How to Sort Counter Based on Values in Python
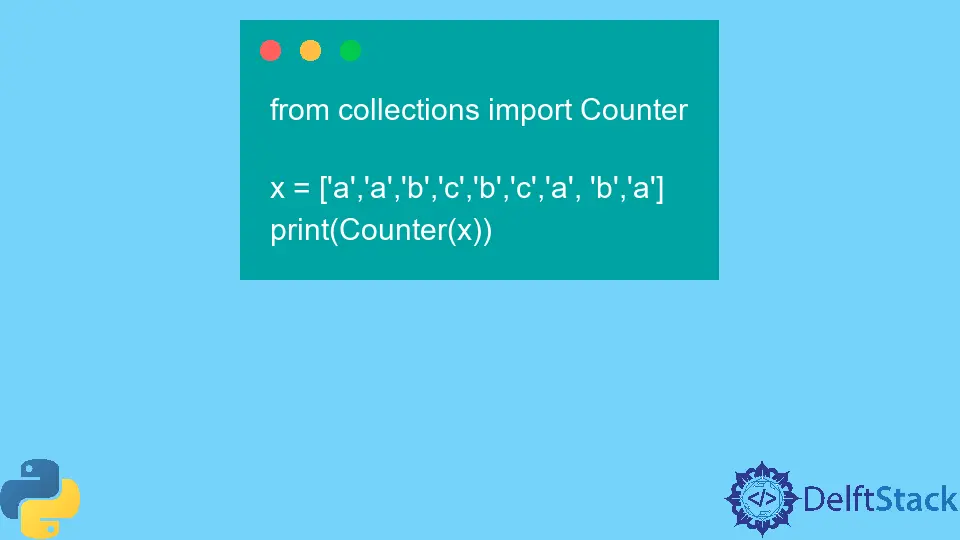
This tutorial demonstrates how to sort the Counter
based on its values using Python.
Overview of a Counter
in Python
A Counter
is a part of the collections
module in Python that helps calculate the total number of occurrences of a particular character. This occurrence could be a part of an array or a string.
Let us understand how to use a collection to count the number of occurrences of a particular character in Python. We can do it with the help of the following code.
from collections import Counter
x = ["a", "a", "b", "c", "b", "c", "a", "b", "a"]
print(Counter(x))
Output:
Counter({'a': 4, 'b': 3, 'c': 2})
Sort Counter
Based on Values in Python
Now that we have learned how to use Counter
in Python using the collections
module, let us try to understand how to sort the values of a Counter
.
We can do it with the help of the most_common()
function of a Counter
. The most_common()
function helps us find the character that has occurred most times in a given data structure.
One can make use of the most_common()
function with the help of the following Python code.
from collections import Counter
x = Counter(["a", "a", "b", "c", "b", "c", "a", "b", "a"])
print(x.most_common())
Output:
[('a', 4), ('b', 3), ('c', 2)]
Note that the output of the most_common()
function of a Counter
is an array of values sorted in descending order.
Similarly, we have the least_common
function within a Counter
. This function takes the count and finds the characters that occur the least number of times. See the following code:
from collections import Counter
x = Counter(["a", "a", "b", "c", "b", "c", "a", "b", "a"])
print(x.most_common()[::-1])
Output:
[('c', 2), ('b', 3), ('a', 4)]
Thus, we have successfully explored how to sort the characters of a data structure using Counter
from the collections
module based on their values in Python.