How to Install SQLite in Visual Studio 2022
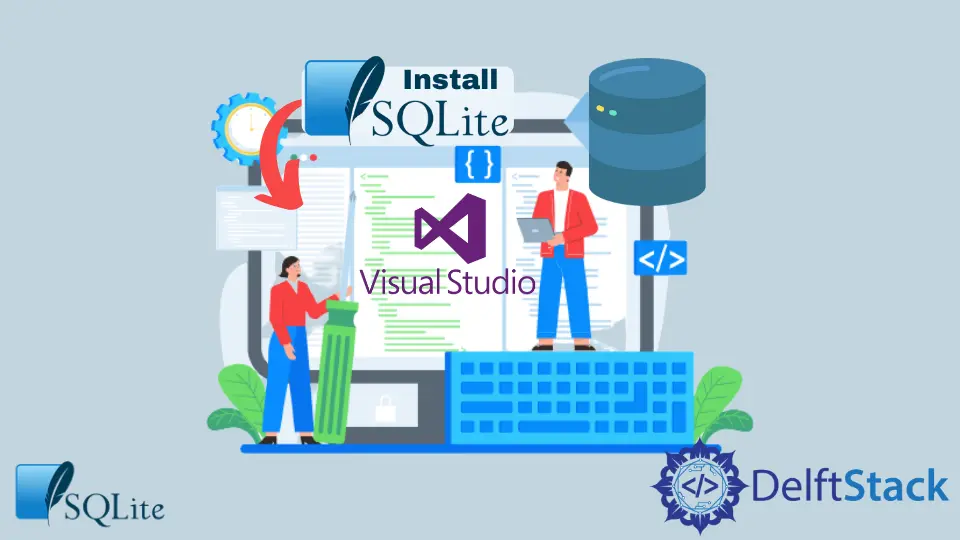
In the following tutorial, we will be learning how to install SQLite in Visual Studio 2022.
You often find using SQLite in Visual Studio 2022 a tedious task, but the procedure to implement it is fairly easy and can be done in simple steps.
Install SQLite in Visual Studio 2022
Follow the steps below to use the SQLite in Visual Studio 2022.
-
Open the Visual Studio 2022 Community application on your computer.
-
Click
Online
underTools - Extensions and Updates
. -
Look for
SQLite
. -
We can have a look at
SQLite Compact Toolbox
. -
Now click on
Install
.Once you hit the
Install
button, the download will start. You can see it below.Once the download is completed, you’ll see that the required program has been installed. This can be understood with the help of the image below.
-
The final step involves restarting the Visual Studio.
Close the Visual Studio tab and restart the tab to get it up and to run. Once installed, there’s a new menu item in Visual Studio’s
Tools
menu:SQL Server Compact/SQLite Toolbox
.
This way, we can install and use SQLite in Visual Studio 2022 in the simple steps above.