How to Implement Sliding Window in Python
- What is the Sliding Window Technique?
- Implementing Sliding Window for Maximum Sum of Subarray of Size K
- Sliding Window for Longest Substring Without Repeating Characters
- Sliding Window for Minimum Size Subarray Sum
- Conclusion
- FAQ
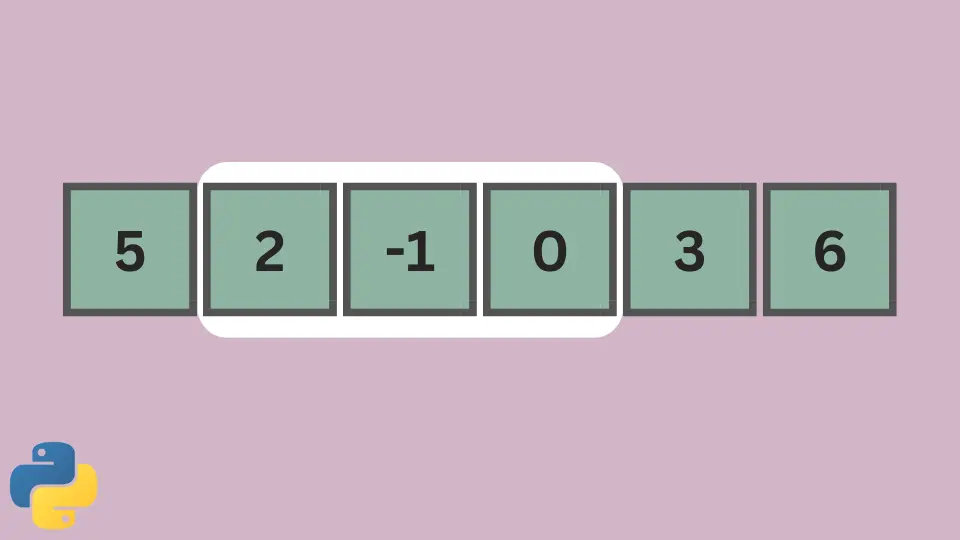
When it comes to solving problems in programming, the sliding window technique is a powerful and efficient approach. It’s particularly useful for dealing with arrays or lists where you need to examine a subset of elements.
In this tutorial, we will delve into what the sliding window technique is and explore how to implement it in Python. You’ll learn about its applications, advantages, and see practical code examples that will help you grasp this technique effectively. Whether you’re preparing for coding interviews or enhancing your programming skills, mastering the sliding window technique will undoubtedly elevate your problem-solving abilities.
What is the Sliding Window Technique?
The sliding window technique is a method used to solve problems that involve linear data structures, such as arrays or lists. The idea is simple: instead of examining every possible subset of elements, you maintain a window of elements that slides over the data structure. This allows for more efficient computations and reduces the time complexity of your algorithms.
For example, if you need to find the maximum sum of any contiguous subarray of size k
, rather than recalculating the sum for every possible subarray, you can compute it for the first k
elements and then slide the window one element at a time, adjusting the sum accordingly. This can significantly reduce the number of operations needed.
Implementing Sliding Window for Maximum Sum of Subarray of Size K
Let’s jump into a practical example of the sliding window technique in Python. We’ll implement a function that finds the maximum sum of contiguous subarrays of size k
in a given array.
def max_sum_subarray(arr, k):
n = len(arr)
if n < k:
return "Invalid"
max_sum = sum(arr[:k])
window_sum = max_sum
for i in range(n - k):
window_sum = window_sum - arr[i] + arr[i + k]
max_sum = max(max_sum, window_sum)
return max_sum
# Example usage
arr = [2, 1, 5, 1, 3, 2]
k = 3
result = max_sum_subarray(arr, k)
Output:
9
The function max_sum_subarray
takes an array arr
and an integer k
as inputs. It first checks if the length of the array is less than k
, returning “Invalid” if true. It then calculates the sum of the first k
elements and initializes max_sum
and window_sum
with this value. As the loop iterates through the array, it updates the window_sum
by subtracting the element that is leaving the window and adding the new element that is entering. The maximum sum is updated accordingly.
Sliding Window for Longest Substring Without Repeating Characters
Another common problem that can be efficiently solved using the sliding window technique is finding the longest substring without repeating characters. This is a classic problem that often comes up in coding interviews.
def longest_substring(s):
char_set = set()
left = max_length = 0
for right in range(len(s)):
while s[right] in char_set:
char_set.remove(s[left])
left += 1
char_set.add(s[right])
max_length = max(max_length, right - left + 1)
return max_length
# Example usage
s = "abcabcbb"
result = longest_substring(s)
Output:
3
In the longest_substring
function, we use a set to track the characters in the current window. The left
pointer represents the start of the window, while the right
pointer iterates over the string. When a duplicate character is found, we shrink the window from the left until the duplicate is removed. The maximum length of the substring is updated during each iteration. This efficient approach ensures that we only traverse the string once, resulting in a time complexity of O(n).
Sliding Window for Minimum Size Subarray Sum
The sliding window technique can also be applied to find the minimum size subarray with a sum greater than or equal to a target value. This problem can be a bit tricky, but the sliding window approach simplifies it significantly.
def min_subarray_len(target, nums):
left = total = 0
min_length = float('inf')
for right in range(len(nums)):
total += nums[right]
while total >= target:
min_length = min(min_length, right - left + 1)
total -= nums[left]
left += 1
return min_length if min_length != float('inf') else 0
# Example usage
target = 7
nums = [2, 3, 1, 2, 4, 3]
result = min_subarray_len(target, nums)
Output:
2
The min_subarray_len
function takes a target sum and an array of numbers as input. It maintains a running total of the current window. As soon as the total meets or exceeds the target, it checks if the current window size is the smallest found so far. If so, it updates min_length
. The left pointer is then moved to shrink the window while still meeting the target condition. This approach ensures that we efficiently find the minimum length subarray.
Conclusion
The sliding window technique is a versatile and efficient approach to solving various problems involving arrays and lists in Python. By maintaining a dynamic window that adjusts as you iterate through the data, you can significantly reduce the time complexity of your algorithms. Whether you’re tackling problems related to sums, substrings, or other linear data structures, mastering this technique will enhance your programming toolkit. As you practice and apply the sliding window technique, you’ll find it becomes an invaluable asset in your coding journey.
FAQ
-
What is the sliding window technique?
The sliding window technique is a method used to solve problems involving linear data structures by maintaining a dynamic subset of elements that slides over the data. -
In which scenarios is the sliding window technique useful?
It is particularly useful for problems involving contiguous subarrays, substrings, or any scenario where you need to analyze a subset of elements in a linear data structure.
-
How does the sliding window improve efficiency?
By avoiding the need to recalculate values for every possible subset, the sliding window technique reduces the number of operations, often resulting in a time complexity of O(n). -
Can the sliding window technique be used with other data structures?
While it is most commonly used with arrays and lists, the sliding window technique can also be adapted for use with other data structures, such as strings. -
What are some common problems solved using the sliding window technique?
Common problems include finding the maximum sum of subarrays, the longest substring without repeating characters, and the minimum size subarray sum.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn