How to Fix Sqlite3.OperationalError: Unable to Open Database File
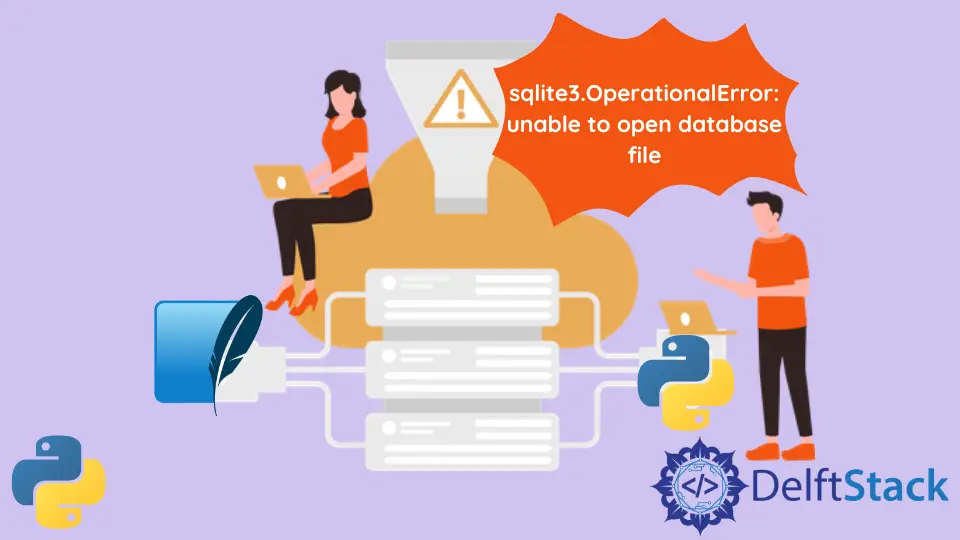
This article teaches how to counter the operational error sqlite3.OperationalError: unable to open database file
.
sqlite3.OperationalError: unable to open database file
Error in SQLite
We need to address the below questions to resolve the error.
-
Is the software being tested on the same computer as you’re testing it?
-
Is it running as the same user as you’re testing it?
-
Is the
/tmp
directory on the disc full? -
Are the permissions on the
/tmp/cer
directory odd?SQLite must be able to create additional files in it to handle things like the commit log.
-
Does the unit test code still use the database?
Concurrent openings are feasible with a contemporary SQLite and the correct filesystem - however,
/tmp
is almost always on the right kind of FS, so it’s probably not that - but it’s still not advised. -
Is the development code attempting to write to that database, or is something “smart” catching you off guard and leading it to attempt to open something else?
-
Are the unit tests and production code using the same SQLite library version?
It’s conceivable that the production system doesn’t have a /tmp/cer
directory if you’re not on the same computer. It is self-evident that this must be addressed first.
Similarly, if you’re operating as several users on the same system, you will likely run into permissions/ownership issues. Another potential snare is a lack of disc space.
It’s probably not the final three, but if the more obvious deployment issues have been resolved, they’re worth reviewing.
If none of the following applies, you’ve encountered an unusual situation and will need to provide considerably more information (it might even be a bug in SQLite, but knowing the developers of it, it’s quite unlikely).
In these ways, we can resolve the sqlite3.OperationalError: unable to open database file
.