How to Implement the Snmpwalk Utility in Python
- Understanding SNMP and snmpwalk
- Setting Up Your Environment
- Implementing snmpwalk with pysnmp
- Handling Multiple OIDs
- Conclusion
- FAQ
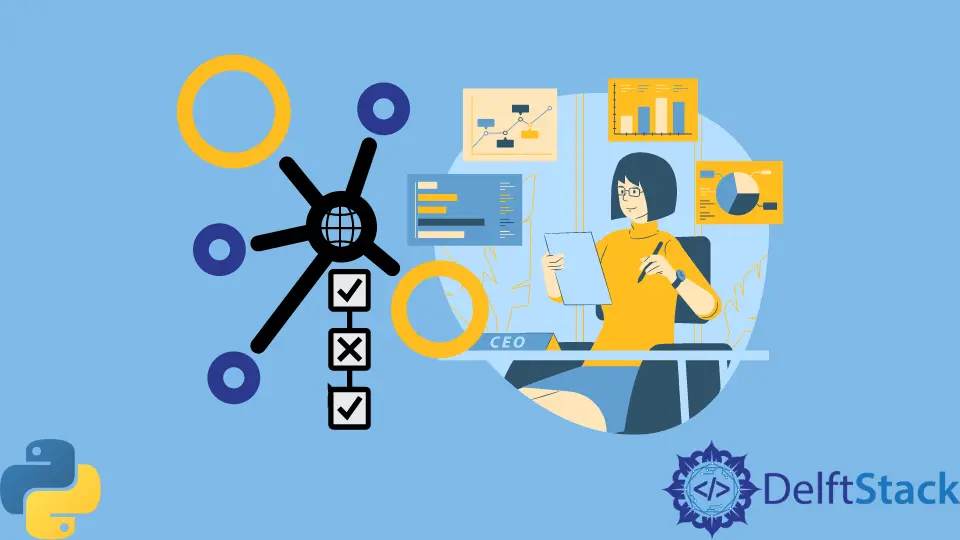
In the world of network management, SNMP (Simple Network Management Protocol) is a critical tool for monitoring and managing devices. One of the most useful utilities associated with SNMP is snmpwalk, which allows users to retrieve a subtree of management values from a network device.
In this tutorial, we will explore how to implement the snmpwalk utility in Python. By leveraging Python’s capabilities, we can automate network monitoring tasks, making it easier to gather data from devices such as routers, switches, and servers. Whether you’re a seasoned developer or just starting with Python, this guide will help you understand how to effectively use snmpwalk in your projects.
Understanding SNMP and snmpwalk
Before diving into the implementation, let’s briefly discuss what SNMP and snmpwalk are. SNMP is a protocol used for network management, allowing devices to exchange information about their status and performance. The snmpwalk command is a simple tool that queries SNMP-enabled devices for information, returning a list of OIDs (Object Identifiers) and their corresponding values.
In Python, we can utilize libraries such as pysnmp
to execute snmpwalk commands programmatically. This provides a flexible way to collect data from network devices without needing to run command-line tools manually.
Setting Up Your Environment
To get started, you need to install the pysnmp
library if you haven’t already. You can easily install it using pip. Open your terminal or command prompt and run:
pip install pysnmp
This will download and install the pysnmp
library, allowing you to interact with SNMP devices directly from your Python scripts.
Implementing snmpwalk with pysnmp
Now that you have set up your environment, let’s implement the snmpwalk utility using the pysnmp
library. Below is a Python script that demonstrates how to use the library to perform an SNMP walk on a device.
from pysnmp.hlapi import *
def snmp_walk(target, community, oid):
iterator = getCmd(SnmpEngine(),
CommunityData(community),
UdpTransportTarget((target, 161)),
ContextData(),
ObjectType(ObjectIdentity(oid)))
errorIndication, errorStatus, errorIndex, varBinds = next(iterator)
if errorIndication:
print(errorIndication)
elif errorStatus:
print('%s at %s' % (errorStatus.prettyPrint(),
errorIndex and varBinds[int(errorIndex) - 1] or '?'))
else:
for varBind in varBinds:
print(varBind)
snmp_walk('192.168.1.1', 'public', '1.3.6.1.2.1.1')
The script begins by importing the necessary components from the pysnmp
library. The snmp_walk
function takes three parameters: target
, community
, and oid
. The target
is the IP address of the device you want to query, community
is the SNMP community string (like a password), and oid
is the object identifier you want to retrieve.
The getCmd
function is used to send the SNMP request, and the response is processed to check for errors. If no errors occur, the script prints out the OID values retrieved from the device.
Output:
SNMPv2-MIB::sysDescr.0 = STRING: Linux 4.15.0-20-generic
This output indicates that the script successfully retrieved the system description from the device at the specified IP address.
Handling Multiple OIDs
In many cases, you may want to retrieve multiple OIDs in one go. The following example demonstrates how to perform an SNMP walk to get a range of OIDs.
def snmp_walk_multiple(target, community, oid):
iterator = nextCmd(SnmpEngine(),
CommunityData(community),
UdpTransportTarget((target, 161)),
ContextData(),
ObjectType(ObjectIdentity(oid)),
lexicographicMode=True)
for errorIndication, errorStatus, errorIndex, varBinds in iterator:
if errorIndication:
print(errorIndication)
break
elif errorStatus:
print('%s at %s' % (errorStatus.prettyPrint(),
errorIndex and varBinds[int(errorIndex) - 1] or '?'))
break
else:
for varBind in varBinds:
print(varBind)
snmp_walk_multiple('192.168.1.1', 'public', '1.3.6.1.2.1.1')
In this function, nextCmd
is used instead of getCmd
. This allows you to retrieve multiple OIDs in a single call, making it efficient for gathering data from devices. The lexicographicMode=True
parameter ensures that the OIDs are retrieved in a sorted order.
Output:
SNMPv2-MIB::sysDescr.0 = STRING: Linux 4.15.0-20-generic
SNMPv2-MIB::sysUpTime.0 = Timeticks: (12345678) 1 day, 10:17:36.78
Here, the output shows both the system description and the uptime of the device. This is a powerful way to gather comprehensive information with minimal code.
Conclusion
Implementing the snmpwalk utility in Python using the pysnmp
library is a straightforward process that can significantly enhance your network management capabilities. By automating the retrieval of SNMP data, you can streamline your monitoring tasks and gain valuable insights into your network devices. Whether you’re working on a small home network or a large enterprise setup, understanding how to use snmpwalk in Python can empower you to manage your devices more effectively. With the examples provided, you now have a solid foundation to start building your own network monitoring solutions.
FAQ
-
what is snmpwalk?
snmpwalk is a command-line utility that retrieves a subtree of management values from a network device using SNMP. -
how do I install pysnmp?
you can install pysnmp using pip with the command: pip install pysnmp. -
can I use snmpwalk for multiple OIDs?
yes, you can use the nextCmd function in pysnmp to retrieve multiple OIDs in one request. -
what is an OID?
an OID (Object Identifier) is a unique identifier used to represent a specific piece of information in SNMP. -
how can I handle errors in pysnmp?
you can check the errorIndication and errorStatus variables after sending an SNMP request to handle errors.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn