How to Show Tables in SQLite
Preet Sanghavi
Feb 02, 2024
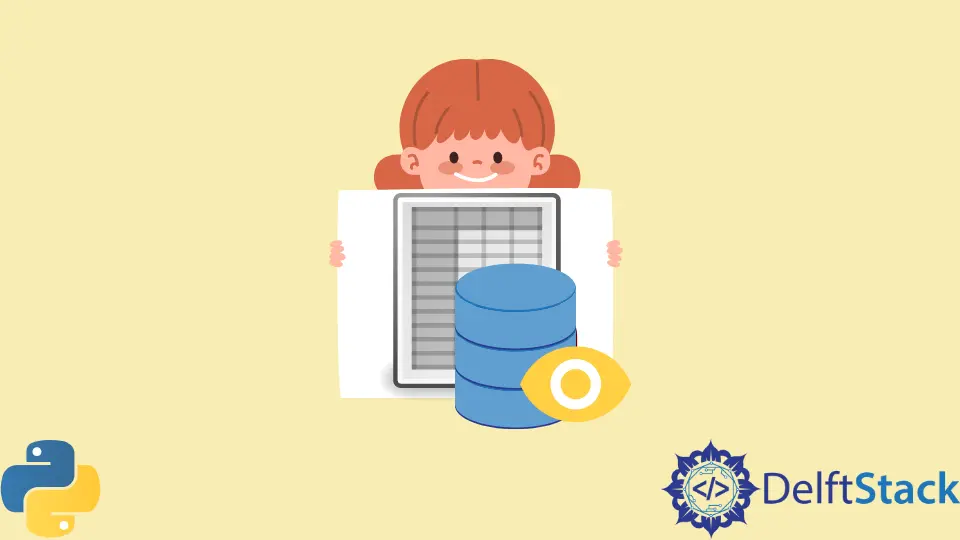
In this tutorial, we will learn how to show tables in SQLite.
Showing tables in SQLite serves as an essential step in performing database operations. We will be going through multiple ways to show tables in SQLite.
Show Tables in SQLite
Let us see the steps involved in the first approach to show tables.
-
List the tables in the database.
.tables
-
Let us see the appearance of tables in our database.
.schema table_name
-
Print the entire table.
SELECT * from table_name;
The helper functions .tables
and .schema
don’t search into ATTACHED databases; instead, they query the SQLITE MASTER table for the primary database.
-
You can search for the attached tables using the following:
ATTACH demo_file.db AS demo_db;
-
We need to write the below statement to show those files:
SELECT name FROM demo_db.sqlite_master WHERE type='table';
-
We must also note that temporary tables do not show up either. To show them, we need to list
sqlite_temp_master
:SELECT name FROM sqlite_temp_master WHERE type='table';
Using the abovementioned ways, we can show tables in SQLite employing different techniques.
Author: Preet Sanghavi