How to Split a String Into a Character Array in Python
-
Use the
for
Loop to Split a String Into a Char Array in Python -
Use the
list()
Function to Split a String Into a Char Array in Python -
Use the
extend()
Function to Split a String Into a Char Array in Python -
Use the Unpacking Operator
*
to Split a String Into a Char Array in Python - Use the List Comprehension to Split a String Into a Char Array in Python
-
Use the
map()
Function to Split a String Into a Char Array in Python -
Use the
itertools.chain
Function to Split a String Into a Char Array in Python - Conclusion
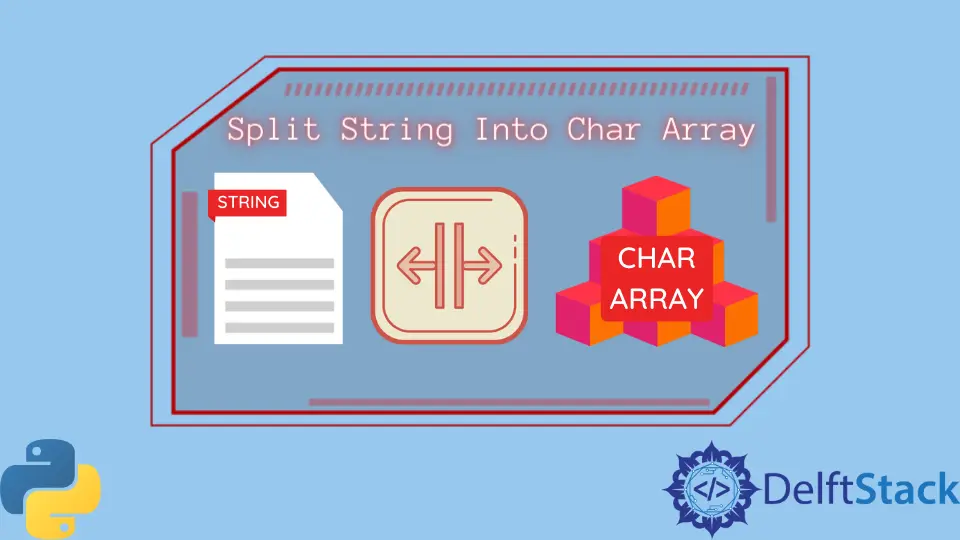
Strings are one of Python’s most fundamental data types, and working with them effectively is essential in many programming tasks. One common operation is splitting a string into individual characters, effectively converting it into an array of characters.
This article will explore multiple methods to split a string into a character array in Python. From traditional for
loops to more Pythonic approaches like list comprehensions and built-in functions, you will learn various techniques to perform this operation precisely and efficiently.
Use the for
Loop to Split a String Into a Char Array in Python
The simplest method to split a string into a character array is by iterating over the string with a for
loop. In this method, we use the for
loop to iterate over the string and append each character to an empty list.
word = "Sample"
lst = []
for i in word:
lst.append(i)
print(lst)
In the above code, we store the string in the variable word
, then initialize an empty list lst
. We use a for
loop to iterate over each character in word
.
We append the character to lst
in each iteration using the append()
function. Finally, we print the resulting character array.
Output:
['S', 'a', 'm', 'p', 'l', 'e']
Use the list()
Function to Split a String Into a Char Array in Python
Typecasting refers to the process of converting a datatype to some other datatype. We can typecast a string to a list using the list()
function, which splits the string into a char array.
word = "Sample"
lst = list(word)
print(lst)
Output:
['S', 'a', 'm', 'p', 'l', 'e']
Use the extend()
Function to Split a String Into a Char Array in Python
The extend()
function adds elements from an iterable object like a list, a tuple, and more to the end of a given list. Refer to this article to know more about the difference between the extend()
and append()
functions.
Since a string is a collection of characters, we can use it with the extend()
function to store each character at the end of a list.
lst = []
word = "Sample"
lst.extend(word)
print(lst)
Output:
['S', 'a', 'm', 'p', 'l', 'e']
Use the Unpacking Operator *
to Split a String Into a Char Array in Python
We can use the *
operator to perform unpacking operations on objects in Python. This method unpacks a string and stores its characters in a list, as shown below.
word = "Sample"
print([*word])
Output:
['S', 'a', 'm', 'p', 'l', 'e']
Use the List Comprehension to Split a String Into a Char Array in Python
List comprehension is an elegant way of creating lists in a single line of code. In the method shown below, we use the for
loop to iterate over the list and store each element.
word = "Sample"
lst = [x for x in word]
print(lst)
Output:
['S', 'a', 'm', 'p', 'l', 'e']
Use the map()
Function to Split a String Into a Char Array in Python
The map()
function can be used to apply a function to all elements of an input list.
word = "Sample"
char_array = list(map(str, word))
print(char_array)
In this method, we store the string in the variable word
and use map()
to apply the str
function (which converts elements to strings) to each character in word
. The resulting list is printed as the character array.
Output:
['S', 'a', 'm', 'p', 'l', 'e']
Use the itertools.chain
Function to Split a String Into a Char Array in Python
Python’s itertools
module provides powerful tools for working with iterators. One of its functions, itertools.chain
, can be used to split a string into a character array.
from itertools import chain
# Define the input string
input_string = "Hello!"
# Use itertools.chain to split the string into a character array
char_array = list(chain(input_string))
# Print the character array
print(char_array)
We first import the chain
function from the itertools
module and define an input_string
containing the text we want to split into characters.
We use chain(input_string)
to create an iterator that iterates over each character in the string and convert the iterator to a list using list()
to obtain the character array. The resulting list is printed as the character array.
Output:
['H', 'e', 'l', 'l', 'o', '!']
In this example, we’ve used itertools.chain
to split the string into a character array efficiently. This can be useful when working with large strings or combining them with other iterators or sequences.
Conclusion
In conclusion, Python provides several methods to split a string into a character array, each with advantages. Understanding these techniques empowers you to manipulate and process strings in your Python programs efficiently.
Whether you prefer a loop-based approach or more concise methods like list comprehension, Python offers versatility in handling strings and characters.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn