeps for two seconds.
# python 3.x
from threading import Thread
import time
import logging
def thread_function(name):
logging.info("Thread %s: starting", name)
time.sleep(2)
logging.info("Thread %s: finishing", name)
if __name__ == "__main__":
format = "%(asctime)s: %(message)s"
logging.basicConfig(format=format, level=logging.INFO, datefmt="%H:%M:%S")
thread = Thread(target=thread_function, args=(1,))
thread.start()
logging.info("Exiting")
Output:
10:23:58: Thread 1: starting
10:23:58: Exiting
10:24:00: Thread 1: finishing
Threading Visualization
The flow of the program is given below. Once you call start()
, it triggers thread_function()
and it runs in a separate thread. The main program also runs in parallel as another thread.