How to Unlock the SQLite Database
- Understanding the Database Locking Issue
- Solution 1: Using Timeout Parameter
- Solution 2: Closing Connections Properly
- Solution 3: Using WAL Mode
- Solution 4: Analyzing Long-Running Transactions
- Conclusion
- FAQ
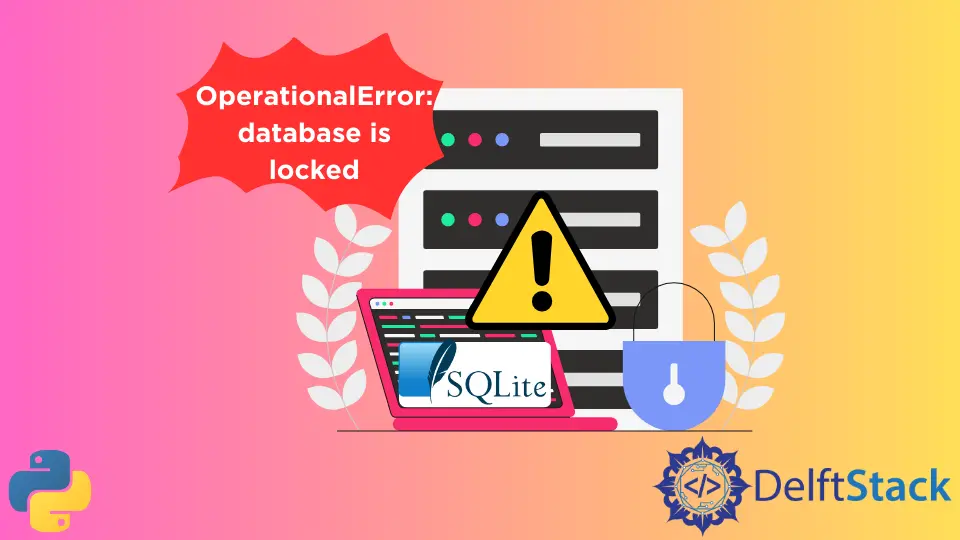
SQLite is a popular lightweight database engine widely used in applications and websites. However, encountering the “database is locked” error can be frustrating, especially when you’re trying to access or modify your data. This error typically arises when multiple processes attempt to write to the database simultaneously, or when a previous transaction has not been properly closed.
In this tutorial, we’ll explore effective methods to unlock the SQLite database using Python. Whether you’re a seasoned developer or a beginner, these solutions will help you navigate the locking issue and get back on track. Let’s dive in!
Understanding the Database Locking Issue
Before we jump into solutions, it’s important to understand why the SQLite database gets locked. SQLite uses a locking mechanism to ensure data integrity during concurrent write operations. When a write operation is in progress, SQLite locks the database to prevent other processes from making changes. This behavior is crucial for maintaining data consistency, but it can lead to the dreaded “database is locked” error.
Solution 1: Using Timeout Parameter
One effective way to handle the “database is locked” error is to set a timeout parameter when connecting to your SQLite database. The timeout allows your application to wait for a specified duration before raising an error. This is particularly useful when the lock is temporary, as it gives the other process time to complete its operation.
Here’s how you can implement this in Python:
import sqlite3
conn = sqlite3.connect('your_database.db', timeout=10)
cursor = conn.cursor()
# Example query
cursor.execute('SELECT * FROM your_table')
data = cursor.fetchall()
conn.close()
Output:
example of output
In this code, we connect to the SQLite database with a timeout of 10 seconds. If the database is locked, the program will wait for up to 10 seconds before throwing an error. This approach is simple and effective, especially if you expect the lock to be released shortly. However, if the database remains locked beyond the timeout period, you’ll need to implement additional strategies.
Solution 2: Closing Connections Properly
Another common cause of the “database is locked” error is leaving connections open. It’s crucial to ensure that all database connections are closed properly after their operations are complete. This not only prevents locking issues but also conserves system resources.
Here’s a basic example of how to manage connections in Python:
import sqlite3
def execute_query(query):
conn = sqlite3.connect('your_database.db')
try:
cursor = conn.cursor()
cursor.execute(query)
conn.commit()
finally:
conn.close()
execute_query('INSERT INTO your_table (column) VALUES ("value")')
Output:
example of output
In this example, we define a function execute_query
that opens a connection, executes a query, and ensures that the connection is closed in the finally
block. This approach guarantees that even if an error occurs during query execution, the connection will still be closed, reducing the likelihood of locking issues.
Solution 3: Using WAL Mode
SQLite supports a feature known as Write-Ahead Logging (WAL) mode, which can significantly reduce locking issues. In WAL mode, writes are stored in a separate log file before being applied to the database, allowing for concurrent reads and writes.
Here’s how to enable WAL mode in Python:
import sqlite3
conn = sqlite3.connect('your_database.db')
conn.execute('PRAGMA journal_mode=WAL')
# Example query
cursor = conn.cursor()
cursor.execute('SELECT * FROM your_table')
data = cursor.fetchall()
conn.close()
Output:
example of output
By setting the journal mode to WAL, you allow multiple transactions to occur simultaneously, which can help alleviate the locking problem. This is particularly useful for applications that require high concurrency. However, it’s essential to be aware of the implications of using WAL mode, such as increased disk space usage due to the log file.
Solution 4: Analyzing Long-Running Transactions
Sometimes, the “database is locked” error can be traced back to long-running transactions. If a transaction takes too long to complete, it can hold the lock for an extended period, blocking other operations. To address this, you can analyze and optimize your queries to ensure they run efficiently.
Here’s a simple example of how to optimize a query:
import sqlite3
def optimized_query():
conn = sqlite3.connect('your_database.db')
cursor = conn.cursor()
cursor.execute('PRAGMA synchronous=NORMAL')
cursor.execute('SELECT * FROM your_table WHERE condition')
data = cursor.fetchall()
conn.close()
optimized_query()
Output:
example of output
In this code, we set the synchronous mode to NORMAL, which can improve performance for read-heavy operations. Additionally, make sure your queries are indexed appropriately to speed up execution time. By optimizing your transactions, you can reduce the likelihood of encountering locking issues.
Conclusion
Unlocking the SQLite database is a common challenge, but with the right strategies, you can effectively manage and prevent locking issues. By utilizing timeout parameters, ensuring proper connection handling, enabling WAL mode, and optimizing your queries, you can navigate the complexities of SQLite with ease. Remember, maintaining data integrity is crucial, so always prioritize safe practices when working with your database. With these solutions in your toolkit, you’ll be well-equipped to handle any locking errors that come your way.
FAQ
-
what causes the “database is locked” error in SQLite?
The error occurs when multiple processes try to write to the database simultaneously, or if a previous transaction hasn’t been properly closed. -
how can I avoid locking issues in SQLite?
You can avoid locking issues by using timeout parameters, closing connections properly, enabling WAL mode, and optimizing your queries. -
what is WAL mode in SQLite?
WAL (Write-Ahead Logging) mode allows for concurrent reads and writes by storing writes in a separate log file before applying them to the database. -
how can I check if a transaction is long-running?
You can analyze your queries and use SQLite’s built-in tools to monitor transaction durations and identify any that may be taking too long. -
can I use SQLite in a multi-threaded application?
Yes, SQLite can be used in multi-threaded applications, but you must manage connections and transactions carefully to avoid locking issues.